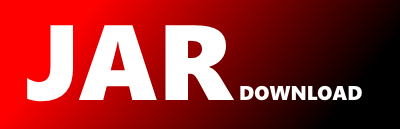
com.pulumi.azurenative.solutions.kotlin.outputs.GetApplicationResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.solutions.kotlin.outputs
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Information about managed application.
* @property applicationDefinitionId The fully qualified path of managed application definition Id.
* @property artifacts The collection of managed application artifacts.
* @property authorizations The read-only authorizations property that is retrieved from the application package.
* @property billingDetails The managed application billing details.
* @property createdBy The client entity that created the JIT request.
* @property customerSupport The read-only customer support property that is retrieved from the application package.
* @property id Resource ID
* @property identity The identity of the resource.
* @property jitAccessPolicy The managed application Jit access policy.
* @property kind The kind of the managed application. Allowed values are MarketPlace and ServiceCatalog.
* @property location Resource location
* @property managedBy ID of the resource that manages this resource.
* @property managedResourceGroupId The managed resource group Id.
* @property managementMode The managed application management mode.
* @property name Resource name
* @property outputs Name and value pairs that define the managed application outputs.
* @property parameters Name and value pairs that define the managed application parameters. It can be a JObject or a well formed JSON string.
* @property plan The plan information.
* @property provisioningState The managed application provisioning state.
* @property publisherTenantId The publisher tenant Id.
* @property sku The SKU of the resource.
* @property supportUrls The read-only support URLs property that is retrieved from the application package.
* @property systemData Metadata pertaining to creation and last modification of the resource.
* @property tags Resource tags
* @property type Resource type
* @property updatedBy The client entity that last updated the JIT request.
*/
public data class GetApplicationResult(
public val applicationDefinitionId: String? = null,
public val artifacts: List,
public val authorizations: List,
public val billingDetails: ApplicationBillingDetailsDefinitionResponse,
public val createdBy: ApplicationClientDetailsResponse,
public val customerSupport: ApplicationPackageContactResponse,
public val id: String,
public val identity: IdentityResponse? = null,
public val jitAccessPolicy: ApplicationJitAccessPolicyResponse? = null,
public val kind: String,
public val location: String? = null,
public val managedBy: String? = null,
public val managedResourceGroupId: String? = null,
public val managementMode: String,
public val name: String,
public val outputs: Any,
public val parameters: Any? = null,
public val plan: PlanResponse? = null,
public val provisioningState: String,
public val publisherTenantId: String,
public val sku: SkuResponse? = null,
public val supportUrls: ApplicationPackageSupportUrlsResponse,
public val systemData: SystemDataResponse,
public val tags: Map? = null,
public val type: String,
public val updatedBy: ApplicationClientDetailsResponse,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.solutions.outputs.GetApplicationResult): GetApplicationResult = GetApplicationResult(
applicationDefinitionId = javaType.applicationDefinitionId().map({ args0 -> args0 }).orElse(null),
artifacts = javaType.artifacts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationArtifactResponse.Companion.toKotlin(args0)
})
}),
authorizations = javaType.authorizations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationAuthorizationResponse.Companion.toKotlin(args0)
})
}),
billingDetails = javaType.billingDetails().let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationBillingDetailsDefinitionResponse.Companion.toKotlin(args0)
}),
createdBy = javaType.createdBy().let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationClientDetailsResponse.Companion.toKotlin(args0)
}),
customerSupport = javaType.customerSupport().let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationPackageContactResponse.Companion.toKotlin(args0)
}),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.IdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
jitAccessPolicy = javaType.jitAccessPolicy().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationJitAccessPolicyResponse.Companion.toKotlin(args0)
})
}).orElse(null),
kind = javaType.kind(),
location = javaType.location().map({ args0 -> args0 }).orElse(null),
managedBy = javaType.managedBy().map({ args0 -> args0 }).orElse(null),
managedResourceGroupId = javaType.managedResourceGroupId().map({ args0 -> args0 }).orElse(null),
managementMode = javaType.managementMode(),
name = javaType.name(),
outputs = javaType.outputs(),
parameters = javaType.parameters().map({ args0 -> args0 }).orElse(null),
plan = javaType.plan().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.PlanResponse.Companion.toKotlin(args0)
})
}).orElse(null),
provisioningState = javaType.provisioningState(),
publisherTenantId = javaType.publisherTenantId(),
sku = javaType.sku().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.SkuResponse.Companion.toKotlin(args0)
})
}).orElse(null),
supportUrls = javaType.supportUrls().let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationPackageSupportUrlsResponse.Companion.toKotlin(args0)
}),
systemData = javaType.systemData().let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.SystemDataResponse.Companion.toKotlin(args0)
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
updatedBy = javaType.updatedBy().let({ args0 ->
com.pulumi.azurenative.solutions.kotlin.outputs.ApplicationClientDetailsResponse.Companion.toKotlin(args0)
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy