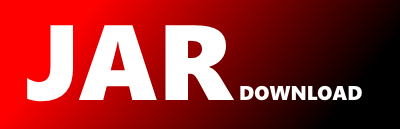
com.pulumi.azurenative.servicelinker.kotlin.LinkerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.servicelinker.kotlin
import com.pulumi.azurenative.servicelinker.LinkerArgs.builder
import com.pulumi.azurenative.servicelinker.kotlin.enums.ClientType
import com.pulumi.azurenative.servicelinker.kotlin.inputs.ConfigurationInfoArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.ConfigurationInfoArgsBuilder
import com.pulumi.azurenative.servicelinker.kotlin.inputs.PublicNetworkSolutionArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.PublicNetworkSolutionArgsBuilder
import com.pulumi.azurenative.servicelinker.kotlin.inputs.SecretStoreArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.SecretStoreArgsBuilder
import com.pulumi.azurenative.servicelinker.kotlin.inputs.VNetSolutionArgs
import com.pulumi.azurenative.servicelinker.kotlin.inputs.VNetSolutionArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Linker of source and target resource
* Azure REST API version: 2022-11-01-preview. Prior API version in Azure Native 1.x: 2021-11-01-preview.
* Other available API versions: 2021-11-01-preview, 2023-04-01-preview, 2024-04-01.
* ## Example Usage
* ### PutLinker
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var linker = new AzureNative.ServiceLinker.Linker("linker", new()
* {
* AuthInfo = new AzureNative.ServiceLinker.Inputs.SecretAuthInfoArgs
* {
* AuthType = "secret",
* Name = "name",
* SecretInfo = new AzureNative.ServiceLinker.Inputs.ValueSecretInfoArgs
* {
* SecretType = "rawValue",
* Value = "secret",
* },
* },
* LinkerName = "linkName",
* ResourceUri = "subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.Web/sites/test-app",
* TargetService = new AzureNative.ServiceLinker.Inputs.AzureResourceArgs
* {
* Id = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.DBforPostgreSQL/servers/test-pg/databases/test-db",
* Type = "AzureResource",
* },
* VNetSolution = new AzureNative.ServiceLinker.Inputs.VNetSolutionArgs
* {
* Type = AzureNative.ServiceLinker.VNetSolutionType.ServiceEndpoint,
* },
* });
* });
* ```
* ```go
* package main
* import (
* servicelinker "github.com/pulumi/pulumi-azure-native-sdk/servicelinker/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := servicelinker.NewLinker(ctx, "linker", &servicelinker.LinkerArgs{
* AuthInfo: &servicelinker.SecretAuthInfoArgs{
* AuthType: pulumi.String("secret"),
* Name: pulumi.String("name"),
* SecretInfo: servicelinker.ValueSecretInfo{
* SecretType: "rawValue",
* Value: "secret",
* },
* },
* LinkerName: pulumi.String("linkName"),
* ResourceUri: pulumi.String("subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.Web/sites/test-app"),
* TargetService: &servicelinker.AzureResourceArgs{
* Id: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.DBforPostgreSQL/servers/test-pg/databases/test-db"),
* Type: pulumi.String("AzureResource"),
* },
* VNetSolution: &servicelinker.VNetSolutionArgs{
* Type: pulumi.String(servicelinker.VNetSolutionTypeServiceEndpoint),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.servicelinker.Linker;
* import com.pulumi.azurenative.servicelinker.LinkerArgs;
* import com.pulumi.azurenative.servicelinker.inputs.VNetSolutionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var linker = new Linker("linker", LinkerArgs.builder()
* .authInfo(AccessKeyInfoBaseArgs.builder()
* .authType("secret")
* .name("name")
* .secretInfo(%!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference))
* .build())
* .linkerName("linkName")
* .resourceUri("subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.Web/sites/test-app")
* .targetService(AzureResourceArgs.builder()
* .id("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/test-rg/providers/Microsoft.DBforPostgreSQL/servers/test-pg/databases/test-db")
* .type("AzureResource")
* .build())
* .vNetSolution(VNetSolutionArgs.builder()
* .type("serviceEndpoint")
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:servicelinker:Linker linkName /{resourceUri}/providers/Microsoft.ServiceLinker/linkers/{linkerName}
* ```
* @property authInfo The authentication type.
* @property clientType The application client type
* @property configurationInfo The connection information consumed by applications, including secrets, connection strings.
* @property linkerName The name Linker resource.
* @property publicNetworkSolution The network solution.
* @property resourceUri The fully qualified Azure Resource manager identifier of the resource to be connected.
* @property scope connection scope in source service.
* @property secretStore An option to store secret value in secure place
* @property targetService The target service properties
* @property vNetSolution The VNet solution.
*/
public data class LinkerArgs(
public val authInfo: Output? = null,
public val clientType: Output>? = null,
public val configurationInfo: Output? = null,
public val linkerName: Output? = null,
public val publicNetworkSolution: Output? = null,
public val resourceUri: Output? = null,
public val scope: Output? = null,
public val secretStore: Output? = null,
public val targetService: Output? = null,
public val vNetSolution: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.servicelinker.LinkerArgs =
com.pulumi.azurenative.servicelinker.LinkerArgs.builder()
.authInfo(authInfo?.applyValue({ args0 -> args0 }))
.clientType(
clientType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.configurationInfo(configurationInfo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.linkerName(linkerName?.applyValue({ args0 -> args0 }))
.publicNetworkSolution(
publicNetworkSolution?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.resourceUri(resourceUri?.applyValue({ args0 -> args0 }))
.scope(scope?.applyValue({ args0 -> args0 }))
.secretStore(secretStore?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.targetService(targetService?.applyValue({ args0 -> args0 }))
.vNetSolution(vNetSolution?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [LinkerArgs].
*/
@PulumiTagMarker
public class LinkerArgsBuilder internal constructor() {
private var authInfo: Output? = null
private var clientType: Output>? = null
private var configurationInfo: Output? = null
private var linkerName: Output? = null
private var publicNetworkSolution: Output? = null
private var resourceUri: Output? = null
private var scope: Output? = null
private var secretStore: Output? = null
private var targetService: Output? = null
private var vNetSolution: Output? = null
/**
* @param value The authentication type.
*/
@JvmName("ribgesdntcwsnkei")
public suspend fun authInfo(`value`: Output) {
this.authInfo = value
}
/**
* @param value The application client type
*/
@JvmName("xknajuvyewayxwwg")
public suspend fun clientType(`value`: Output>) {
this.clientType = value
}
/**
* @param value The connection information consumed by applications, including secrets, connection strings.
*/
@JvmName("weigbfmwvgqcalga")
public suspend fun configurationInfo(`value`: Output) {
this.configurationInfo = value
}
/**
* @param value The name Linker resource.
*/
@JvmName("sclponrwqfgwdvhw")
public suspend fun linkerName(`value`: Output) {
this.linkerName = value
}
/**
* @param value The network solution.
*/
@JvmName("ncvgiaqejorhxegv")
public suspend fun publicNetworkSolution(`value`: Output) {
this.publicNetworkSolution = value
}
/**
* @param value The fully qualified Azure Resource manager identifier of the resource to be connected.
*/
@JvmName("rioignwnevjjbvqb")
public suspend fun resourceUri(`value`: Output) {
this.resourceUri = value
}
/**
* @param value connection scope in source service.
*/
@JvmName("ftacatpxmdpobbaq")
public suspend fun scope(`value`: Output) {
this.scope = value
}
/**
* @param value An option to store secret value in secure place
*/
@JvmName("dhukclefsonepgei")
public suspend fun secretStore(`value`: Output) {
this.secretStore = value
}
/**
* @param value The target service properties
*/
@JvmName("vwmgccrdbfbjjkbq")
public suspend fun targetService(`value`: Output) {
this.targetService = value
}
/**
* @param value The VNet solution.
*/
@JvmName("mwyeitlugcmttsxu")
public suspend fun vNetSolution(`value`: Output) {
this.vNetSolution = value
}
/**
* @param value The authentication type.
*/
@JvmName("oapyitlbjibasjsa")
public suspend fun authInfo(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authInfo = mapped
}
/**
* @param value The application client type
*/
@JvmName("fqtfbqjsjbvtrynu")
public suspend fun clientType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientType = mapped
}
/**
* @param value The application client type
*/
@JvmName("nvtshwhktvawapjp")
public fun clientType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientType = mapped
}
/**
* @param value The application client type
*/
@JvmName("vfwuwpdgkmorsegg")
public fun clientType(`value`: ClientType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clientType = mapped
}
/**
* @param value The connection information consumed by applications, including secrets, connection strings.
*/
@JvmName("jryjqyeataxagvei")
public suspend fun configurationInfo(`value`: ConfigurationInfoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configurationInfo = mapped
}
/**
* @param argument The connection information consumed by applications, including secrets, connection strings.
*/
@JvmName("wkjjijhmdwkwpvcr")
public suspend fun configurationInfo(argument: suspend ConfigurationInfoArgsBuilder.() -> Unit) {
val toBeMapped = ConfigurationInfoArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.configurationInfo = mapped
}
/**
* @param value The name Linker resource.
*/
@JvmName("sjnbopmpixmgccob")
public suspend fun linkerName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linkerName = mapped
}
/**
* @param value The network solution.
*/
@JvmName("sdwatnmskbcwqice")
public suspend fun publicNetworkSolution(`value`: PublicNetworkSolutionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicNetworkSolution = mapped
}
/**
* @param argument The network solution.
*/
@JvmName("nakednghpxurvabe")
public suspend fun publicNetworkSolution(argument: suspend PublicNetworkSolutionArgsBuilder.() -> Unit) {
val toBeMapped = PublicNetworkSolutionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.publicNetworkSolution = mapped
}
/**
* @param value The fully qualified Azure Resource manager identifier of the resource to be connected.
*/
@JvmName("hwmishbmgllusjig")
public suspend fun resourceUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceUri = mapped
}
/**
* @param value connection scope in source service.
*/
@JvmName("ouvlhmkvbltnqpao")
public suspend fun scope(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scope = mapped
}
/**
* @param value An option to store secret value in secure place
*/
@JvmName("bfskfooxqhebqoyi")
public suspend fun secretStore(`value`: SecretStoreArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretStore = mapped
}
/**
* @param argument An option to store secret value in secure place
*/
@JvmName("ndrocgtukojgsaxv")
public suspend fun secretStore(argument: suspend SecretStoreArgsBuilder.() -> Unit) {
val toBeMapped = SecretStoreArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secretStore = mapped
}
/**
* @param value The target service properties
*/
@JvmName("uaemvduwhwbymcqi")
public suspend fun targetService(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetService = mapped
}
/**
* @param value The VNet solution.
*/
@JvmName("mwwajcqunhqnpvia")
public suspend fun vNetSolution(`value`: VNetSolutionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vNetSolution = mapped
}
/**
* @param argument The VNet solution.
*/
@JvmName("seorposikpdktyvw")
public suspend fun vNetSolution(argument: suspend VNetSolutionArgsBuilder.() -> Unit) {
val toBeMapped = VNetSolutionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.vNetSolution = mapped
}
internal fun build(): LinkerArgs = LinkerArgs(
authInfo = authInfo,
clientType = clientType,
configurationInfo = configurationInfo,
linkerName = linkerName,
publicNetworkSolution = publicNetworkSolution,
resourceUri = resourceUri,
scope = scope,
secretStore = secretStore,
targetService = targetService,
vNetSolution = vNetSolution,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy