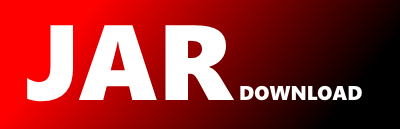
com.pulumi.azurenative.testbase.kotlin.FavoriteProcessArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.testbase.kotlin
import com.pulumi.azurenative.testbase.FavoriteProcessArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A favorite process identifier.
* Azure REST API version: 2022-04-01-preview. Prior API version in Azure Native 1.x: 2022-04-01-preview.
* Other available API versions: 2023-11-01-preview.
* ## Example Usage
* ### FavoriteProcessCreate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var favoriteProcess = new AzureNative.TestBase.FavoriteProcess("favoriteProcess", new()
* {
* ActualProcessName = "testApp&.exe",
* FavoriteProcessResourceName = "testAppProcess",
* PackageName = "contoso-package2",
* ResourceGroupName = "contoso-rg1",
* TestBaseAccountName = "contoso-testBaseAccount1",
* });
* });
* ```
* ```go
* package main
* import (
* testbase "github.com/pulumi/pulumi-azure-native-sdk/testbase/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := testbase.NewFavoriteProcess(ctx, "favoriteProcess", &testbase.FavoriteProcessArgs{
* ActualProcessName: pulumi.String("testApp&.exe"),
* FavoriteProcessResourceName: pulumi.String("testAppProcess"),
* PackageName: pulumi.String("contoso-package2"),
* ResourceGroupName: pulumi.String("contoso-rg1"),
* TestBaseAccountName: pulumi.String("contoso-testBaseAccount1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.testbase.FavoriteProcess;
* import com.pulumi.azurenative.testbase.FavoriteProcessArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var favoriteProcess = new FavoriteProcess("favoriteProcess", FavoriteProcessArgs.builder()
* .actualProcessName("testApp&.exe")
* .favoriteProcessResourceName("testAppProcess")
* .packageName("contoso-package2")
* .resourceGroupName("contoso-rg1")
* .testBaseAccountName("contoso-testBaseAccount1")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:testbase:FavoriteProcess testAppProcess /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.TestBase/testBaseAccounts/{testBaseAccountName}/packages/{packageName}/favoriteProcesses/{favoriteProcessResourceName}
* ```
* @property actualProcessName The actual name of the favorite process. It will be equal to resource name except for the scenario that the process name contains characters that are not allowed in the resource name.
* @property favoriteProcessResourceName The resource name of a favorite process in a package. If the process name contains characters that are not allowed in Azure Resource Name, we use 'actualProcessName' in request body to submit the name.
* @property packageName The resource name of the Test Base Package.
* @property resourceGroupName The name of the resource group that contains the resource.
* @property testBaseAccountName The resource name of the Test Base Account.
*/
public data class FavoriteProcessArgs(
public val actualProcessName: Output? = null,
public val favoriteProcessResourceName: Output? = null,
public val packageName: Output? = null,
public val resourceGroupName: Output? = null,
public val testBaseAccountName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.testbase.FavoriteProcessArgs =
com.pulumi.azurenative.testbase.FavoriteProcessArgs.builder()
.actualProcessName(actualProcessName?.applyValue({ args0 -> args0 }))
.favoriteProcessResourceName(favoriteProcessResourceName?.applyValue({ args0 -> args0 }))
.packageName(packageName?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.testBaseAccountName(testBaseAccountName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FavoriteProcessArgs].
*/
@PulumiTagMarker
public class FavoriteProcessArgsBuilder internal constructor() {
private var actualProcessName: Output? = null
private var favoriteProcessResourceName: Output? = null
private var packageName: Output? = null
private var resourceGroupName: Output? = null
private var testBaseAccountName: Output? = null
/**
* @param value The actual name of the favorite process. It will be equal to resource name except for the scenario that the process name contains characters that are not allowed in the resource name.
*/
@JvmName("wjjwiyeddyolhham")
public suspend fun actualProcessName(`value`: Output) {
this.actualProcessName = value
}
/**
* @param value The resource name of a favorite process in a package. If the process name contains characters that are not allowed in Azure Resource Name, we use 'actualProcessName' in request body to submit the name.
*/
@JvmName("kglyamkxxrirfldi")
public suspend fun favoriteProcessResourceName(`value`: Output) {
this.favoriteProcessResourceName = value
}
/**
* @param value The resource name of the Test Base Package.
*/
@JvmName("bwxelkmiqkssmhrk")
public suspend fun packageName(`value`: Output) {
this.packageName = value
}
/**
* @param value The name of the resource group that contains the resource.
*/
@JvmName("gptrlhwaxxuosnsv")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The resource name of the Test Base Account.
*/
@JvmName("tkgqacfyaslrhtpq")
public suspend fun testBaseAccountName(`value`: Output) {
this.testBaseAccountName = value
}
/**
* @param value The actual name of the favorite process. It will be equal to resource name except for the scenario that the process name contains characters that are not allowed in the resource name.
*/
@JvmName("lmbfdefxdlpciyhd")
public suspend fun actualProcessName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.actualProcessName = mapped
}
/**
* @param value The resource name of a favorite process in a package. If the process name contains characters that are not allowed in Azure Resource Name, we use 'actualProcessName' in request body to submit the name.
*/
@JvmName("yhlrbpyspnfokcbi")
public suspend fun favoriteProcessResourceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.favoriteProcessResourceName = mapped
}
/**
* @param value The resource name of the Test Base Package.
*/
@JvmName("bquvxqegnqwumgmc")
public suspend fun packageName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.packageName = mapped
}
/**
* @param value The name of the resource group that contains the resource.
*/
@JvmName("fvavflmnjdfbcxrk")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The resource name of the Test Base Account.
*/
@JvmName("vnfqvuopkhhnnrro")
public suspend fun testBaseAccountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.testBaseAccountName = mapped
}
internal fun build(): FavoriteProcessArgs = FavoriteProcessArgs(
actualProcessName = actualProcessName,
favoriteProcessResourceName = favoriteProcessResourceName,
packageName = packageName,
resourceGroupName = resourceGroupName,
testBaseAccountName = testBaseAccountName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy