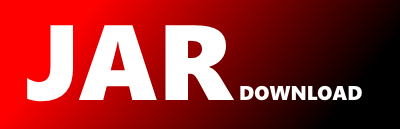
com.pulumi.azurenative.storagecache.kotlin.outputs.GetCacheResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.storagecache.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A cache instance. Follows Azure Resource Manager standards: https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/resource-api-reference.md
* @property cacheSizeGB The size of this Cache, in GB.
* @property directoryServicesSettings Specifies Directory Services settings of the cache.
* @property encryptionSettings Specifies encryption settings of the cache.
* @property health Health of the cache.
* @property id Resource ID of the cache.
* @property identity The identity of the cache, if configured.
* @property location Region name string.
* @property mountAddresses Array of IPv4 addresses that can be used by clients mounting this cache.
* @property name Name of cache.
* @property networkSettings Specifies network settings of the cache.
* @property primingJobs Specifies the priming jobs defined in the cache.
* @property provisioningState ARM provisioning state, see https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/Addendum.md#provisioningstate-property
* @property securitySettings Specifies security settings of the cache.
* @property sku SKU for the cache.
* @property spaceAllocation Specifies the space allocation percentage for each storage target in the cache.
* @property subnet Subnet used for the cache.
* @property systemData The system meta data relating to this resource.
* @property tags Resource tags.
* @property type Type of the cache; Microsoft.StorageCache/Cache
* @property upgradeSettings Upgrade settings of the cache.
* @property upgradeStatus Upgrade status of the cache.
* @property zones Availability zones for resources. This field should only contain a single element in the array.
*/
public data class GetCacheResult(
public val cacheSizeGB: Int? = null,
public val directoryServicesSettings: CacheDirectorySettingsResponse? = null,
public val encryptionSettings: CacheEncryptionSettingsResponse? = null,
public val health: CacheHealthResponse,
public val id: String,
public val identity: CacheIdentityResponse? = null,
public val location: String? = null,
public val mountAddresses: List,
public val name: String,
public val networkSettings: CacheNetworkSettingsResponse? = null,
public val primingJobs: List,
public val provisioningState: String,
public val securitySettings: CacheSecuritySettingsResponse? = null,
public val sku: CacheResponseSku? = null,
public val spaceAllocation: List,
public val subnet: String? = null,
public val systemData: SystemDataResponse,
public val tags: Map? = null,
public val type: String,
public val upgradeSettings: CacheUpgradeSettingsResponse? = null,
public val upgradeStatus: CacheUpgradeStatusResponse,
public val zones: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.storagecache.outputs.GetCacheResult): GetCacheResult = GetCacheResult(
cacheSizeGB = javaType.cacheSizeGB().map({ args0 -> args0 }).orElse(null),
directoryServicesSettings = javaType.directoryServicesSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheDirectorySettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
encryptionSettings = javaType.encryptionSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheEncryptionSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
health = javaType.health().let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheHealthResponse.Companion.toKotlin(args0)
}),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
location = javaType.location().map({ args0 -> args0 }).orElse(null),
mountAddresses = javaType.mountAddresses().map({ args0 -> args0 }),
name = javaType.name(),
networkSettings = javaType.networkSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheNetworkSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
primingJobs = javaType.primingJobs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.PrimingJobResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
securitySettings = javaType.securitySettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheSecuritySettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
sku = javaType.sku().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheResponseSku.Companion.toKotlin(args0)
})
}).orElse(null),
spaceAllocation = javaType.spaceAllocation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.StorageTargetSpaceAllocationResponse.Companion.toKotlin(args0)
})
}),
subnet = javaType.subnet().map({ args0 -> args0 }).orElse(null),
systemData = javaType.systemData().let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.SystemDataResponse.Companion.toKotlin(args0)
}),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
upgradeSettings = javaType.upgradeSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheUpgradeSettingsResponse.Companion.toKotlin(args0)
})
}).orElse(null),
upgradeStatus = javaType.upgradeStatus().let({ args0 ->
com.pulumi.azurenative.storagecache.kotlin.outputs.CacheUpgradeStatusResponse.Companion.toKotlin(args0)
}),
zones = javaType.zones().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy