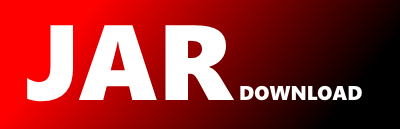
com.pulumi.azurenative.storagemover.kotlin.StoragemoverFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.storagemover.kotlin
import com.pulumi.azurenative.storagemover.StoragemoverFunctions.getAgentPlain
import com.pulumi.azurenative.storagemover.StoragemoverFunctions.getEndpointPlain
import com.pulumi.azurenative.storagemover.StoragemoverFunctions.getJobDefinitionPlain
import com.pulumi.azurenative.storagemover.StoragemoverFunctions.getProjectPlain
import com.pulumi.azurenative.storagemover.StoragemoverFunctions.getStorageMoverPlain
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetAgentPlainArgs
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetAgentPlainArgsBuilder
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetEndpointPlainArgs
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetEndpointPlainArgsBuilder
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetJobDefinitionPlainArgs
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetJobDefinitionPlainArgsBuilder
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetProjectPlainArgs
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetProjectPlainArgsBuilder
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetStorageMoverPlainArgs
import com.pulumi.azurenative.storagemover.kotlin.inputs.GetStorageMoverPlainArgsBuilder
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetAgentResult
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetEndpointResult
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetJobDefinitionResult
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetProjectResult
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetStorageMoverResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetAgentResult.Companion.toKotlin as getAgentResultToKotlin
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetEndpointResult.Companion.toKotlin as getEndpointResultToKotlin
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetJobDefinitionResult.Companion.toKotlin as getJobDefinitionResultToKotlin
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetProjectResult.Companion.toKotlin as getProjectResultToKotlin
import com.pulumi.azurenative.storagemover.kotlin.outputs.GetStorageMoverResult.Companion.toKotlin as getStorageMoverResultToKotlin
public object StoragemoverFunctions {
/**
* Gets an Agent resource.
* Azure REST API version: 2023-03-01.
* Other available API versions: 2023-07-01-preview, 2023-10-01, 2024-07-01.
* @param argument null
* @return The Agent resource.
*/
public suspend fun getAgent(argument: GetAgentPlainArgs): GetAgentResult =
getAgentResultToKotlin(getAgentPlain(argument.toJava()).await())
/**
* @see [getAgent].
* @param agentName The name of the Agent resource.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageMoverName The name of the Storage Mover resource.
* @return The Agent resource.
*/
public suspend fun getAgent(
agentName: String,
resourceGroupName: String,
storageMoverName: String,
): GetAgentResult {
val argument = GetAgentPlainArgs(
agentName = agentName,
resourceGroupName = resourceGroupName,
storageMoverName = storageMoverName,
)
return getAgentResultToKotlin(getAgentPlain(argument.toJava()).await())
}
/**
* @see [getAgent].
* @param argument Builder for [com.pulumi.azurenative.storagemover.kotlin.inputs.GetAgentPlainArgs].
* @return The Agent resource.
*/
public suspend fun getAgent(argument: suspend GetAgentPlainArgsBuilder.() -> Unit): GetAgentResult {
val builder = GetAgentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAgentResultToKotlin(getAgentPlain(builtArgument.toJava()).await())
}
/**
* Gets an Endpoint resource.
* Azure REST API version: 2023-03-01.
* Other available API versions: 2023-07-01-preview, 2023-10-01, 2024-07-01.
* @param argument null
* @return The Endpoint resource, which contains information about file sources and targets.
*/
public suspend fun getEndpoint(argument: GetEndpointPlainArgs): GetEndpointResult =
getEndpointResultToKotlin(getEndpointPlain(argument.toJava()).await())
/**
* @see [getEndpoint].
* @param endpointName The name of the Endpoint resource.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageMoverName The name of the Storage Mover resource.
* @return The Endpoint resource, which contains information about file sources and targets.
*/
public suspend fun getEndpoint(
endpointName: String,
resourceGroupName: String,
storageMoverName: String,
): GetEndpointResult {
val argument = GetEndpointPlainArgs(
endpointName = endpointName,
resourceGroupName = resourceGroupName,
storageMoverName = storageMoverName,
)
return getEndpointResultToKotlin(getEndpointPlain(argument.toJava()).await())
}
/**
* @see [getEndpoint].
* @param argument Builder for [com.pulumi.azurenative.storagemover.kotlin.inputs.GetEndpointPlainArgs].
* @return The Endpoint resource, which contains information about file sources and targets.
*/
public suspend fun getEndpoint(argument: suspend GetEndpointPlainArgsBuilder.() -> Unit): GetEndpointResult {
val builder = GetEndpointPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEndpointResultToKotlin(getEndpointPlain(builtArgument.toJava()).await())
}
/**
* Gets a Job Definition resource.
* Azure REST API version: 2023-03-01.
* Other available API versions: 2023-07-01-preview, 2023-10-01, 2024-07-01.
* @param argument null
* @return The Job Definition resource.
*/
public suspend fun getJobDefinition(argument: GetJobDefinitionPlainArgs): GetJobDefinitionResult =
getJobDefinitionResultToKotlin(getJobDefinitionPlain(argument.toJava()).await())
/**
* @see [getJobDefinition].
* @param jobDefinitionName The name of the Job Definition resource.
* @param projectName The name of the Project resource.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageMoverName The name of the Storage Mover resource.
* @return The Job Definition resource.
*/
public suspend fun getJobDefinition(
jobDefinitionName: String,
projectName: String,
resourceGroupName: String,
storageMoverName: String,
): GetJobDefinitionResult {
val argument = GetJobDefinitionPlainArgs(
jobDefinitionName = jobDefinitionName,
projectName = projectName,
resourceGroupName = resourceGroupName,
storageMoverName = storageMoverName,
)
return getJobDefinitionResultToKotlin(getJobDefinitionPlain(argument.toJava()).await())
}
/**
* @see [getJobDefinition].
* @param argument Builder for [com.pulumi.azurenative.storagemover.kotlin.inputs.GetJobDefinitionPlainArgs].
* @return The Job Definition resource.
*/
public suspend fun getJobDefinition(argument: suspend GetJobDefinitionPlainArgsBuilder.() -> Unit): GetJobDefinitionResult {
val builder = GetJobDefinitionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getJobDefinitionResultToKotlin(getJobDefinitionPlain(builtArgument.toJava()).await())
}
/**
* Gets a Project resource.
* Azure REST API version: 2023-03-01.
* Other available API versions: 2023-07-01-preview, 2023-10-01, 2024-07-01.
* @param argument null
* @return The Project resource.
*/
public suspend fun getProject(argument: GetProjectPlainArgs): GetProjectResult =
getProjectResultToKotlin(getProjectPlain(argument.toJava()).await())
/**
* @see [getProject].
* @param projectName The name of the Project resource.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageMoverName The name of the Storage Mover resource.
* @return The Project resource.
*/
public suspend fun getProject(
projectName: String,
resourceGroupName: String,
storageMoverName: String,
): GetProjectResult {
val argument = GetProjectPlainArgs(
projectName = projectName,
resourceGroupName = resourceGroupName,
storageMoverName = storageMoverName,
)
return getProjectResultToKotlin(getProjectPlain(argument.toJava()).await())
}
/**
* @see [getProject].
* @param argument Builder for [com.pulumi.azurenative.storagemover.kotlin.inputs.GetProjectPlainArgs].
* @return The Project resource.
*/
public suspend fun getProject(argument: suspend GetProjectPlainArgsBuilder.() -> Unit): GetProjectResult {
val builder = GetProjectPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProjectResultToKotlin(getProjectPlain(builtArgument.toJava()).await())
}
/**
* Gets a Storage Mover resource.
* Azure REST API version: 2023-03-01.
* Other available API versions: 2023-07-01-preview, 2023-10-01, 2024-07-01.
* @param argument null
* @return The Storage Mover resource, which is a container for a group of Agents, Projects, and Endpoints.
*/
public suspend fun getStorageMover(argument: GetStorageMoverPlainArgs): GetStorageMoverResult =
getStorageMoverResultToKotlin(getStorageMoverPlain(argument.toJava()).await())
/**
* @see [getStorageMover].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageMoverName The name of the Storage Mover resource.
* @return The Storage Mover resource, which is a container for a group of Agents, Projects, and Endpoints.
*/
public suspend fun getStorageMover(resourceGroupName: String, storageMoverName: String): GetStorageMoverResult {
val argument = GetStorageMoverPlainArgs(
resourceGroupName = resourceGroupName,
storageMoverName = storageMoverName,
)
return getStorageMoverResultToKotlin(getStorageMoverPlain(argument.toJava()).await())
}
/**
* @see [getStorageMover].
* @param argument Builder for [com.pulumi.azurenative.storagemover.kotlin.inputs.GetStorageMoverPlainArgs].
* @return The Storage Mover resource, which is a container for a group of Agents, Projects, and Endpoints.
*/
public suspend fun getStorageMover(argument: suspend GetStorageMoverPlainArgsBuilder.() -> Unit): GetStorageMoverResult {
val builder = GetStorageMoverPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStorageMoverResultToKotlin(getStorageMoverPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy