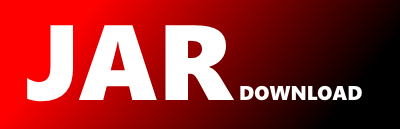
com.pulumi.azurenative.storagesync.kotlin.StoragesyncFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.storagesync.kotlin
import com.pulumi.azurenative.storagesync.StoragesyncFunctions.getCloudEndpointPlain
import com.pulumi.azurenative.storagesync.StoragesyncFunctions.getPrivateEndpointConnectionPlain
import com.pulumi.azurenative.storagesync.StoragesyncFunctions.getRegisteredServerPlain
import com.pulumi.azurenative.storagesync.StoragesyncFunctions.getServerEndpointPlain
import com.pulumi.azurenative.storagesync.StoragesyncFunctions.getStorageSyncServicePlain
import com.pulumi.azurenative.storagesync.StoragesyncFunctions.getSyncGroupPlain
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetCloudEndpointPlainArgs
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetCloudEndpointPlainArgsBuilder
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetPrivateEndpointConnectionPlainArgsBuilder
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetRegisteredServerPlainArgs
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetRegisteredServerPlainArgsBuilder
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetServerEndpointPlainArgs
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetServerEndpointPlainArgsBuilder
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetStorageSyncServicePlainArgs
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetStorageSyncServicePlainArgsBuilder
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetSyncGroupPlainArgs
import com.pulumi.azurenative.storagesync.kotlin.inputs.GetSyncGroupPlainArgsBuilder
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetCloudEndpointResult
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetPrivateEndpointConnectionResult
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetRegisteredServerResult
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetServerEndpointResult
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetStorageSyncServiceResult
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetSyncGroupResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetCloudEndpointResult.Companion.toKotlin as getCloudEndpointResultToKotlin
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetPrivateEndpointConnectionResult.Companion.toKotlin as getPrivateEndpointConnectionResultToKotlin
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetRegisteredServerResult.Companion.toKotlin as getRegisteredServerResultToKotlin
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetServerEndpointResult.Companion.toKotlin as getServerEndpointResultToKotlin
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetStorageSyncServiceResult.Companion.toKotlin as getStorageSyncServiceResultToKotlin
import com.pulumi.azurenative.storagesync.kotlin.outputs.GetSyncGroupResult.Companion.toKotlin as getSyncGroupResultToKotlin
public object StoragesyncFunctions {
/**
* Get a given CloudEndpoint.
* Azure REST API version: 2022-06-01.
* Other available API versions: 2017-06-05-preview, 2018-04-02, 2018-07-01, 2018-10-01, 2022-09-01.
* @param argument null
* @return Cloud Endpoint object.
*/
public suspend fun getCloudEndpoint(argument: GetCloudEndpointPlainArgs): GetCloudEndpointResult =
getCloudEndpointResultToKotlin(getCloudEndpointPlain(argument.toJava()).await())
/**
* @see [getCloudEndpoint].
* @param cloudEndpointName Name of Cloud Endpoint object.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageSyncServiceName Name of Storage Sync Service resource.
* @param syncGroupName Name of Sync Group resource.
* @return Cloud Endpoint object.
*/
public suspend fun getCloudEndpoint(
cloudEndpointName: String,
resourceGroupName: String,
storageSyncServiceName: String,
syncGroupName: String,
): GetCloudEndpointResult {
val argument = GetCloudEndpointPlainArgs(
cloudEndpointName = cloudEndpointName,
resourceGroupName = resourceGroupName,
storageSyncServiceName = storageSyncServiceName,
syncGroupName = syncGroupName,
)
return getCloudEndpointResultToKotlin(getCloudEndpointPlain(argument.toJava()).await())
}
/**
* @see [getCloudEndpoint].
* @param argument Builder for [com.pulumi.azurenative.storagesync.kotlin.inputs.GetCloudEndpointPlainArgs].
* @return Cloud Endpoint object.
*/
public suspend fun getCloudEndpoint(argument: suspend GetCloudEndpointPlainArgsBuilder.() -> Unit): GetCloudEndpointResult {
val builder = GetCloudEndpointPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCloudEndpointResultToKotlin(getCloudEndpointPlain(builtArgument.toJava()).await())
}
/**
* Gets the specified private endpoint connection associated with the storage sync service.
* Azure REST API version: 2022-06-01.
* Other available API versions: 2022-09-01.
* @param argument null
* @return The Private Endpoint Connection resource.
*/
public suspend fun getPrivateEndpointConnection(argument: GetPrivateEndpointConnectionPlainArgs): GetPrivateEndpointConnectionResult =
getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
/**
* @see [getPrivateEndpointConnection].
* @param privateEndpointConnectionName The name of the private endpoint connection associated with the Azure resource
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageSyncServiceName The name of the storage sync service name within the specified resource group.
* @return The Private Endpoint Connection resource.
*/
public suspend fun getPrivateEndpointConnection(
privateEndpointConnectionName: String,
resourceGroupName: String,
storageSyncServiceName: String,
): GetPrivateEndpointConnectionResult {
val argument = GetPrivateEndpointConnectionPlainArgs(
privateEndpointConnectionName = privateEndpointConnectionName,
resourceGroupName = resourceGroupName,
storageSyncServiceName = storageSyncServiceName,
)
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
}
/**
* @see [getPrivateEndpointConnection].
* @param argument Builder for [com.pulumi.azurenative.storagesync.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs].
* @return The Private Endpoint Connection resource.
*/
public suspend fun getPrivateEndpointConnection(argument: suspend GetPrivateEndpointConnectionPlainArgsBuilder.() -> Unit): GetPrivateEndpointConnectionResult {
val builder = GetPrivateEndpointConnectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(builtArgument.toJava()).await())
}
/**
* Get a given registered server.
* Azure REST API version: 2022-06-01.
* Other available API versions: 2017-06-05-preview, 2018-04-02, 2018-07-01, 2022-09-01.
* @param argument null
* @return Registered Server resource.
*/
public suspend fun getRegisteredServer(argument: GetRegisteredServerPlainArgs): GetRegisteredServerResult =
getRegisteredServerResultToKotlin(getRegisteredServerPlain(argument.toJava()).await())
/**
* @see [getRegisteredServer].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serverId GUID identifying the on-premises server.
* @param storageSyncServiceName Name of Storage Sync Service resource.
* @return Registered Server resource.
*/
public suspend fun getRegisteredServer(
resourceGroupName: String,
serverId: String,
storageSyncServiceName: String,
): GetRegisteredServerResult {
val argument = GetRegisteredServerPlainArgs(
resourceGroupName = resourceGroupName,
serverId = serverId,
storageSyncServiceName = storageSyncServiceName,
)
return getRegisteredServerResultToKotlin(getRegisteredServerPlain(argument.toJava()).await())
}
/**
* @see [getRegisteredServer].
* @param argument Builder for [com.pulumi.azurenative.storagesync.kotlin.inputs.GetRegisteredServerPlainArgs].
* @return Registered Server resource.
*/
public suspend fun getRegisteredServer(argument: suspend GetRegisteredServerPlainArgsBuilder.() -> Unit): GetRegisteredServerResult {
val builder = GetRegisteredServerPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRegisteredServerResultToKotlin(getRegisteredServerPlain(builtArgument.toJava()).await())
}
/**
* Get a ServerEndpoint.
* Azure REST API version: 2022-06-01.
* Other available API versions: 2017-06-05-preview, 2018-04-02, 2018-07-01, 2018-10-01, 2019-10-01, 2022-09-01.
* @param argument null
* @return Server Endpoint object.
*/
public suspend fun getServerEndpoint(argument: GetServerEndpointPlainArgs): GetServerEndpointResult =
getServerEndpointResultToKotlin(getServerEndpointPlain(argument.toJava()).await())
/**
* @see [getServerEndpoint].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param serverEndpointName Name of Server Endpoint object.
* @param storageSyncServiceName Name of Storage Sync Service resource.
* @param syncGroupName Name of Sync Group resource.
* @return Server Endpoint object.
*/
public suspend fun getServerEndpoint(
resourceGroupName: String,
serverEndpointName: String,
storageSyncServiceName: String,
syncGroupName: String,
): GetServerEndpointResult {
val argument = GetServerEndpointPlainArgs(
resourceGroupName = resourceGroupName,
serverEndpointName = serverEndpointName,
storageSyncServiceName = storageSyncServiceName,
syncGroupName = syncGroupName,
)
return getServerEndpointResultToKotlin(getServerEndpointPlain(argument.toJava()).await())
}
/**
* @see [getServerEndpoint].
* @param argument Builder for [com.pulumi.azurenative.storagesync.kotlin.inputs.GetServerEndpointPlainArgs].
* @return Server Endpoint object.
*/
public suspend fun getServerEndpoint(argument: suspend GetServerEndpointPlainArgsBuilder.() -> Unit): GetServerEndpointResult {
val builder = GetServerEndpointPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getServerEndpointResultToKotlin(getServerEndpointPlain(builtArgument.toJava()).await())
}
/**
* Get a given StorageSyncService.
* Azure REST API version: 2022-06-01.
* Other available API versions: 2017-06-05-preview, 2019-10-01, 2022-09-01.
* @param argument null
* @return Storage Sync Service object.
*/
public suspend fun getStorageSyncService(argument: GetStorageSyncServicePlainArgs): GetStorageSyncServiceResult =
getStorageSyncServiceResultToKotlin(getStorageSyncServicePlain(argument.toJava()).await())
/**
* @see [getStorageSyncService].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageSyncServiceName Name of Storage Sync Service resource.
* @return Storage Sync Service object.
*/
public suspend fun getStorageSyncService(
resourceGroupName: String,
storageSyncServiceName: String,
): GetStorageSyncServiceResult {
val argument = GetStorageSyncServicePlainArgs(
resourceGroupName = resourceGroupName,
storageSyncServiceName = storageSyncServiceName,
)
return getStorageSyncServiceResultToKotlin(getStorageSyncServicePlain(argument.toJava()).await())
}
/**
* @see [getStorageSyncService].
* @param argument Builder for [com.pulumi.azurenative.storagesync.kotlin.inputs.GetStorageSyncServicePlainArgs].
* @return Storage Sync Service object.
*/
public suspend fun getStorageSyncService(argument: suspend GetStorageSyncServicePlainArgsBuilder.() -> Unit): GetStorageSyncServiceResult {
val builder = GetStorageSyncServicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStorageSyncServiceResultToKotlin(getStorageSyncServicePlain(builtArgument.toJava()).await())
}
/**
* Get a given SyncGroup.
* Azure REST API version: 2022-06-01.
* Other available API versions: 2017-06-05-preview, 2018-04-02, 2022-09-01.
* @param argument null
* @return Sync Group object.
*/
public suspend fun getSyncGroup(argument: GetSyncGroupPlainArgs): GetSyncGroupResult =
getSyncGroupResultToKotlin(getSyncGroupPlain(argument.toJava()).await())
/**
* @see [getSyncGroup].
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param storageSyncServiceName Name of Storage Sync Service resource.
* @param syncGroupName Name of Sync Group resource.
* @return Sync Group object.
*/
public suspend fun getSyncGroup(
resourceGroupName: String,
storageSyncServiceName: String,
syncGroupName: String,
): GetSyncGroupResult {
val argument = GetSyncGroupPlainArgs(
resourceGroupName = resourceGroupName,
storageSyncServiceName = storageSyncServiceName,
syncGroupName = syncGroupName,
)
return getSyncGroupResultToKotlin(getSyncGroupPlain(argument.toJava()).await())
}
/**
* @see [getSyncGroup].
* @param argument Builder for [com.pulumi.azurenative.storagesync.kotlin.inputs.GetSyncGroupPlainArgs].
* @return Sync Group object.
*/
public suspend fun getSyncGroup(argument: suspend GetSyncGroupPlainArgsBuilder.() -> Unit): GetSyncGroupResult {
val builder = GetSyncGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSyncGroupResultToKotlin(getSyncGroupPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy