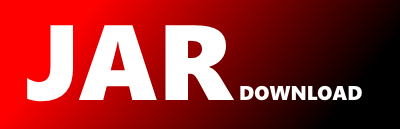
com.pulumi.azurenative.timeseriesinsights.kotlin.EventHubEventSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.timeseriesinsights.kotlin
import com.pulumi.azurenative.timeseriesinsights.EventHubEventSourceArgs.builder
import com.pulumi.azurenative.timeseriesinsights.kotlin.enums.IngressStartAtType
import com.pulumi.azurenative.timeseriesinsights.kotlin.inputs.LocalTimestampArgs
import com.pulumi.azurenative.timeseriesinsights.kotlin.inputs.LocalTimestampArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* An event source that receives its data from an Azure EventHub.
* Azure REST API version: 2020-05-15. Prior API version in Azure Native 1.x: 2020-05-15.
* ## Example Usage
* ### CreateEventHubEventSource
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventHubEventSource = new AzureNative.TimeSeriesInsights.EventHubEventSource("eventHubEventSource", new()
* {
* ConsumerGroupName = "cgn",
* EnvironmentName = "env1",
* EventHubName = "ehn",
* EventSourceName = "es1",
* EventSourceResourceId = "somePathInArm",
* KeyName = "managementKey",
* Kind = "Microsoft.EventHub",
* Location = "West US",
* ResourceGroupName = "rg1",
* ServiceBusNamespace = "sbn",
* SharedAccessKey = "someSecretvalue",
* TimestampPropertyName = "someTimestampProperty",
* Type = AzureNative.TimeSeriesInsights.IngressStartAtType.EarliestAvailable,
* });
* });
* ```
* ```go
* package main
* import (
* timeseriesinsights "github.com/pulumi/pulumi-azure-native-sdk/timeseriesinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := timeseriesinsights.NewEventHubEventSource(ctx, "eventHubEventSource", ×eriesinsights.EventHubEventSourceArgs{
* ConsumerGroupName: pulumi.String("cgn"),
* EnvironmentName: pulumi.String("env1"),
* EventHubName: pulumi.String("ehn"),
* EventSourceName: pulumi.String("es1"),
* EventSourceResourceId: pulumi.String("somePathInArm"),
* KeyName: pulumi.String("managementKey"),
* Kind: pulumi.String("Microsoft.EventHub"),
* Location: pulumi.String("West US"),
* ResourceGroupName: pulumi.String("rg1"),
* ServiceBusNamespace: pulumi.String("sbn"),
* SharedAccessKey: pulumi.String("someSecretvalue"),
* TimestampPropertyName: pulumi.String("someTimestampProperty"),
* Type: pulumi.String(timeseriesinsights.IngressStartAtTypeEarliestAvailable),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.timeseriesinsights.EventHubEventSource;
* import com.pulumi.azurenative.timeseriesinsights.EventHubEventSourceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventHubEventSource = new EventHubEventSource("eventHubEventSource", EventHubEventSourceArgs.builder()
* .consumerGroupName("cgn")
* .environmentName("env1")
* .eventHubName("ehn")
* .eventSourceName("es1")
* .eventSourceResourceId("somePathInArm")
* .keyName("managementKey")
* .kind("Microsoft.EventHub")
* .location("West US")
* .resourceGroupName("rg1")
* .serviceBusNamespace("sbn")
* .sharedAccessKey("someSecretvalue")
* .timestampPropertyName("someTimestampProperty")
* .type("EarliestAvailable")
* .build());
* }
* }
* ```
* ### EventSourcesCreateEventHubWithCustomEnquedTime
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var eventHubEventSource = new AzureNative.TimeSeriesInsights.EventHubEventSource("eventHubEventSource", new()
* {
* ConsumerGroupName = "cgn",
* EnvironmentName = "env1",
* EventHubName = "ehn",
* EventSourceName = "es1",
* EventSourceResourceId = "somePathInArm",
* KeyName = "managementKey",
* Kind = "Microsoft.EventHub",
* Location = "West US",
* ResourceGroupName = "rg1",
* ServiceBusNamespace = "sbn",
* SharedAccessKey = "someSecretvalue",
* Time = "2017-04-01T19:20:33.2288820Z",
* TimestampPropertyName = "someTimestampProperty",
* Type = AzureNative.TimeSeriesInsights.IngressStartAtType.CustomEnqueuedTime,
* });
* });
* ```
* ```go
* package main
* import (
* timeseriesinsights "github.com/pulumi/pulumi-azure-native-sdk/timeseriesinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := timeseriesinsights.NewEventHubEventSource(ctx, "eventHubEventSource", ×eriesinsights.EventHubEventSourceArgs{
* ConsumerGroupName: pulumi.String("cgn"),
* EnvironmentName: pulumi.String("env1"),
* EventHubName: pulumi.String("ehn"),
* EventSourceName: pulumi.String("es1"),
* EventSourceResourceId: pulumi.String("somePathInArm"),
* KeyName: pulumi.String("managementKey"),
* Kind: pulumi.String("Microsoft.EventHub"),
* Location: pulumi.String("West US"),
* ResourceGroupName: pulumi.String("rg1"),
* ServiceBusNamespace: pulumi.String("sbn"),
* SharedAccessKey: pulumi.String("someSecretvalue"),
* Time: pulumi.String("2017-04-01T19:20:33.2288820Z"),
* TimestampPropertyName: pulumi.String("someTimestampProperty"),
* Type: pulumi.String(timeseriesinsights.IngressStartAtTypeCustomEnqueuedTime),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.timeseriesinsights.EventHubEventSource;
* import com.pulumi.azurenative.timeseriesinsights.EventHubEventSourceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var eventHubEventSource = new EventHubEventSource("eventHubEventSource", EventHubEventSourceArgs.builder()
* .consumerGroupName("cgn")
* .environmentName("env1")
* .eventHubName("ehn")
* .eventSourceName("es1")
* .eventSourceResourceId("somePathInArm")
* .keyName("managementKey")
* .kind("Microsoft.EventHub")
* .location("West US")
* .resourceGroupName("rg1")
* .serviceBusNamespace("sbn")
* .sharedAccessKey("someSecretvalue")
* .time("2017-04-01T19:20:33.2288820Z")
* .timestampPropertyName("someTimestampProperty")
* .type("CustomEnqueuedTime")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:timeseriesinsights:EventHubEventSource es1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.TimeSeriesInsights/environments/{environmentName}/eventSources/{eventSourceName}
* ```
* @property consumerGroupName The name of the event hub's consumer group that holds the partitions from which events will be read.
* @property environmentName The name of the Time Series Insights environment associated with the specified resource group.
* @property eventHubName The name of the event hub.
* @property eventSourceName Name of the event source.
* @property eventSourceResourceId The resource id of the event source in Azure Resource Manager.
* @property keyName The name of the SAS key that grants the Time Series Insights service access to the event hub. The shared access policies for this key must grant 'Listen' permissions to the event hub.
* @property kind The kind of the event source.
* Expected value is 'Microsoft.EventHub'.
* @property localTimestamp An object that represents the local timestamp property. It contains the format of local timestamp that needs to be used and the corresponding timezone offset information. If a value isn't specified for localTimestamp, or if null, then the local timestamp will not be ingressed with the events.
* @property location The location of the resource.
* @property resourceGroupName Name of an Azure Resource group.
* @property serviceBusNamespace The name of the service bus that contains the event hub.
* @property sharedAccessKey The value of the shared access key that grants the Time Series Insights service read access to the event hub. This property is not shown in event source responses.
* @property tags Key-value pairs of additional properties for the resource.
* @property time ISO8601 UTC datetime with seconds precision (milliseconds are optional), specifying the date and time that will be the starting point for Events to be consumed.
* @property timestampPropertyName The event property that will be used as the event source's timestamp. If a value isn't specified for timestampPropertyName, or if null or empty-string is specified, the event creation time will be used.
* @property type The type of the ingressStartAt, It can be "EarliestAvailable", "EventSourceCreationTime", "CustomEnqueuedTime".
*/
public data class EventHubEventSourceArgs(
public val consumerGroupName: Output? = null,
public val environmentName: Output? = null,
public val eventHubName: Output? = null,
public val eventSourceName: Output? = null,
public val eventSourceResourceId: Output? = null,
public val keyName: Output? = null,
public val kind: Output? = null,
public val localTimestamp: Output? = null,
public val location: Output? = null,
public val resourceGroupName: Output? = null,
public val serviceBusNamespace: Output? = null,
public val sharedAccessKey: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy