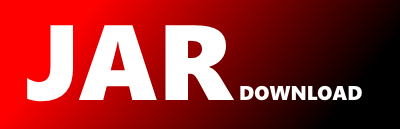
com.pulumi.azurenative.streamanalytics.kotlin.StreamanalyticsFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.streamanalytics.kotlin
import com.pulumi.azurenative.streamanalytics.StreamanalyticsFunctions.getClusterPlain
import com.pulumi.azurenative.streamanalytics.StreamanalyticsFunctions.getFunctionPlain
import com.pulumi.azurenative.streamanalytics.StreamanalyticsFunctions.getInputPlain
import com.pulumi.azurenative.streamanalytics.StreamanalyticsFunctions.getOutputPlain
import com.pulumi.azurenative.streamanalytics.StreamanalyticsFunctions.getPrivateEndpointPlain
import com.pulumi.azurenative.streamanalytics.StreamanalyticsFunctions.getStreamingJobPlain
import com.pulumi.azurenative.streamanalytics.StreamanalyticsFunctions.listClusterStreamingJobsPlain
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetClusterPlainArgs
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetClusterPlainArgsBuilder
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetFunctionPlainArgs
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetFunctionPlainArgsBuilder
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetInputPlainArgs
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetInputPlainArgsBuilder
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetOutputPlainArgs
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetOutputPlainArgsBuilder
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetPrivateEndpointPlainArgs
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetPrivateEndpointPlainArgsBuilder
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetStreamingJobPlainArgs
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetStreamingJobPlainArgsBuilder
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.ListClusterStreamingJobsPlainArgs
import com.pulumi.azurenative.streamanalytics.kotlin.inputs.ListClusterStreamingJobsPlainArgsBuilder
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetClusterResult
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetFunctionResult
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetInputResult
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetOutputResult
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetPrivateEndpointResult
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetStreamingJobResult
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.ListClusterStreamingJobsResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetClusterResult.Companion.toKotlin as getClusterResultToKotlin
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetFunctionResult.Companion.toKotlin as getFunctionResultToKotlin
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetInputResult.Companion.toKotlin as getInputResultToKotlin
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetOutputResult.Companion.toKotlin as getOutputResultToKotlin
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetPrivateEndpointResult.Companion.toKotlin as getPrivateEndpointResultToKotlin
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.GetStreamingJobResult.Companion.toKotlin as getStreamingJobResultToKotlin
import com.pulumi.azurenative.streamanalytics.kotlin.outputs.ListClusterStreamingJobsResult.Companion.toKotlin as listClusterStreamingJobsResultToKotlin
public object StreamanalyticsFunctions {
/**
* Gets information about the specified cluster.
* Azure REST API version: 2020-03-01.
* Other available API versions: 2020-03-01-preview.
* @param argument null
* @return A Stream Analytics Cluster object
*/
public suspend fun getCluster(argument: GetClusterPlainArgs): GetClusterResult =
getClusterResultToKotlin(getClusterPlain(argument.toJava()).await())
/**
* @see [getCluster].
* @param clusterName The name of the cluster.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return A Stream Analytics Cluster object
*/
public suspend fun getCluster(clusterName: String, resourceGroupName: String): GetClusterResult {
val argument = GetClusterPlainArgs(
clusterName = clusterName,
resourceGroupName = resourceGroupName,
)
return getClusterResultToKotlin(getClusterPlain(argument.toJava()).await())
}
/**
* @see [getCluster].
* @param argument Builder for [com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetClusterPlainArgs].
* @return A Stream Analytics Cluster object
*/
public suspend fun getCluster(argument: suspend GetClusterPlainArgsBuilder.() -> Unit): GetClusterResult {
val builder = GetClusterPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getClusterResultToKotlin(getClusterPlain(builtArgument.toJava()).await())
}
/**
* Gets details about the specified function.
* Azure REST API version: 2020-03-01.
* Other available API versions: 2016-03-01, 2021-10-01-preview.
* @param argument null
* @return A function object, containing all information associated with the named function. All functions are contained under a streaming job.
*/
public suspend fun getFunction(argument: GetFunctionPlainArgs): GetFunctionResult =
getFunctionResultToKotlin(getFunctionPlain(argument.toJava()).await())
/**
* @see [getFunction].
* @param functionName The name of the function.
* @param jobName The name of the streaming job.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return A function object, containing all information associated with the named function. All functions are contained under a streaming job.
*/
public suspend fun getFunction(
functionName: String,
jobName: String,
resourceGroupName: String,
): GetFunctionResult {
val argument = GetFunctionPlainArgs(
functionName = functionName,
jobName = jobName,
resourceGroupName = resourceGroupName,
)
return getFunctionResultToKotlin(getFunctionPlain(argument.toJava()).await())
}
/**
* @see [getFunction].
* @param argument Builder for [com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetFunctionPlainArgs].
* @return A function object, containing all information associated with the named function. All functions are contained under a streaming job.
*/
public suspend fun getFunction(argument: suspend GetFunctionPlainArgsBuilder.() -> Unit): GetFunctionResult {
val builder = GetFunctionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFunctionResultToKotlin(getFunctionPlain(builtArgument.toJava()).await())
}
/**
* Gets details about the specified input.
* Azure REST API version: 2020-03-01.
* Other available API versions: 2021-10-01-preview.
* @param argument null
* @return An input object, containing all information associated with the named input. All inputs are contained under a streaming job.
*/
public suspend fun getInput(argument: GetInputPlainArgs): GetInputResult =
getInputResultToKotlin(getInputPlain(argument.toJava()).await())
/**
* @see [getInput].
* @param inputName The name of the input.
* @param jobName The name of the streaming job.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An input object, containing all information associated with the named input. All inputs are contained under a streaming job.
*/
public suspend fun getInput(
inputName: String,
jobName: String,
resourceGroupName: String,
): GetInputResult {
val argument = GetInputPlainArgs(
inputName = inputName,
jobName = jobName,
resourceGroupName = resourceGroupName,
)
return getInputResultToKotlin(getInputPlain(argument.toJava()).await())
}
/**
* @see [getInput].
* @param argument Builder for [com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetInputPlainArgs].
* @return An input object, containing all information associated with the named input. All inputs are contained under a streaming job.
*/
public suspend fun getInput(argument: suspend GetInputPlainArgsBuilder.() -> Unit): GetInputResult {
val builder = GetInputPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getInputResultToKotlin(getInputPlain(builtArgument.toJava()).await())
}
/**
* Gets details about the specified output.
* Azure REST API version: 2020-03-01.
* Other available API versions: 2021-10-01-preview.
* @param argument null
* @return An output object, containing all information associated with the named output. All outputs are contained under a streaming job.
*/
public suspend fun getOutput(argument: GetOutputPlainArgs): GetOutputResult =
getOutputResultToKotlin(getOutputPlain(argument.toJava()).await())
/**
* @see [getOutput].
* @param jobName The name of the streaming job.
* @param outputName The name of the output.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An output object, containing all information associated with the named output. All outputs are contained under a streaming job.
*/
public suspend fun getOutput(
jobName: String,
outputName: String,
resourceGroupName: String,
): GetOutputResult {
val argument = GetOutputPlainArgs(
jobName = jobName,
outputName = outputName,
resourceGroupName = resourceGroupName,
)
return getOutputResultToKotlin(getOutputPlain(argument.toJava()).await())
}
/**
* @see [getOutput].
* @param argument Builder for [com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetOutputPlainArgs].
* @return An output object, containing all information associated with the named output. All outputs are contained under a streaming job.
*/
public suspend fun getOutput(argument: suspend GetOutputPlainArgsBuilder.() -> Unit): GetOutputResult {
val builder = GetOutputPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getOutputResultToKotlin(getOutputPlain(builtArgument.toJava()).await())
}
/**
* Gets information about the specified Private Endpoint.
* Azure REST API version: 2020-03-01.
* Other available API versions: 2020-03-01-preview.
* @param argument null
* @return Complete information about the private endpoint.
*/
public suspend fun getPrivateEndpoint(argument: GetPrivateEndpointPlainArgs): GetPrivateEndpointResult =
getPrivateEndpointResultToKotlin(getPrivateEndpointPlain(argument.toJava()).await())
/**
* @see [getPrivateEndpoint].
* @param clusterName The name of the cluster.
* @param privateEndpointName The name of the private endpoint.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return Complete information about the private endpoint.
*/
public suspend fun getPrivateEndpoint(
clusterName: String,
privateEndpointName: String,
resourceGroupName: String,
): GetPrivateEndpointResult {
val argument = GetPrivateEndpointPlainArgs(
clusterName = clusterName,
privateEndpointName = privateEndpointName,
resourceGroupName = resourceGroupName,
)
return getPrivateEndpointResultToKotlin(getPrivateEndpointPlain(argument.toJava()).await())
}
/**
* @see [getPrivateEndpoint].
* @param argument Builder for [com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetPrivateEndpointPlainArgs].
* @return Complete information about the private endpoint.
*/
public suspend fun getPrivateEndpoint(argument: suspend GetPrivateEndpointPlainArgsBuilder.() -> Unit): GetPrivateEndpointResult {
val builder = GetPrivateEndpointPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPrivateEndpointResultToKotlin(getPrivateEndpointPlain(builtArgument.toJava()).await())
}
/**
* Gets details about the specified streaming job.
* Azure REST API version: 2020-03-01.
* Other available API versions: 2017-04-01-preview, 2021-10-01-preview.
* @param argument null
* @return A streaming job object, containing all information associated with the named streaming job.
*/
public suspend fun getStreamingJob(argument: GetStreamingJobPlainArgs): GetStreamingJobResult =
getStreamingJobResultToKotlin(getStreamingJobPlain(argument.toJava()).await())
/**
* @see [getStreamingJob].
* @param expand The $expand OData query parameter. This is a comma-separated list of additional streaming job properties to include in the response, beyond the default set returned when this parameter is absent. The default set is all streaming job properties other than 'inputs', 'transformation', 'outputs', and 'functions'.
* @param jobName The name of the streaming job.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return A streaming job object, containing all information associated with the named streaming job.
*/
public suspend fun getStreamingJob(
expand: String? = null,
jobName: String,
resourceGroupName: String,
): GetStreamingJobResult {
val argument = GetStreamingJobPlainArgs(
expand = expand,
jobName = jobName,
resourceGroupName = resourceGroupName,
)
return getStreamingJobResultToKotlin(getStreamingJobPlain(argument.toJava()).await())
}
/**
* @see [getStreamingJob].
* @param argument Builder for [com.pulumi.azurenative.streamanalytics.kotlin.inputs.GetStreamingJobPlainArgs].
* @return A streaming job object, containing all information associated with the named streaming job.
*/
public suspend fun getStreamingJob(argument: suspend GetStreamingJobPlainArgsBuilder.() -> Unit): GetStreamingJobResult {
val builder = GetStreamingJobPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStreamingJobResultToKotlin(getStreamingJobPlain(builtArgument.toJava()).await())
}
/**
* Lists all of the streaming jobs in the given cluster.
* Azure REST API version: 2020-03-01.
* Other available API versions: 2020-03-01-preview.
* @param argument null
* @return A list of streaming jobs. Populated by a List operation.
*/
public suspend fun listClusterStreamingJobs(argument: ListClusterStreamingJobsPlainArgs): ListClusterStreamingJobsResult =
listClusterStreamingJobsResultToKotlin(listClusterStreamingJobsPlain(argument.toJava()).await())
/**
* @see [listClusterStreamingJobs].
* @param clusterName The name of the cluster.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return A list of streaming jobs. Populated by a List operation.
*/
public suspend fun listClusterStreamingJobs(clusterName: String, resourceGroupName: String): ListClusterStreamingJobsResult {
val argument = ListClusterStreamingJobsPlainArgs(
clusterName = clusterName,
resourceGroupName = resourceGroupName,
)
return listClusterStreamingJobsResultToKotlin(listClusterStreamingJobsPlain(argument.toJava()).await())
}
/**
* @see [listClusterStreamingJobs].
* @param argument Builder for [com.pulumi.azurenative.streamanalytics.kotlin.inputs.ListClusterStreamingJobsPlainArgs].
* @return A list of streaming jobs. Populated by a List operation.
*/
public suspend fun listClusterStreamingJobs(argument: suspend ListClusterStreamingJobsPlainArgsBuilder.() -> Unit): ListClusterStreamingJobsResult {
val builder = ListClusterStreamingJobsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listClusterStreamingJobsResultToKotlin(listClusterStreamingJobsPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy