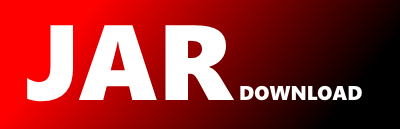
com.pulumi.azurenative.testbase.kotlin.inputs.CommandArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.testbase.kotlin.inputs
import com.pulumi.azurenative.testbase.inputs.CommandArgs.builder
import com.pulumi.azurenative.testbase.kotlin.enums.Action
import com.pulumi.azurenative.testbase.kotlin.enums.ContentType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The command used in the test
* @property action The action of the command.
* @property alwaysRun Specifies whether to run the command even if a previous command is failed.
* @property applyUpdateBefore Specifies whether to apply update before the command.
* @property content The content of the command. The content depends on source type.
* @property contentType The type of command content.
* @property enrollIntuneBefore Specifies whether to enroll Intune before the command.
* @property install1PAppBefore Specifies whether to install first party applications before running the command.
* @property maxRunTime Specifies the max run time of the command.
* @property name The name of the command.
* @property postUpgrade Specifies whether the command is assigned to be executed after in-place upgrade.
* @property preUpgrade Specifies whether the command is assigned to be executed before in-place upgrade.
* @property restartAfter Specifies whether to restart the VM after the command executed.
* @property runAsInteractive Specifies whether to run the command in interactive mode.
* @property runElevated Specifies whether to run the command as administrator.
*/
public data class CommandArgs(
public val action: Output>,
public val alwaysRun: Output? = null,
public val applyUpdateBefore: Output? = null,
public val content: Output,
public val contentType: Output>,
public val enrollIntuneBefore: Output? = null,
public val install1PAppBefore: Output? = null,
public val maxRunTime: Output? = null,
public val name: Output,
public val postUpgrade: Output? = null,
public val preUpgrade: Output? = null,
public val restartAfter: Output? = null,
public val runAsInteractive: Output? = null,
public val runElevated: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.testbase.inputs.CommandArgs =
com.pulumi.azurenative.testbase.inputs.CommandArgs.builder()
.action(
action.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.alwaysRun(alwaysRun?.applyValue({ args0 -> args0 }))
.applyUpdateBefore(applyUpdateBefore?.applyValue({ args0 -> args0 }))
.content(content.applyValue({ args0 -> args0 }))
.contentType(
contentType.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.enrollIntuneBefore(enrollIntuneBefore?.applyValue({ args0 -> args0 }))
.install1PAppBefore(install1PAppBefore?.applyValue({ args0 -> args0 }))
.maxRunTime(maxRunTime?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.postUpgrade(postUpgrade?.applyValue({ args0 -> args0 }))
.preUpgrade(preUpgrade?.applyValue({ args0 -> args0 }))
.restartAfter(restartAfter?.applyValue({ args0 -> args0 }))
.runAsInteractive(runAsInteractive?.applyValue({ args0 -> args0 }))
.runElevated(runElevated?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CommandArgs].
*/
@PulumiTagMarker
public class CommandArgsBuilder internal constructor() {
private var action: Output>? = null
private var alwaysRun: Output? = null
private var applyUpdateBefore: Output? = null
private var content: Output? = null
private var contentType: Output>? = null
private var enrollIntuneBefore: Output? = null
private var install1PAppBefore: Output? = null
private var maxRunTime: Output? = null
private var name: Output? = null
private var postUpgrade: Output? = null
private var preUpgrade: Output? = null
private var restartAfter: Output? = null
private var runAsInteractive: Output? = null
private var runElevated: Output? = null
/**
* @param value The action of the command.
*/
@JvmName("radmveupxkppaqys")
public suspend fun action(`value`: Output>) {
this.action = value
}
/**
* @param value Specifies whether to run the command even if a previous command is failed.
*/
@JvmName("bkulsidoxvtvorib")
public suspend fun alwaysRun(`value`: Output) {
this.alwaysRun = value
}
/**
* @param value Specifies whether to apply update before the command.
*/
@JvmName("thuprutqmcveipdw")
public suspend fun applyUpdateBefore(`value`: Output) {
this.applyUpdateBefore = value
}
/**
* @param value The content of the command. The content depends on source type.
*/
@JvmName("fsjfnxtbwahojfys")
public suspend fun content(`value`: Output) {
this.content = value
}
/**
* @param value The type of command content.
*/
@JvmName("ergimeqslwgixyvm")
public suspend fun contentType(`value`: Output>) {
this.contentType = value
}
/**
* @param value Specifies whether to enroll Intune before the command.
*/
@JvmName("ekanlkdwhojivgjv")
public suspend fun enrollIntuneBefore(`value`: Output) {
this.enrollIntuneBefore = value
}
/**
* @param value Specifies whether to install first party applications before running the command.
*/
@JvmName("gykeuthhbcnugdls")
public suspend fun install1PAppBefore(`value`: Output) {
this.install1PAppBefore = value
}
/**
* @param value Specifies the max run time of the command.
*/
@JvmName("kqclfjewlxjjioti")
public suspend fun maxRunTime(`value`: Output) {
this.maxRunTime = value
}
/**
* @param value The name of the command.
*/
@JvmName("vwvlminwccqtwrdi")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Specifies whether the command is assigned to be executed after in-place upgrade.
*/
@JvmName("syrocdfusmnqvduu")
public suspend fun postUpgrade(`value`: Output) {
this.postUpgrade = value
}
/**
* @param value Specifies whether the command is assigned to be executed before in-place upgrade.
*/
@JvmName("rkwfqpixywbpbwho")
public suspend fun preUpgrade(`value`: Output) {
this.preUpgrade = value
}
/**
* @param value Specifies whether to restart the VM after the command executed.
*/
@JvmName("yakqgqxkgxynwnvp")
public suspend fun restartAfter(`value`: Output) {
this.restartAfter = value
}
/**
* @param value Specifies whether to run the command in interactive mode.
*/
@JvmName("mqjcsyvgblouukpr")
public suspend fun runAsInteractive(`value`: Output) {
this.runAsInteractive = value
}
/**
* @param value Specifies whether to run the command as administrator.
*/
@JvmName("tukvgjvkoxumvoau")
public suspend fun runElevated(`value`: Output) {
this.runElevated = value
}
/**
* @param value The action of the command.
*/
@JvmName("lgolriaidxtgrrek")
public suspend fun action(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value The action of the command.
*/
@JvmName("cjgliybkbfbjbfxk")
public fun action(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value The action of the command.
*/
@JvmName("hwfrnndavgdqulgk")
public fun action(`value`: Action) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value Specifies whether to run the command even if a previous command is failed.
*/
@JvmName("niadagcrmlihkrog")
public suspend fun alwaysRun(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alwaysRun = mapped
}
/**
* @param value Specifies whether to apply update before the command.
*/
@JvmName("mtewwbkjnqksrqqo")
public suspend fun applyUpdateBefore(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.applyUpdateBefore = mapped
}
/**
* @param value The content of the command. The content depends on source type.
*/
@JvmName("cbfdxagdurvejxxd")
public suspend fun content(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.content = mapped
}
/**
* @param value The type of command content.
*/
@JvmName("tadwwkwoyjguselv")
public suspend fun contentType(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.contentType = mapped
}
/**
* @param value The type of command content.
*/
@JvmName("mkcbjuagcdoaxkrf")
public fun contentType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.contentType = mapped
}
/**
* @param value The type of command content.
*/
@JvmName("qnbetvkohdcjaxsv")
public fun contentType(`value`: ContentType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.contentType = mapped
}
/**
* @param value Specifies whether to enroll Intune before the command.
*/
@JvmName("ohrfamfidscjhaol")
public suspend fun enrollIntuneBefore(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enrollIntuneBefore = mapped
}
/**
* @param value Specifies whether to install first party applications before running the command.
*/
@JvmName("fqceigqocaxplyhp")
public suspend fun install1PAppBefore(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.install1PAppBefore = mapped
}
/**
* @param value Specifies the max run time of the command.
*/
@JvmName("onvkuyciguunrgey")
public suspend fun maxRunTime(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRunTime = mapped
}
/**
* @param value The name of the command.
*/
@JvmName("ctxvaadyxqmbfbqe")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Specifies whether the command is assigned to be executed after in-place upgrade.
*/
@JvmName("hgllidlxuahvghsm")
public suspend fun postUpgrade(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postUpgrade = mapped
}
/**
* @param value Specifies whether the command is assigned to be executed before in-place upgrade.
*/
@JvmName("wfgptwmmgsojhtia")
public suspend fun preUpgrade(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preUpgrade = mapped
}
/**
* @param value Specifies whether to restart the VM after the command executed.
*/
@JvmName("ckfjrftubutauhhb")
public suspend fun restartAfter(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.restartAfter = mapped
}
/**
* @param value Specifies whether to run the command in interactive mode.
*/
@JvmName("lypmqfirymmhurwo")
public suspend fun runAsInteractive(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.runAsInteractive = mapped
}
/**
* @param value Specifies whether to run the command as administrator.
*/
@JvmName("uibxxjxqdpacxstu")
public suspend fun runElevated(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.runElevated = mapped
}
internal fun build(): CommandArgs = CommandArgs(
action = action ?: throw PulumiNullFieldException("action"),
alwaysRun = alwaysRun,
applyUpdateBefore = applyUpdateBefore,
content = content ?: throw PulumiNullFieldException("content"),
contentType = contentType ?: throw PulumiNullFieldException("contentType"),
enrollIntuneBefore = enrollIntuneBefore,
install1PAppBefore = install1PAppBefore,
maxRunTime = maxRunTime,
name = name ?: throw PulumiNullFieldException("name"),
postUpgrade = postUpgrade,
preUpgrade = preUpgrade,
restartAfter = restartAfter,
runAsInteractive = runAsInteractive,
runElevated = runElevated,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy