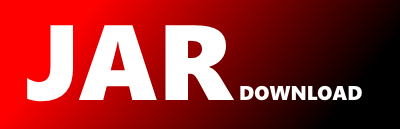
com.pulumi.azurenative.streamanalytics.kotlin.inputs.AzureDataLakeStoreOutputDataSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.streamanalytics.kotlin.inputs
import com.pulumi.azurenative.streamanalytics.inputs.AzureDataLakeStoreOutputDataSourceArgs.builder
import com.pulumi.azurenative.streamanalytics.kotlin.enums.AuthenticationMode
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Describes an Azure Data Lake Store output data source.
* @property accountName The name of the Azure Data Lake Store account. Required on PUT (CreateOrReplace) requests.
* @property authenticationMode Authentication Mode.
* @property dateFormat The date format. Wherever {date} appears in filePathPrefix, the value of this property is used as the date format instead.
* @property filePathPrefix The location of the file to which the output should be written to. Required on PUT (CreateOrReplace) requests.
* @property refreshToken A refresh token that can be used to obtain a valid access token that can then be used to authenticate with the data source. A valid refresh token is currently only obtainable via the Azure Portal. It is recommended to put a dummy string value here when creating the data source and then going to the Azure Portal to authenticate the data source which will update this property with a valid refresh token. Required on PUT (CreateOrReplace) requests.
* @property tenantId The tenant id of the user used to obtain the refresh token. Required on PUT (CreateOrReplace) requests.
* @property timeFormat The time format. Wherever {time} appears in filePathPrefix, the value of this property is used as the time format instead.
* @property tokenUserDisplayName The user display name of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
* @property tokenUserPrincipalName The user principal name (UPN) of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
* @property type Indicates the type of data source output will be written to. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.DataLake/Accounts'.
*/
public data class AzureDataLakeStoreOutputDataSourceArgs(
public val accountName: Output? = null,
public val authenticationMode: Output>? = null,
public val dateFormat: Output? = null,
public val filePathPrefix: Output? = null,
public val refreshToken: Output? = null,
public val tenantId: Output? = null,
public val timeFormat: Output? = null,
public val tokenUserDisplayName: Output? = null,
public val tokenUserPrincipalName: Output? = null,
public val type: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.streamanalytics.inputs.AzureDataLakeStoreOutputDataSourceArgs =
com.pulumi.azurenative.streamanalytics.inputs.AzureDataLakeStoreOutputDataSourceArgs.builder()
.accountName(accountName?.applyValue({ args0 -> args0 }))
.authenticationMode(
authenticationMode?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.dateFormat(dateFormat?.applyValue({ args0 -> args0 }))
.filePathPrefix(filePathPrefix?.applyValue({ args0 -> args0 }))
.refreshToken(refreshToken?.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 }))
.timeFormat(timeFormat?.applyValue({ args0 -> args0 }))
.tokenUserDisplayName(tokenUserDisplayName?.applyValue({ args0 -> args0 }))
.tokenUserPrincipalName(tokenUserPrincipalName?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AzureDataLakeStoreOutputDataSourceArgs].
*/
@PulumiTagMarker
public class AzureDataLakeStoreOutputDataSourceArgsBuilder internal constructor() {
private var accountName: Output? = null
private var authenticationMode: Output>? = null
private var dateFormat: Output? = null
private var filePathPrefix: Output? = null
private var refreshToken: Output? = null
private var tenantId: Output? = null
private var timeFormat: Output? = null
private var tokenUserDisplayName: Output? = null
private var tokenUserPrincipalName: Output? = null
private var type: Output? = null
/**
* @param value The name of the Azure Data Lake Store account. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("vldigcqbenxtleon")
public suspend fun accountName(`value`: Output) {
this.accountName = value
}
/**
* @param value Authentication Mode.
*/
@JvmName("ridxfjvawcigjcqw")
public suspend fun authenticationMode(`value`: Output>) {
this.authenticationMode = value
}
/**
* @param value The date format. Wherever {date} appears in filePathPrefix, the value of this property is used as the date format instead.
*/
@JvmName("whatcqtpqemmfggg")
public suspend fun dateFormat(`value`: Output) {
this.dateFormat = value
}
/**
* @param value The location of the file to which the output should be written to. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("wjlfbcrvoefthiye")
public suspend fun filePathPrefix(`value`: Output) {
this.filePathPrefix = value
}
/**
* @param value A refresh token that can be used to obtain a valid access token that can then be used to authenticate with the data source. A valid refresh token is currently only obtainable via the Azure Portal. It is recommended to put a dummy string value here when creating the data source and then going to the Azure Portal to authenticate the data source which will update this property with a valid refresh token. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("osqptdcwiltevbcb")
public suspend fun refreshToken(`value`: Output) {
this.refreshToken = value
}
/**
* @param value The tenant id of the user used to obtain the refresh token. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("ohbxsagamgfvqhef")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value The time format. Wherever {time} appears in filePathPrefix, the value of this property is used as the time format instead.
*/
@JvmName("xnxhwywakrfasmfe")
public suspend fun timeFormat(`value`: Output) {
this.timeFormat = value
}
/**
* @param value The user display name of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*/
@JvmName("bbnyxtitadnomueh")
public suspend fun tokenUserDisplayName(`value`: Output) {
this.tokenUserDisplayName = value
}
/**
* @param value The user principal name (UPN) of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*/
@JvmName("gsfyhdwwoopukmkq")
public suspend fun tokenUserPrincipalName(`value`: Output) {
this.tokenUserPrincipalName = value
}
/**
* @param value Indicates the type of data source output will be written to. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.DataLake/Accounts'.
*/
@JvmName("emuvhbafygsfrljk")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The name of the Azure Data Lake Store account. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("qiqotrxtktbkcypi")
public suspend fun accountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accountName = mapped
}
/**
* @param value Authentication Mode.
*/
@JvmName("kmsmgvkhopvumngy")
public suspend fun authenticationMode(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authenticationMode = mapped
}
/**
* @param value Authentication Mode.
*/
@JvmName("cnajfescsvdavqil")
public fun authenticationMode(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authenticationMode = mapped
}
/**
* @param value Authentication Mode.
*/
@JvmName("lvjixecsxhvkqfqd")
public fun authenticationMode(`value`: AuthenticationMode) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authenticationMode = mapped
}
/**
* @param value The date format. Wherever {date} appears in filePathPrefix, the value of this property is used as the date format instead.
*/
@JvmName("evgfokwibsfduhln")
public suspend fun dateFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dateFormat = mapped
}
/**
* @param value The location of the file to which the output should be written to. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("rgdwsxblciwymmgb")
public suspend fun filePathPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filePathPrefix = mapped
}
/**
* @param value A refresh token that can be used to obtain a valid access token that can then be used to authenticate with the data source. A valid refresh token is currently only obtainable via the Azure Portal. It is recommended to put a dummy string value here when creating the data source and then going to the Azure Portal to authenticate the data source which will update this property with a valid refresh token. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("ldftbhkwywbystis")
public suspend fun refreshToken(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.refreshToken = mapped
}
/**
* @param value The tenant id of the user used to obtain the refresh token. Required on PUT (CreateOrReplace) requests.
*/
@JvmName("togvngsjxmtmulfd")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
/**
* @param value The time format. Wherever {time} appears in filePathPrefix, the value of this property is used as the time format instead.
*/
@JvmName("ntifnitqolyibsmg")
public suspend fun timeFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeFormat = mapped
}
/**
* @param value The user display name of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*/
@JvmName("baorbhsjhyvapooi")
public suspend fun tokenUserDisplayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tokenUserDisplayName = mapped
}
/**
* @param value The user principal name (UPN) of the user that was used to obtain the refresh token. Use this property to help remember which user was used to obtain the refresh token.
*/
@JvmName("xmjewbdbwyhpyqks")
public suspend fun tokenUserPrincipalName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tokenUserPrincipalName = mapped
}
/**
* @param value Indicates the type of data source output will be written to. Required on PUT (CreateOrReplace) requests.
* Expected value is 'Microsoft.DataLake/Accounts'.
*/
@JvmName("ekicagdohtdjhgmw")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): AzureDataLakeStoreOutputDataSourceArgs =
AzureDataLakeStoreOutputDataSourceArgs(
accountName = accountName,
authenticationMode = authenticationMode,
dateFormat = dateFormat,
filePathPrefix = filePathPrefix,
refreshToken = refreshToken,
tenantId = tenantId,
timeFormat = timeFormat,
tokenUserDisplayName = tokenUserDisplayName,
tokenUserPrincipalName = tokenUserPrincipalName,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy