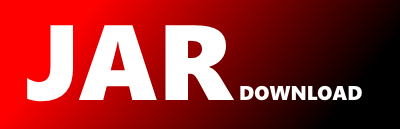
com.pulumi.azurenative.servicelinker.kotlin.ConnectorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.servicelinker.kotlin
import com.pulumi.azurenative.servicelinker.ConnectorArgs.builder
import com.pulumi.azurenative.servicelinker.kotlin.enums.ClientType
import com.pulumi.azurenative.servicelinker.kotlin.inputs.ConfigurationInfoArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.ConfigurationInfoArgsBuilder
import com.pulumi.azurenative.servicelinker.kotlin.inputs.PublicNetworkSolutionArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.PublicNetworkSolutionArgsBuilder
import com.pulumi.azurenative.servicelinker.kotlin.inputs.SecretStoreArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.SecretStoreArgsBuilder
import com.pulumi.azurenative.servicelinker.kotlin.inputs.VNetSolutionArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.VNetSolutionArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Linker of source and target resource
* Azure REST API version: 2022-11-01-preview.
* Other available API versions: 2023-04-01-preview, 2024-04-01.
* ## Example Usage
* ### PutConnector
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var connector = new AzureNative.ServiceLinker.Connector("connector", new()
* {
* AuthInfo = new AzureNative.ServiceLinker.Inputs.SecretAuthInfoArgs
* {
* AuthType = "secret",
* },
* ConnectorName = "connectorName",
* Location = "westus",
* ResourceGroupName = "test-rg",
* SecretStore = new AzureNative.ServiceLinker.Inputs.SecretStoreArgs
* {
* KeyVaultId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.KeyVault/vaults/test-kv",
* },
* TargetService = new AzureNative.ServiceLinker.Inputs.AzureResourceArgs
* {
* Id = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.DocumentDb/databaseAccounts/test-acc/mongodbDatabases/test-db",
* Type = "AzureResource",
* },
* });
* });
* ```
* ```go
* package main
* import (
* servicelinker "github.com/pulumi/pulumi-azure-native-sdk/servicelinker/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := servicelinker.NewConnector(ctx, "connector", &servicelinker.ConnectorArgs{
* AuthInfo: &servicelinker.SecretAuthInfoArgs{
* AuthType: pulumi.String("secret"),
* },
* ConnectorName: pulumi.String("connectorName"),
* Location: pulumi.String("westus"),
* ResourceGroupName: pulumi.String("test-rg"),
* SecretStore: &servicelinker.SecretStoreArgs{
* KeyVaultId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.KeyVault/vaults/test-kv"),
* },
* TargetService: &servicelinker.AzureResourceArgs{
* Id: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.DocumentDb/databaseAccounts/test-acc/mongodbDatabases/test-db"),
* Type: pulumi.String("AzureResource"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.servicelinker.Connector;
* import com.pulumi.azurenative.servicelinker.ConnectorArgs;
* import com.pulumi.azurenative.servicelinker.inputs.SecretStoreArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var connector = new Connector("connector", ConnectorArgs.builder()
* .authInfo(AccessKeyInfoBaseArgs.builder()
* .authType("secret")
* .build())
* .connectorName("connectorName")
* .location("westus")
* .resourceGroupName("test-rg")
* .secretStore(SecretStoreArgs.builder()
* .keyVaultId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.KeyVault/vaults/test-kv")
* .build())
* .targetService(AzureResourceArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.DocumentDb/databaseAccounts/test-acc/mongodbDatabases/test-db")
* .type("AzureResource")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:servicelinker:Connector linkName /subscriptions/{subscriptionId}/resourcegroups/{resourceGroupName}/providers/Microsoft.ServiceLinker/locations/{location}/connectors/{connectorName}
* ```
* @property authInfo The authentication type.
* @property clientType The application client type
* @property configurationInfo The connection information consumed by applications, including secrets, connection strings.
* @property connectorName The name of resource.
* @property location The name of Azure region.
* @property publicNetworkSolution The network solution.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property scope connection scope in source service.
* @property secretStore An option to store secret value in secure place
* @property subscriptionId The ID of the target subscription.
* @property targetService The target service properties
* @property vNetSolution The VNet solution.
*/
public data class ConnectorArgs(
public val authInfo: Output? = null,
public val clientType: Output>? = null,
public val configurationInfo: Output? = null,
public val connectorName: Output? = null,
public val location: Output? = null,
public val publicNetworkSolution: Output? = null,
public val resourceGroupName: Output? = null,
public val scope: Output? = null,
public val secretStore: Output? = null,
public val subscriptionId: Output? = null,
public val targetService: Output? = null,
public val vNetSolution: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.servicelinker.ConnectorArgs =
com.pulumi.azurenative.servicelinker.ConnectorArgs.builder()
.authInfo(authInfo?.applyValue({ args0 -> args0 }))
.clientType(
clientType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.configurationInfo(configurationInfo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.connectorName(connectorName?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.publicNetworkSolution(
publicNetworkSolution?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.scope(scope?.applyValue({ args0 -> args0 }))
.secretStore(secretStore?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.subscriptionId(subscriptionId?.applyValue({ args0 -> args0 }))
.targetService(targetService?.applyValue({ args0 -> args0 }))
.vNetSolution(vNetSolution?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ConnectorArgs].
*/
@PulumiTagMarker
public class ConnectorArgsBuilder internal constructor() {
private var authInfo: Output? = null
private var clientType: Output>? = null
private var configurationInfo: Output? = null
private var connectorName: Output? = null
private var location: Output? = null
private var publicNetworkSolution: Output? = null
private var resourceGroupName: Output? = null
private var scope: Output? = null
private var secretStore: Output? = null
private var subscriptionId: Output? = null
private var targetService: Output? = null
private var vNetSolution: Output? = null
/**
* @param value The authentication type.
*/
@JvmName("oltyqjccnkwpevkx")
public suspend fun authInfo(`value`: Output) {
this.authInfo = value
}
/**
* @param value The application client type
*/
@JvmName("ouuixslelbmmtbky")
public suspend fun clientType(`value`: Output>) {
this.clientType = value
}
/**
* @param value The connection information consumed by applications, including secrets, connection strings.
*/
@JvmName("lhahbjfkurebyljp")
public suspend fun configurationInfo(`value`: Output) {
this.configurationInfo = value
}
/**
* @param value The name of resource.
*/
@JvmName("wwcxmrtxgyvejldb")
public suspend fun connectorName(`value`: Output) {
this.connectorName = value
}
/**
* @param value The name of Azure region.
*/
@JvmName("aktmuqktustiywfu")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The network solution.
*/
@JvmName("bgubckxhiklvcowy")
public suspend fun publicNetworkSolution(`value`: Output) {
this.publicNetworkSolution = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("rebnquqiaoxyhepq")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value connection scope in source service.
*/
@JvmName("jkxaikkwlkbhwnoo")
public suspend fun scope(`value`: Output) {
this.scope = value
}
/**
* @param value An option to store secret value in secure place
*/
@JvmName("lhpsbrxayqpdlqpc")
public suspend fun secretStore(`value`: Output) {
this.secretStore = value
}
/**
* @param value The ID of the target subscription.
*/
@JvmName("tsvdwkrpxthndhcx")
public suspend fun subscriptionId(`value`: Output) {
this.subscriptionId = value
}
/**
* @param value The target service properties
*/
@JvmName("bjhajxsavrfvuocf")
public suspend fun targetService(`value`: Output) {
this.targetService = value
}
/**
* @param value The VNet solution.
*/
@JvmName("phmyficqhjnacxyv")
public suspend fun vNetSolution(`value`: Output) {
this.vNetSolution = value
}
/**
* @param value The authentication type.
*/
@JvmName("yffvgpeyotdexkke")
public suspend fun authInfo(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authInfo = mapped
}
/**
* @param value The application client type
*/
@JvmName("rokghrrdjnswymul")
public suspend fun clientType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientType = mapped
}
/**
* @param value The application client type
*/
@JvmName("hfikjlnxkwqbjaoi")
public fun clientType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientType = mapped
}
/**
* @param value The application client type
*/
@JvmName("lqlobuoybupyrghu")
public fun clientType(`value`: ClientType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientType = mapped
}
/**
* @param value The connection information consumed by applications, including secrets, connection strings.
*/
@JvmName("hcobjavcpcxwmvcw")
public suspend fun configurationInfo(`value`: ConfigurationInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configurationInfo = mapped
}
/**
* @param argument The connection information consumed by applications, including secrets, connection strings.
*/
@JvmName("tagumjmiuuygdrqe")
public suspend fun configurationInfo(argument: suspend ConfigurationInfoArgsBuilder.() -> Unit) {
val toBeMapped = ConfigurationInfoArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.configurationInfo = mapped
}
/**
* @param value The name of resource.
*/
@JvmName("fxqkogsmxlcmqwub")
public suspend fun connectorName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorName = mapped
}
/**
* @param value The name of Azure region.
*/
@JvmName("jktkybuivpjcqksn")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The network solution.
*/
@JvmName("krsdnohxbleytjqr")
public suspend fun publicNetworkSolution(`value`: PublicNetworkSolutionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicNetworkSolution = mapped
}
/**
* @param argument The network solution.
*/
@JvmName("dgsnwrwwthsgdcqv")
public suspend fun publicNetworkSolution(argument: suspend PublicNetworkSolutionArgsBuilder.() -> Unit) {
val toBeMapped = PublicNetworkSolutionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.publicNetworkSolution = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("oorysxsocrqnoelc")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value connection scope in source service.
*/
@JvmName("pdospcfhxmepblmj")
public suspend fun scope(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scope = mapped
}
/**
* @param value An option to store secret value in secure place
*/
@JvmName("tvjqynwupsbrjetw")
public suspend fun secretStore(`value`: SecretStoreArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretStore = mapped
}
/**
* @param argument An option to store secret value in secure place
*/
@JvmName("nulnilfawclxckfv")
public suspend fun secretStore(argument: suspend SecretStoreArgsBuilder.() -> Unit) {
val toBeMapped = SecretStoreArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secretStore = mapped
}
/**
* @param value The ID of the target subscription.
*/
@JvmName("vhupxipyetbobjmw")
public suspend fun subscriptionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subscriptionId = mapped
}
/**
* @param value The target service properties
*/
@JvmName("uvpygwsjttpecdjl")
public suspend fun targetService(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetService = mapped
}
/**
* @param value The VNet solution.
*/
@JvmName("hvocuiglcbuyjspp")
public suspend fun vNetSolution(`value`: VNetSolutionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vNetSolution = mapped
}
/**
* @param argument The VNet solution.
*/
@JvmName("udusyfokdyqkutdt")
public suspend fun vNetSolution(argument: suspend VNetSolutionArgsBuilder.() -> Unit) {
val toBeMapped = VNetSolutionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.vNetSolution = mapped
}
internal fun build(): ConnectorArgs = ConnectorArgs(
authInfo = authInfo,
clientType = clientType,
configurationInfo = configurationInfo,
connectorName = connectorName,
location = location,
publicNetworkSolution = publicNetworkSolution,
resourceGroupName = resourceGroupName,
scope = scope,
secretStore = secretStore,
subscriptionId = subscriptionId,
targetService = targetService,
vNetSolution = vNetSolution,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy