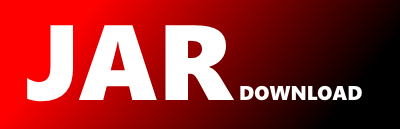
com.pulumi.azurenative.sql.kotlin.JobStepArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.sql.kotlin
import com.pulumi.azurenative.sql.JobStepArgs.builder
import com.pulumi.azurenative.sql.kotlin.inputs.JobStepActionArgs
import com.pulumi.azurenative.sql.kotlin.inputs.JobStepActionArgsBuilder
import com.pulumi.azurenative.sql.kotlin.inputs.JobStepExecutionOptionsArgs
import com.pulumi.azurenative.sql.kotlin.inputs.JobStepExecutionOptionsArgsBuilder
import com.pulumi.azurenative.sql.kotlin.inputs.JobStepOutputArgs
import com.pulumi.azurenative.sql.kotlin.inputs.JobStepOutputArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A job step.
* Azure REST API version: 2021-11-01. Prior API version in Azure Native 1.x: 2020-11-01-preview.
* Other available API versions: 2022-11-01-preview, 2023-02-01-preview, 2023-05-01-preview, 2023-08-01-preview.
* ## Example Usage
* ### Create or update a job step with all properties specified.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var jobStep = new AzureNative.Sql.JobStep("jobStep", new()
* {
* Action = new AzureNative.Sql.Inputs.JobStepActionArgs
* {
* Source = AzureNative.Sql.JobStepActionSource.Inline,
* Type = AzureNative.Sql.JobStepActionType.TSql,
* Value = "select 2",
* },
* Credential = "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred1",
* ExecutionOptions = new AzureNative.Sql.Inputs.JobStepExecutionOptionsArgs
* {
* InitialRetryIntervalSeconds = 11,
* MaximumRetryIntervalSeconds = 222,
* RetryAttempts = 42,
* RetryIntervalBackoffMultiplier = 3,
* TimeoutSeconds = 1234,
* },
* JobAgentName = "agent1",
* JobName = "job1",
* Output = new AzureNative.Sql.Inputs.JobStepOutputArgs
* {
* Credential = "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred0",
* DatabaseName = "database3",
* ResourceGroupName = "group3",
* SchemaName = "myschema1234",
* ServerName = "server3",
* SubscriptionId = "3501b905-a848-4b5d-96e8-b253f62d735a",
* TableName = "mytable5678",
* Type = AzureNative.Sql.JobStepOutputType.SqlDatabase,
* },
* ResourceGroupName = "group1",
* ServerName = "server1",
* StepId = 1,
* StepName = "step1",
* TargetGroup = "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/targetGroups/targetGroup1",
* });
* });
* ```
* ```go
* package main
* import (
* sql "github.com/pulumi/pulumi-azure-native-sdk/sql/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sql.NewJobStep(ctx, "jobStep", &sql.JobStepArgs{
* Action: &sql.JobStepActionArgs{
* Source: pulumi.String(sql.JobStepActionSourceInline),
* Type: pulumi.String(sql.JobStepActionTypeTSql),
* Value: pulumi.String("select 2"),
* },
* Credential: pulumi.String("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred1"),
* ExecutionOptions: &sql.JobStepExecutionOptionsArgs{
* InitialRetryIntervalSeconds: pulumi.Int(11),
* MaximumRetryIntervalSeconds: pulumi.Int(222),
* RetryAttempts: pulumi.Int(42),
* RetryIntervalBackoffMultiplier: pulumi.Float64(3),
* TimeoutSeconds: pulumi.Int(1234),
* },
* JobAgentName: pulumi.String("agent1"),
* JobName: pulumi.String("job1"),
* Output: &sql.JobStepOutputTypeArgs{
* Credential: pulumi.String("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred0"),
* DatabaseName: pulumi.String("database3"),
* ResourceGroupName: pulumi.String("group3"),
* SchemaName: pulumi.String("myschema1234"),
* ServerName: pulumi.String("server3"),
* SubscriptionId: pulumi.String("3501b905-a848-4b5d-96e8-b253f62d735a"),
* TableName: pulumi.String("mytable5678"),
* Type: pulumi.String(sql.JobStepOutputTypeSqlDatabase),
* },
* ResourceGroupName: pulumi.String("group1"),
* ServerName: pulumi.String("server1"),
* StepId: pulumi.Int(1),
* StepName: pulumi.String("step1"),
* TargetGroup: pulumi.String("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/targetGroups/targetGroup1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.sql.JobStep;
* import com.pulumi.azurenative.sql.JobStepArgs;
* import com.pulumi.azurenative.sql.inputs.JobStepActionArgs;
* import com.pulumi.azurenative.sql.inputs.JobStepExecutionOptionsArgs;
* import com.pulumi.azurenative.sql.inputs.JobStepOutputArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var jobStep = new JobStep("jobStep", JobStepArgs.builder()
* .action(JobStepActionArgs.builder()
* .source("Inline")
* .type("TSql")
* .value("select 2")
* .build())
* .credential("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred1")
* .executionOptions(JobStepExecutionOptionsArgs.builder()
* .initialRetryIntervalSeconds(11)
* .maximumRetryIntervalSeconds(222)
* .retryAttempts(42)
* .retryIntervalBackoffMultiplier(3)
* .timeoutSeconds(1234)
* .build())
* .jobAgentName("agent1")
* .jobName("job1")
* .output(JobStepOutputArgs.builder()
* .credential("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred0")
* .databaseName("database3")
* .resourceGroupName("group3")
* .schemaName("myschema1234")
* .serverName("server3")
* .subscriptionId("3501b905-a848-4b5d-96e8-b253f62d735a")
* .tableName("mytable5678")
* .type("SqlDatabase")
* .build())
* .resourceGroupName("group1")
* .serverName("server1")
* .stepId(1)
* .stepName("step1")
* .targetGroup("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/targetGroups/targetGroup1")
* .build());
* }
* }
* ```
* ### Create or update a job step with minimal properties specified.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var jobStep = new AzureNative.Sql.JobStep("jobStep", new()
* {
* Action = new AzureNative.Sql.Inputs.JobStepActionArgs
* {
* Value = "select 1",
* },
* Credential = "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred0",
* JobAgentName = "agent1",
* JobName = "job1",
* ResourceGroupName = "group1",
* ServerName = "server1",
* StepName = "step1",
* TargetGroup = "/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/targetGroups/targetGroup0",
* });
* });
* ```
* ```go
* package main
* import (
* sql "github.com/pulumi/pulumi-azure-native-sdk/sql/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sql.NewJobStep(ctx, "jobStep", &sql.JobStepArgs{
* Action: &sql.JobStepActionArgs{
* Value: pulumi.String("select 1"),
* },
* Credential: pulumi.String("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred0"),
* JobAgentName: pulumi.String("agent1"),
* JobName: pulumi.String("job1"),
* ResourceGroupName: pulumi.String("group1"),
* ServerName: pulumi.String("server1"),
* StepName: pulumi.String("step1"),
* TargetGroup: pulumi.String("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/targetGroups/targetGroup0"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.sql.JobStep;
* import com.pulumi.azurenative.sql.JobStepArgs;
* import com.pulumi.azurenative.sql.inputs.JobStepActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var jobStep = new JobStep("jobStep", JobStepArgs.builder()
* .action(JobStepActionArgs.builder()
* .value("select 1")
* .build())
* .credential("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/credentials/cred0")
* .jobAgentName("agent1")
* .jobName("job1")
* .resourceGroupName("group1")
* .serverName("server1")
* .stepName("step1")
* .targetGroup("/subscriptions/00000000-1111-2222-3333-444444444444/resourceGroups/group1/providers/Microsoft.Sql/servers/server1/jobAgents/agent1/targetGroups/targetGroup0")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:sql:JobStep step1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/jobAgents/{jobAgentName}/jobs/{jobName}/steps/{stepName}
* ```
* @property action The action payload of the job step.
* @property credential The resource ID of the job credential that will be used to connect to the targets.
* @property executionOptions Execution options for the job step.
* @property jobAgentName The name of the job agent.
* @property jobName The name of the job.
* @property output Output destination properties of the job step.
* @property resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @property serverName The name of the server.
* @property stepId The job step's index within the job. If not specified when creating the job step, it will be created as the last step. If not specified when updating the job step, the step id is not modified.
* @property stepName The name of the job step.
* @property targetGroup The resource ID of the target group that the job step will be executed on.
*/
public data class JobStepArgs(
public val action: Output? = null,
public val credential: Output? = null,
public val executionOptions: Output? = null,
public val jobAgentName: Output? = null,
public val jobName: Output? = null,
public val output: Output? = null,
public val resourceGroupName: Output? = null,
public val serverName: Output? = null,
public val stepId: Output? = null,
public val stepName: Output? = null,
public val targetGroup: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.sql.JobStepArgs =
com.pulumi.azurenative.sql.JobStepArgs.builder()
.action(action?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.credential(credential?.applyValue({ args0 -> args0 }))
.executionOptions(executionOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.jobAgentName(jobAgentName?.applyValue({ args0 -> args0 }))
.jobName(jobName?.applyValue({ args0 -> args0 }))
.output(output?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.serverName(serverName?.applyValue({ args0 -> args0 }))
.stepId(stepId?.applyValue({ args0 -> args0 }))
.stepName(stepName?.applyValue({ args0 -> args0 }))
.targetGroup(targetGroup?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [JobStepArgs].
*/
@PulumiTagMarker
public class JobStepArgsBuilder internal constructor() {
private var action: Output? = null
private var credential: Output? = null
private var executionOptions: Output? = null
private var jobAgentName: Output? = null
private var jobName: Output? = null
private var output: Output? = null
private var resourceGroupName: Output? = null
private var serverName: Output? = null
private var stepId: Output? = null
private var stepName: Output? = null
private var targetGroup: Output? = null
/**
* @param value The action payload of the job step.
*/
@JvmName("olylqlctfalubhjl")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value The resource ID of the job credential that will be used to connect to the targets.
*/
@JvmName("unpyqsqwyyoigqkb")
public suspend fun credential(`value`: Output) {
this.credential = value
}
/**
* @param value Execution options for the job step.
*/
@JvmName("bhiovyyeicfdjtnu")
public suspend fun executionOptions(`value`: Output) {
this.executionOptions = value
}
/**
* @param value The name of the job agent.
*/
@JvmName("xhrufgyigphkamft")
public suspend fun jobAgentName(`value`: Output) {
this.jobAgentName = value
}
/**
* @param value The name of the job.
*/
@JvmName("ktwdxkcrmokmsvhl")
public suspend fun jobName(`value`: Output) {
this.jobName = value
}
/**
* @param value Output destination properties of the job step.
*/
@JvmName("udgjaxmvrdrmtgwu")
public suspend fun output(`value`: Output) {
this.output = value
}
/**
* @param value The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
*/
@JvmName("ravdorgdwlpjdlqo")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the server.
*/
@JvmName("wafqqvqbyguaegrn")
public suspend fun serverName(`value`: Output) {
this.serverName = value
}
/**
* @param value The job step's index within the job. If not specified when creating the job step, it will be created as the last step. If not specified when updating the job step, the step id is not modified.
*/
@JvmName("nmkfqpyivlwnfayd")
public suspend fun stepId(`value`: Output) {
this.stepId = value
}
/**
* @param value The name of the job step.
*/
@JvmName("atbkqmdwmdodgawt")
public suspend fun stepName(`value`: Output) {
this.stepName = value
}
/**
* @param value The resource ID of the target group that the job step will be executed on.
*/
@JvmName("spgsntgtaylgdxro")
public suspend fun targetGroup(`value`: Output) {
this.targetGroup = value
}
/**
* @param value The action payload of the job step.
*/
@JvmName("oohfvglrhoexrjss")
public suspend fun action(`value`: JobStepActionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param argument The action payload of the job step.
*/
@JvmName("eklbbuvqykekwpxq")
public suspend fun action(argument: suspend JobStepActionArgsBuilder.() -> Unit) {
val toBeMapped = JobStepActionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.action = mapped
}
/**
* @param value The resource ID of the job credential that will be used to connect to the targets.
*/
@JvmName("ucknwsrfjcbhehod")
public suspend fun credential(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.credential = mapped
}
/**
* @param value Execution options for the job step.
*/
@JvmName("gpvylilavxioayqr")
public suspend fun executionOptions(`value`: JobStepExecutionOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.executionOptions = mapped
}
/**
* @param argument Execution options for the job step.
*/
@JvmName("kuaghlyewtqkncit")
public suspend fun executionOptions(argument: suspend JobStepExecutionOptionsArgsBuilder.() -> Unit) {
val toBeMapped = JobStepExecutionOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.executionOptions = mapped
}
/**
* @param value The name of the job agent.
*/
@JvmName("xbcgvldknroaxfnq")
public suspend fun jobAgentName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.jobAgentName = mapped
}
/**
* @param value The name of the job.
*/
@JvmName("qfnjotrjlprjaetp")
public suspend fun jobName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.jobName = mapped
}
/**
* @param value Output destination properties of the job step.
*/
@JvmName("adwwlswyvagmxyxv")
public suspend fun output(`value`: JobStepOutputArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.output = mapped
}
/**
* @param argument Output destination properties of the job step.
*/
@JvmName("nkjoebgebtgvpwux")
public suspend fun output(argument: suspend JobStepOutputArgsBuilder.() -> Unit) {
val toBeMapped = JobStepOutputArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.output = mapped
}
/**
* @param value The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
*/
@JvmName("bxcmbaubfyevbskr")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The name of the server.
*/
@JvmName("hsvklmatxkamxpqe")
public suspend fun serverName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serverName = mapped
}
/**
* @param value The job step's index within the job. If not specified when creating the job step, it will be created as the last step. If not specified when updating the job step, the step id is not modified.
*/
@JvmName("jdnpufrxvwuruwik")
public suspend fun stepId(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stepId = mapped
}
/**
* @param value The name of the job step.
*/
@JvmName("qqmninbbtkejpffb")
public suspend fun stepName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stepName = mapped
}
/**
* @param value The resource ID of the target group that the job step will be executed on.
*/
@JvmName("lvoctbkmoqpkjqmu")
public suspend fun targetGroup(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetGroup = mapped
}
internal fun build(): JobStepArgs = JobStepArgs(
action = action,
credential = credential,
executionOptions = executionOptions,
jobAgentName = jobAgentName,
jobName = jobName,
output = output,
resourceGroupName = resourceGroupName,
serverName = serverName,
stepId = stepId,
stepName = stepName,
targetGroup = targetGroup,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy