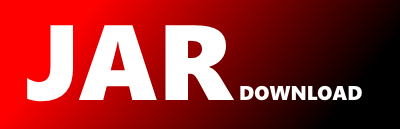
com.pulumi.azurenative.storagecache.kotlin.StoragecacheFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.storagecache.kotlin
import com.pulumi.azurenative.storagecache.StoragecacheFunctions.getAmlFilesystemPlain
import com.pulumi.azurenative.storagecache.StoragecacheFunctions.getCachePlain
import com.pulumi.azurenative.storagecache.StoragecacheFunctions.getImportJobPlain
import com.pulumi.azurenative.storagecache.StoragecacheFunctions.getRequiredAmlFSSubnetsSizePlain
import com.pulumi.azurenative.storagecache.StoragecacheFunctions.getStorageTargetPlain
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetAmlFilesystemPlainArgs
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetAmlFilesystemPlainArgsBuilder
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetCachePlainArgs
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetCachePlainArgsBuilder
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetImportJobPlainArgs
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetImportJobPlainArgsBuilder
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetRequiredAmlFSSubnetsSizePlainArgs
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetRequiredAmlFSSubnetsSizePlainArgsBuilder
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetStorageTargetPlainArgs
import com.pulumi.azurenative.storagecache.kotlin.inputs.GetStorageTargetPlainArgsBuilder
import com.pulumi.azurenative.storagecache.kotlin.inputs.SkuName
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetAmlFilesystemResult
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetCacheResult
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetImportJobResult
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetRequiredAmlFSSubnetsSizeResult
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetStorageTargetResult
import kotlinx.coroutines.future.await
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetAmlFilesystemResult.Companion.toKotlin as getAmlFilesystemResultToKotlin
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetCacheResult.Companion.toKotlin as getCacheResultToKotlin
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetImportJobResult.Companion.toKotlin as getImportJobResultToKotlin
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetRequiredAmlFSSubnetsSizeResult.Companion.toKotlin as getRequiredAmlFSSubnetsSizeResultToKotlin
import com.pulumi.azurenative.storagecache.kotlin.outputs.GetStorageTargetResult.Companion.toKotlin as getStorageTargetResultToKotlin
public object StoragecacheFunctions {
/**
* Returns an AML file system.
* Azure REST API version: 2023-05-01.
* @param argument null
* @return An AML file system instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getAmlFilesystem(argument: GetAmlFilesystemPlainArgs): GetAmlFilesystemResult =
getAmlFilesystemResultToKotlin(getAmlFilesystemPlain(argument.toJava()).await())
/**
* @see [getAmlFilesystem].
* @param amlFilesystemName Name for the AML file system. Allows alphanumerics, underscores, and hyphens. Start and end with alphanumeric.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An AML file system instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getAmlFilesystem(amlFilesystemName: String, resourceGroupName: String): GetAmlFilesystemResult {
val argument = GetAmlFilesystemPlainArgs(
amlFilesystemName = amlFilesystemName,
resourceGroupName = resourceGroupName,
)
return getAmlFilesystemResultToKotlin(getAmlFilesystemPlain(argument.toJava()).await())
}
/**
* @see [getAmlFilesystem].
* @param argument Builder for [com.pulumi.azurenative.storagecache.kotlin.inputs.GetAmlFilesystemPlainArgs].
* @return An AML file system instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getAmlFilesystem(argument: suspend GetAmlFilesystemPlainArgsBuilder.() -> Unit): GetAmlFilesystemResult {
val builder = GetAmlFilesystemPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAmlFilesystemResultToKotlin(getAmlFilesystemPlain(builtArgument.toJava()).await())
}
/**
* Returns a cache.
* Azure REST API version: 2023-05-01.
* Other available API versions: 2020-10-01, 2021-03-01, 2023-03-01-preview, 2023-11-01-preview, 2024-03-01.
* @param argument null
* @return A cache instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getCache(argument: GetCachePlainArgs): GetCacheResult =
getCacheResultToKotlin(getCachePlain(argument.toJava()).await())
/**
* @see [getCache].
* @param cacheName Name of cache. Length of name must not be greater than 80 and chars must be from the [-0-9a-zA-Z_] char class.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return A cache instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getCache(cacheName: String, resourceGroupName: String): GetCacheResult {
val argument = GetCachePlainArgs(
cacheName = cacheName,
resourceGroupName = resourceGroupName,
)
return getCacheResultToKotlin(getCachePlain(argument.toJava()).await())
}
/**
* @see [getCache].
* @param argument Builder for [com.pulumi.azurenative.storagecache.kotlin.inputs.GetCachePlainArgs].
* @return A cache instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getCache(argument: suspend GetCachePlainArgsBuilder.() -> Unit): GetCacheResult {
val builder = GetCachePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCacheResultToKotlin(getCachePlain(builtArgument.toJava()).await())
}
/**
* Returns an import job.
* Azure REST API version: 2024-03-01.
* @param argument null
* @return An import job instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getImportJob(argument: GetImportJobPlainArgs): GetImportJobResult =
getImportJobResultToKotlin(getImportJobPlain(argument.toJava()).await())
/**
* @see [getImportJob].
* @param amlFilesystemName Name for the AML file system. Allows alphanumerics, underscores, and hyphens. Start and end with alphanumeric.
* @param importJobName Name for the import job. Allows alphanumerics, underscores, and hyphens. Start and end with alphanumeric.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An import job instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getImportJob(
amlFilesystemName: String,
importJobName: String,
resourceGroupName: String,
): GetImportJobResult {
val argument = GetImportJobPlainArgs(
amlFilesystemName = amlFilesystemName,
importJobName = importJobName,
resourceGroupName = resourceGroupName,
)
return getImportJobResultToKotlin(getImportJobPlain(argument.toJava()).await())
}
/**
* @see [getImportJob].
* @param argument Builder for [com.pulumi.azurenative.storagecache.kotlin.inputs.GetImportJobPlainArgs].
* @return An import job instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
*/
public suspend fun getImportJob(argument: suspend GetImportJobPlainArgsBuilder.() -> Unit): GetImportJobResult {
val builder = GetImportJobPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getImportJobResultToKotlin(getImportJobPlain(builtArgument.toJava()).await())
}
/**
* Get the number of available IP addresses needed for the AML file system information provided.
* Azure REST API version: 2023-05-01.
* Other available API versions: 2023-03-01-preview, 2023-11-01-preview, 2024-03-01.
* @param argument null
* @return Information about the number of available IP addresses that are required for the AML file system.
*/
public suspend fun getRequiredAmlFSSubnetsSize(argument: GetRequiredAmlFSSubnetsSizePlainArgs): GetRequiredAmlFSSubnetsSizeResult =
getRequiredAmlFSSubnetsSizeResultToKotlin(getRequiredAmlFSSubnetsSizePlain(argument.toJava()).await())
/**
* @see [getRequiredAmlFSSubnetsSize].
* @param sku SKU for the resource.
* @param storageCapacityTiB The size of the AML file system, in TiB.
* @return Information about the number of available IP addresses that are required for the AML file system.
*/
public suspend fun getRequiredAmlFSSubnetsSize(
sku: SkuName? = null,
storageCapacityTiB: Double? =
null,
): GetRequiredAmlFSSubnetsSizeResult {
val argument = GetRequiredAmlFSSubnetsSizePlainArgs(
sku = sku,
storageCapacityTiB = storageCapacityTiB,
)
return getRequiredAmlFSSubnetsSizeResultToKotlin(getRequiredAmlFSSubnetsSizePlain(argument.toJava()).await())
}
/**
* @see [getRequiredAmlFSSubnetsSize].
* @param argument Builder for [com.pulumi.azurenative.storagecache.kotlin.inputs.GetRequiredAmlFSSubnetsSizePlainArgs].
* @return Information about the number of available IP addresses that are required for the AML file system.
*/
public suspend fun getRequiredAmlFSSubnetsSize(argument: suspend GetRequiredAmlFSSubnetsSizePlainArgsBuilder.() -> Unit): GetRequiredAmlFSSubnetsSizeResult {
val builder = GetRequiredAmlFSSubnetsSizePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRequiredAmlFSSubnetsSizeResultToKotlin(getRequiredAmlFSSubnetsSizePlain(builtArgument.toJava()).await())
}
/**
* Returns a Storage Target from a cache.
* Azure REST API version: 2023-05-01.
* Other available API versions: 2019-11-01, 2021-03-01, 2023-11-01-preview, 2024-03-01.
* @param argument null
* @return Type of the Storage Target.
*/
public suspend fun getStorageTarget(argument: GetStorageTargetPlainArgs): GetStorageTargetResult =
getStorageTargetResultToKotlin(getStorageTargetPlain(argument.toJava()).await())
/**
* @see [getStorageTarget].
* @param cacheName Name of cache. Length of name must not be greater than 80 and chars must be from the [-0-9a-zA-Z_] char class.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageTargetName Name of Storage Target.
* @return Type of the Storage Target.
*/
public suspend fun getStorageTarget(
cacheName: String,
resourceGroupName: String,
storageTargetName: String,
): GetStorageTargetResult {
val argument = GetStorageTargetPlainArgs(
cacheName = cacheName,
resourceGroupName = resourceGroupName,
storageTargetName = storageTargetName,
)
return getStorageTargetResultToKotlin(getStorageTargetPlain(argument.toJava()).await())
}
/**
* @see [getStorageTarget].
* @param argument Builder for [com.pulumi.azurenative.storagecache.kotlin.inputs.GetStorageTargetPlainArgs].
* @return Type of the Storage Target.
*/
public suspend fun getStorageTarget(argument: suspend GetStorageTargetPlainArgsBuilder.() -> Unit): GetStorageTargetResult {
val builder = GetStorageTargetPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStorageTargetResultToKotlin(getStorageTargetPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy