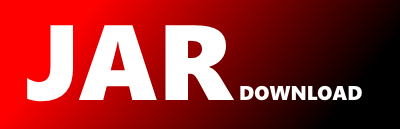
com.pulumi.azurenative.storage.kotlin.LocalUserArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.storage.kotlin
import com.pulumi.azurenative.storage.LocalUserArgs.builder
import com.pulumi.azurenative.storage.kotlin.inputs.PermissionScopeArgs
import com.pulumi.azurenative.storage.kotlin.inputs.PermissionScopeArgsBuilder
import com.pulumi.azurenative.storage.kotlin.inputs.SshPublicKeyArgs
import com.pulumi.azurenative.storage.kotlin.inputs.SshPublicKeyArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The local user associated with the storage accounts.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2021-08-01.
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
* ## Example Usage
* ### CreateLocalUser
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var localUser = new AzureNative.Storage.LocalUser("localUser", new()
* {
* AccountName = "sto2527",
* HasSshPassword = true,
* HomeDirectory = "homedirectory",
* PermissionScopes = new[]
* {
* new AzureNative.Storage.Inputs.PermissionScopeArgs
* {
* Permissions = "rwd",
* ResourceName = "share1",
* Service = "file",
* },
* new AzureNative.Storage.Inputs.PermissionScopeArgs
* {
* Permissions = "rw",
* ResourceName = "share2",
* Service = "file",
* },
* },
* ResourceGroupName = "res6977",
* SshAuthorizedKeys = new[]
* {
* new AzureNative.Storage.Inputs.SshPublicKeyArgs
* {
* Description = "key name",
* Key = "ssh-rsa keykeykeykeykey=",
* },
* },
* Username = "user1",
* });
* });
* ```
* ```go
* package main
* import (
* storage "github.com/pulumi/pulumi-azure-native-sdk/storage/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.NewLocalUser(ctx, "localUser", &storage.LocalUserArgs{
* AccountName: pulumi.String("sto2527"),
* HasSshPassword: pulumi.Bool(true),
* HomeDirectory: pulumi.String("homedirectory"),
* PermissionScopes: storage.PermissionScopeArray{
* &storage.PermissionScopeArgs{
* Permissions: pulumi.String("rwd"),
* ResourceName: pulumi.String("share1"),
* Service: pulumi.String("file"),
* },
* &storage.PermissionScopeArgs{
* Permissions: pulumi.String("rw"),
* ResourceName: pulumi.String("share2"),
* Service: pulumi.String("file"),
* },
* },
* ResourceGroupName: pulumi.String("res6977"),
* SshAuthorizedKeys: storage.SshPublicKeyArray{
* &storage.SshPublicKeyArgs{
* Description: pulumi.String("key name"),
* Key: pulumi.String("ssh-rsa keykeykeykeykey="),
* },
* },
* Username: pulumi.String("user1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.LocalUser;
* import com.pulumi.azurenative.storage.LocalUserArgs;
* import com.pulumi.azurenative.storage.inputs.PermissionScopeArgs;
* import com.pulumi.azurenative.storage.inputs.SshPublicKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var localUser = new LocalUser("localUser", LocalUserArgs.builder()
* .accountName("sto2527")
* .hasSshPassword(true)
* .homeDirectory("homedirectory")
* .permissionScopes(
* PermissionScopeArgs.builder()
* .permissions("rwd")
* .resourceName("share1")
* .service("file")
* .build(),
* PermissionScopeArgs.builder()
* .permissions("rw")
* .resourceName("share2")
* .service("file")
* .build())
* .resourceGroupName("res6977")
* .sshAuthorizedKeys(SshPublicKeyArgs.builder()
* .description("key name")
* .key("ssh-rsa keykeykeykeykey=")
* .build())
* .username("user1")
* .build());
* }
* }
* ```
* ### UpdateLocalUser
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var localUser = new AzureNative.Storage.LocalUser("localUser", new()
* {
* AccountName = "sto2527",
* HasSharedKey = false,
* HasSshKey = false,
* HasSshPassword = false,
* HomeDirectory = "homedirectory2",
* ResourceGroupName = "res6977",
* Username = "user1",
* });
* });
* ```
* ```go
* package main
* import (
* storage "github.com/pulumi/pulumi-azure-native-sdk/storage/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.NewLocalUser(ctx, "localUser", &storage.LocalUserArgs{
* AccountName: pulumi.String("sto2527"),
* HasSharedKey: pulumi.Bool(false),
* HasSshKey: pulumi.Bool(false),
* HasSshPassword: pulumi.Bool(false),
* HomeDirectory: pulumi.String("homedirectory2"),
* ResourceGroupName: pulumi.String("res6977"),
* Username: pulumi.String("user1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.LocalUser;
* import com.pulumi.azurenative.storage.LocalUserArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var localUser = new LocalUser("localUser", LocalUserArgs.builder()
* .accountName("sto2527")
* .hasSharedKey(false)
* .hasSshKey(false)
* .hasSshPassword(false)
* .homeDirectory("homedirectory2")
* .resourceGroupName("res6977")
* .username("user1")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:storage:LocalUser user1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Storage/storageAccounts/{accountName}/localUsers/{username}
* ```
* @property accountName The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @property hasSharedKey Indicates whether shared key exists. Set it to false to remove existing shared key.
* @property hasSshKey Indicates whether ssh key exists. Set it to false to remove existing SSH key.
* @property hasSshPassword Indicates whether ssh password exists. Set it to false to remove existing SSH password.
* @property homeDirectory Optional, local user home directory.
* @property permissionScopes The permission scopes of the local user.
* @property resourceGroupName The name of the resource group within the user's subscription. The name is case insensitive.
* @property sshAuthorizedKeys Optional, local user ssh authorized keys for SFTP.
* @property username The name of local user. The username must contain lowercase letters and numbers only. It must be unique only within the storage account.
*/
public data class LocalUserArgs(
public val accountName: Output? = null,
public val hasSharedKey: Output? = null,
public val hasSshKey: Output? = null,
public val hasSshPassword: Output? = null,
public val homeDirectory: Output? = null,
public val permissionScopes: Output>? = null,
public val resourceGroupName: Output? = null,
public val sshAuthorizedKeys: Output>? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.storage.LocalUserArgs =
com.pulumi.azurenative.storage.LocalUserArgs.builder()
.accountName(accountName?.applyValue({ args0 -> args0 }))
.hasSharedKey(hasSharedKey?.applyValue({ args0 -> args0 }))
.hasSshKey(hasSshKey?.applyValue({ args0 -> args0 }))
.hasSshPassword(hasSshPassword?.applyValue({ args0 -> args0 }))
.homeDirectory(homeDirectory?.applyValue({ args0 -> args0 }))
.permissionScopes(
permissionScopes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.sshAuthorizedKeys(
sshAuthorizedKeys?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LocalUserArgs].
*/
@PulumiTagMarker
public class LocalUserArgsBuilder internal constructor() {
private var accountName: Output? = null
private var hasSharedKey: Output? = null
private var hasSshKey: Output? = null
private var hasSshPassword: Output? = null
private var homeDirectory: Output? = null
private var permissionScopes: Output>? = null
private var resourceGroupName: Output? = null
private var sshAuthorizedKeys: Output>? = null
private var username: Output? = null
/**
* @param value The name of the storage account within the specified resource group. Storage account names must be between 3 and 24 characters in length and use numbers and lower-case letters only.
*/
@JvmName("motfoonbetobwaqu")
public suspend fun accountName(`value`: Output) {
this.accountName = value
}
/**
* @param value Indicates whether shared key exists. Set it to false to remove existing shared key.
*/
@JvmName("ttkhtybthcjcjibt")
public suspend fun hasSharedKey(`value`: Output) {
this.hasSharedKey = value
}
/**
* @param value Indicates whether ssh key exists. Set it to false to remove existing SSH key.
*/
@JvmName("qacttsjahvjrcktl")
public suspend fun hasSshKey(`value`: Output) {
this.hasSshKey = value
}
/**
* @param value Indicates whether ssh password exists. Set it to false to remove existing SSH password.
*/
@JvmName("qeyusjfxyjtorhfh")
public suspend fun hasSshPassword(`value`: Output) {
this.hasSshPassword = value
}
/**
* @param value Optional, local user home directory.
*/
@JvmName("onoqqgegeclssxii")
public suspend fun homeDirectory(`value`: Output) {
this.homeDirectory = value
}
/**
* @param value The permission scopes of the local user.
*/
@JvmName("omivubsffewlwyej")
public suspend fun permissionScopes(`value`: Output>) {
this.permissionScopes = value
}
@JvmName("dmmuttbntjcdrhqd")
public suspend fun permissionScopes(vararg values: Output) {
this.permissionScopes = Output.all(values.asList())
}
/**
* @param values The permission scopes of the local user.
*/
@JvmName("wwgsbewvypjomiqt")
public suspend fun permissionScopes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy