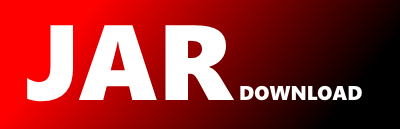
com.pulumi.azurenative.storage.kotlin.inputs.SmbSettingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.storage.kotlin.inputs
import com.pulumi.azurenative.storage.inputs.SmbSettingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Setting for SMB protocol
* @property authenticationMethods SMB authentication methods supported by server. Valid values are NTLMv2, Kerberos. Should be passed as a string with delimiter ';'.
* @property channelEncryption SMB channel encryption supported by server. Valid values are AES-128-CCM, AES-128-GCM, AES-256-GCM. Should be passed as a string with delimiter ';'.
* @property kerberosTicketEncryption Kerberos ticket encryption supported by server. Valid values are RC4-HMAC, AES-256. Should be passed as a string with delimiter ';'
* @property multichannel Multichannel setting. Applies to Premium FileStorage only.
* @property versions SMB protocol versions supported by server. Valid values are SMB2.1, SMB3.0, SMB3.1.1. Should be passed as a string with delimiter ';'.
*/
public data class SmbSettingArgs(
public val authenticationMethods: Output? = null,
public val channelEncryption: Output? = null,
public val kerberosTicketEncryption: Output? = null,
public val multichannel: Output? = null,
public val versions: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.storage.inputs.SmbSettingArgs =
com.pulumi.azurenative.storage.inputs.SmbSettingArgs.builder()
.authenticationMethods(authenticationMethods?.applyValue({ args0 -> args0 }))
.channelEncryption(channelEncryption?.applyValue({ args0 -> args0 }))
.kerberosTicketEncryption(kerberosTicketEncryption?.applyValue({ args0 -> args0 }))
.multichannel(multichannel?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.versions(versions?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SmbSettingArgs].
*/
@PulumiTagMarker
public class SmbSettingArgsBuilder internal constructor() {
private var authenticationMethods: Output? = null
private var channelEncryption: Output? = null
private var kerberosTicketEncryption: Output? = null
private var multichannel: Output? = null
private var versions: Output? = null
/**
* @param value SMB authentication methods supported by server. Valid values are NTLMv2, Kerberos. Should be passed as a string with delimiter ';'.
*/
@JvmName("pfvbuhpglupeogfw")
public suspend fun authenticationMethods(`value`: Output) {
this.authenticationMethods = value
}
/**
* @param value SMB channel encryption supported by server. Valid values are AES-128-CCM, AES-128-GCM, AES-256-GCM. Should be passed as a string with delimiter ';'.
*/
@JvmName("gtfgssswvjvovixn")
public suspend fun channelEncryption(`value`: Output) {
this.channelEncryption = value
}
/**
* @param value Kerberos ticket encryption supported by server. Valid values are RC4-HMAC, AES-256. Should be passed as a string with delimiter ';'
*/
@JvmName("ekjvttqqypmnhcum")
public suspend fun kerberosTicketEncryption(`value`: Output) {
this.kerberosTicketEncryption = value
}
/**
* @param value Multichannel setting. Applies to Premium FileStorage only.
*/
@JvmName("hhivwlajtspcapkj")
public suspend fun multichannel(`value`: Output) {
this.multichannel = value
}
/**
* @param value SMB protocol versions supported by server. Valid values are SMB2.1, SMB3.0, SMB3.1.1. Should be passed as a string with delimiter ';'.
*/
@JvmName("xfwwlrrcjccpbjme")
public suspend fun versions(`value`: Output) {
this.versions = value
}
/**
* @param value SMB authentication methods supported by server. Valid values are NTLMv2, Kerberos. Should be passed as a string with delimiter ';'.
*/
@JvmName("xmgxeakyfrbweetg")
public suspend fun authenticationMethods(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authenticationMethods = mapped
}
/**
* @param value SMB channel encryption supported by server. Valid values are AES-128-CCM, AES-128-GCM, AES-256-GCM. Should be passed as a string with delimiter ';'.
*/
@JvmName("ksjuujimjixqgrfc")
public suspend fun channelEncryption(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelEncryption = mapped
}
/**
* @param value Kerberos ticket encryption supported by server. Valid values are RC4-HMAC, AES-256. Should be passed as a string with delimiter ';'
*/
@JvmName("wpebmrkrdujrtaoa")
public suspend fun kerberosTicketEncryption(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kerberosTicketEncryption = mapped
}
/**
* @param value Multichannel setting. Applies to Premium FileStorage only.
*/
@JvmName("rdqefywlgyfwjwin")
public suspend fun multichannel(`value`: MultichannelArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.multichannel = mapped
}
/**
* @param argument Multichannel setting. Applies to Premium FileStorage only.
*/
@JvmName("aidlgrcxwqrryjga")
public suspend fun multichannel(argument: suspend MultichannelArgsBuilder.() -> Unit) {
val toBeMapped = MultichannelArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.multichannel = mapped
}
/**
* @param value SMB protocol versions supported by server. Valid values are SMB2.1, SMB3.0, SMB3.1.1. Should be passed as a string with delimiter ';'.
*/
@JvmName("vsxuvfjdlycuojiq")
public suspend fun versions(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.versions = mapped
}
internal fun build(): SmbSettingArgs = SmbSettingArgs(
authenticationMethods = authenticationMethods,
channelEncryption = channelEncryption,
kerberosTicketEncryption = kerberosTicketEncryption,
multichannel = multichannel,
versions = versions,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy