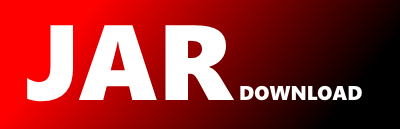
com.pulumi.azurenative.signalrservice.kotlin.outputs.GetSignalRResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.signalrservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A class represent a resource.
* @property cors Cross-Origin Resource Sharing (CORS) settings.
* @property disableAadAuth DisableLocalAuth
* Enable or disable aad auth
* When set as true, connection with AuthType=aad won't work.
* @property disableLocalAuth DisableLocalAuth
* Enable or disable local auth with AccessKey
* When set as true, connection with AccessKey=xxx won't work.
* @property externalIP The publicly accessible IP of the resource.
* @property features List of the featureFlags.
* FeatureFlags that are not included in the parameters for the update operation will not be modified.
* And the response will only include featureFlags that are explicitly set.
* When a featureFlag is not explicitly set, its globally default value will be used
* But keep in mind, the default value doesn't mean "false". It varies in terms of different FeatureFlags.
* @property hostName FQDN of the service instance.
* @property hostNamePrefix Deprecated.
* @property id Fully qualified resource Id for the resource.
* @property identity A class represent managed identities used for request and response
* @property kind The kind of the service, it can be SignalR or RawWebSockets
* @property liveTraceConfiguration Live trace configuration of a Microsoft.SignalRService resource.
* @property location The GEO location of the resource. e.g. West US | East US | North Central US | South Central US.
* @property name The name of the resource.
* @property networkACLs Network ACLs for the resource
* @property privateEndpointConnections Private endpoint connections to the resource.
* @property provisioningState Provisioning state of the resource.
* @property publicNetworkAccess Enable or disable public network access. Default to "Enabled".
* When it's Enabled, network ACLs still apply.
* When it's Disabled, public network access is always disabled no matter what you set in network ACLs.
* @property publicPort The publicly accessible port of the resource which is designed for browser/client side usage.
* @property resourceLogConfiguration Resource log configuration of a Microsoft.SignalRService resource.
* @property serverPort The publicly accessible port of the resource which is designed for customer server side usage.
* @property serverless Serverless settings.
* @property sharedPrivateLinkResources The list of shared private link resources.
* @property sku The billing information of the resource.
* @property systemData Metadata pertaining to creation and last modification of the resource.
* @property tags Tags of the service which is a list of key value pairs that describe the resource.
* @property tls TLS settings for the resource
* @property type The type of the resource - e.g. "Microsoft.SignalRService/SignalR"
* @property upstream The settings for the Upstream when the service is in server-less mode.
* @property version Version of the resource. Probably you need the same or higher version of client SDKs.
*/
public data class GetSignalRResult(
public val cors: SignalRCorsSettingsResponse? = null,
public val disableAadAuth: Boolean? = null,
public val disableLocalAuth: Boolean? = null,
public val externalIP: String,
public val features: List? = null,
public val hostName: String,
public val hostNamePrefix: String,
public val id: String,
public val identity: ManagedIdentityResponse? = null,
public val kind: String? = null,
public val liveTraceConfiguration: LiveTraceConfigurationResponse? = null,
public val location: String? = null,
public val name: String,
public val networkACLs: SignalRNetworkACLsResponse? = null,
public val privateEndpointConnections: List,
public val provisioningState: String,
public val publicNetworkAccess: String? = null,
public val publicPort: Int,
public val resourceLogConfiguration: ResourceLogConfigurationResponse? = null,
public val serverPort: Int,
public val serverless: ServerlessSettingsResponse? = null,
public val sharedPrivateLinkResources: List,
public val sku: ResourceSkuResponse? = null,
public val systemData: SystemDataResponse,
public val tags: Map? = null,
public val tls: SignalRTlsSettingsResponse? = null,
public val type: String,
public val upstream: ServerlessUpstreamSettingsResponse? = null,
public val version: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.signalrservice.outputs.GetSignalRResult): GetSignalRResult = GetSignalRResult(
cors = javaType.cors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.SignalRCorsSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
disableAadAuth = javaType.disableAadAuth().map({ args0 -> args0 }).orElse(null),
disableLocalAuth = javaType.disableLocalAuth().map({ args0 -> args0 }).orElse(null),
externalIP = javaType.externalIP(),
features = javaType.features().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.SignalRFeatureResponse.Companion.toKotlin(args0)
})
}),
hostName = javaType.hostName(),
hostNamePrefix = javaType.hostNamePrefix(),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.ManagedIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
kind = javaType.kind().map({ args0 -> args0 }).orElse(null),
liveTraceConfiguration = javaType.liveTraceConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.LiveTraceConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
location = javaType.location().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
networkACLs = javaType.networkACLs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.SignalRNetworkACLsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
privateEndpointConnections = javaType.privateEndpointConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.PrivateEndpointConnectionResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
publicNetworkAccess = javaType.publicNetworkAccess().map({ args0 -> args0 }).orElse(null),
publicPort = javaType.publicPort(),
resourceLogConfiguration = javaType.resourceLogConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.ResourceLogConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
serverPort = javaType.serverPort(),
serverless = javaType.serverless().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.ServerlessSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
sharedPrivateLinkResources = javaType.sharedPrivateLinkResources().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.SharedPrivateLinkResourceResponse.Companion.toKotlin(args0)
})
}),
sku = javaType.sku().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.ResourceSkuResponse.Companion.toKotlin(args0)
})
}).orElse(null),
systemData = javaType.systemData().let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.SystemDataResponse.Companion.toKotlin(args0)
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
tls = javaType.tls().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.SignalRTlsSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
type = javaType.type(),
upstream = javaType.upstream().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.signalrservice.kotlin.outputs.ServerlessUpstreamSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
version = javaType.version(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy