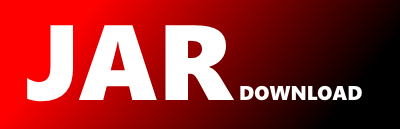
com.pulumi.azurenative.sql.kotlin.ServerAzureADAdministratorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.sql.kotlin
import com.pulumi.azurenative.sql.ServerAzureADAdministratorArgs.builder
import com.pulumi.azurenative.sql.kotlin.enums.AdministratorType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Azure Active Directory administrator.
* Azure REST API version: 2021-11-01. Prior API version in Azure Native 1.x: 2020-11-01-preview.
* Other available API versions: 2014-04-01, 2022-11-01-preview, 2023-02-01-preview, 2023-05-01-preview, 2023-08-01-preview.
* ## Example Usage
* ### Creates or updates an existing Azure Active Directory administrator.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var serverAzureADAdministrator = new AzureNative.Sql.ServerAzureADAdministrator("serverAzureADAdministrator", new()
* {
* AdministratorName = "ActiveDirectory",
* AdministratorType = AzureNative.Sql.AdministratorType.ActiveDirectory,
* Login = "[email protected]",
* ResourceGroupName = "sqlcrudtest-4799",
* ServerName = "sqlcrudtest-6440",
* Sid = "c6b82b90-a647-49cb-8a62-0d2d3cb7ac7c",
* TenantId = "c6b82b90-a647-49cb-8a62-0d2d3cb7ac7c",
* });
* });
* ```
* ```go
* package main
* import (
* sql "github.com/pulumi/pulumi-azure-native-sdk/sql/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := sql.NewServerAzureADAdministrator(ctx, "serverAzureADAdministrator", &sql.ServerAzureADAdministratorArgs{
* AdministratorName: pulumi.String("ActiveDirectory"),
* AdministratorType: pulumi.String(sql.AdministratorTypeActiveDirectory),
* Login: pulumi.String("[email protected]"),
* ResourceGroupName: pulumi.String("sqlcrudtest-4799"),
* ServerName: pulumi.String("sqlcrudtest-6440"),
* Sid: pulumi.String("c6b82b90-a647-49cb-8a62-0d2d3cb7ac7c"),
* TenantId: pulumi.String("c6b82b90-a647-49cb-8a62-0d2d3cb7ac7c"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.sql.ServerAzureADAdministrator;
* import com.pulumi.azurenative.sql.ServerAzureADAdministratorArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var serverAzureADAdministrator = new ServerAzureADAdministrator("serverAzureADAdministrator", ServerAzureADAdministratorArgs.builder()
* .administratorName("ActiveDirectory")
* .administratorType("ActiveDirectory")
* .login("[email protected]")
* .resourceGroupName("sqlcrudtest-4799")
* .serverName("sqlcrudtest-6440")
* .sid("c6b82b90-a647-49cb-8a62-0d2d3cb7ac7c")
* .tenantId("c6b82b90-a647-49cb-8a62-0d2d3cb7ac7c")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:sql:ServerAzureADAdministrator ActiveDirectory /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/administrators/{administratorName}
* ```
* @property administratorName The name of server active directory administrator.
* @property administratorType Type of the sever administrator.
* @property login Login name of the server administrator.
* @property resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @property serverName The name of the server.
* @property sid SID (object ID) of the server administrator.
* @property tenantId Tenant ID of the administrator.
*/
public data class ServerAzureADAdministratorArgs(
public val administratorName: Output? = null,
public val administratorType: Output>? = null,
public val login: Output? = null,
public val resourceGroupName: Output? = null,
public val serverName: Output? = null,
public val sid: Output? = null,
public val tenantId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.sql.ServerAzureADAdministratorArgs =
com.pulumi.azurenative.sql.ServerAzureADAdministratorArgs.builder()
.administratorName(administratorName?.applyValue({ args0 -> args0 }))
.administratorType(
administratorType?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.login(login?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.serverName(serverName?.applyValue({ args0 -> args0 }))
.sid(sid?.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServerAzureADAdministratorArgs].
*/
@PulumiTagMarker
public class ServerAzureADAdministratorArgsBuilder internal constructor() {
private var administratorName: Output? = null
private var administratorType: Output>? = null
private var login: Output? = null
private var resourceGroupName: Output? = null
private var serverName: Output? = null
private var sid: Output? = null
private var tenantId: Output? = null
/**
* @param value The name of server active directory administrator.
*/
@JvmName("ceduqhdyydgrowlb")
public suspend fun administratorName(`value`: Output) {
this.administratorName = value
}
/**
* @param value Type of the sever administrator.
*/
@JvmName("htquhrnfccdkgody")
public suspend fun administratorType(`value`: Output>) {
this.administratorType = value
}
/**
* @param value Login name of the server administrator.
*/
@JvmName("owferbinxlpbwswg")
public suspend fun login(`value`: Output) {
this.login = value
}
/**
* @param value The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
*/
@JvmName("qxiynjxnayiwmhtj")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the server.
*/
@JvmName("cakyrptcgwewpnpx")
public suspend fun serverName(`value`: Output) {
this.serverName = value
}
/**
* @param value SID (object ID) of the server administrator.
*/
@JvmName("gmdcjvespblpiaal")
public suspend fun sid(`value`: Output) {
this.sid = value
}
/**
* @param value Tenant ID of the administrator.
*/
@JvmName("tjrdecurcwvlnjbk")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value The name of server active directory administrator.
*/
@JvmName("ffjtcxxwaoctovlc")
public suspend fun administratorName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.administratorName = mapped
}
/**
* @param value Type of the sever administrator.
*/
@JvmName("hktjrfaccudhietx")
public suspend fun administratorType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.administratorType = mapped
}
/**
* @param value Type of the sever administrator.
*/
@JvmName("gtxhpkrtwfmehtnm")
public fun administratorType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.administratorType = mapped
}
/**
* @param value Type of the sever administrator.
*/
@JvmName("ygxrhdoaodyaogyl")
public fun administratorType(`value`: AdministratorType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.administratorType = mapped
}
/**
* @param value Login name of the server administrator.
*/
@JvmName("ubvlrrayxfnkgswf")
public suspend fun login(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.login = mapped
}
/**
* @param value The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
*/
@JvmName("syainglrxousewxy")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The name of the server.
*/
@JvmName("spxvmvqxpvwrgjst")
public suspend fun serverName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serverName = mapped
}
/**
* @param value SID (object ID) of the server administrator.
*/
@JvmName("amcfidxlhnsdkvnj")
public suspend fun sid(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sid = mapped
}
/**
* @param value Tenant ID of the administrator.
*/
@JvmName("qbyhfgoyusbcniei")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
internal fun build(): ServerAzureADAdministratorArgs = ServerAzureADAdministratorArgs(
administratorName = administratorName,
administratorType = administratorType,
login = login,
resourceGroupName = resourceGroupName,
serverName = serverName,
sid = sid,
tenantId = tenantId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy