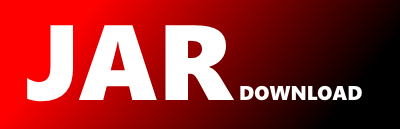
com.pulumi.azurenative.videoanalyzer.kotlin.AccessPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.videoanalyzer.kotlin
import com.pulumi.azurenative.videoanalyzer.AccessPolicyArgs.builder
import com.pulumi.azurenative.videoanalyzer.kotlin.enums.AccessPolicyRole
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.JwtAuthenticationArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.JwtAuthenticationArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Access policies help define the authentication rules, and control access to specific video resources.
* Azure REST API version: 2021-11-01-preview. Prior API version in Azure Native 1.x: 2021-05-01-preview.
* ## Example Usage
* ### Register access policy entity.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var accessPolicy = new AzureNative.VideoAnalyzer.AccessPolicy("accessPolicy", new()
* {
* AccessPolicyName = "accessPolicyName1",
* AccountName = "testaccount2",
* Authentication = new AzureNative.VideoAnalyzer.Inputs.JwtAuthenticationArgs
* {
* Audiences = new[]
* {
* "audience1",
* },
* Claims = new[]
* {
* new AzureNative.VideoAnalyzer.Inputs.TokenClaimArgs
* {
* Name = "claimname1",
* Value = "claimvalue1",
* },
* new AzureNative.VideoAnalyzer.Inputs.TokenClaimArgs
* {
* Name = "claimname2",
* Value = "claimvalue2",
* },
* },
* Issuers = new[]
* {
* "issuer1",
* "issuer2",
* },
* Keys =
* {
* new AzureNative.VideoAnalyzer.Inputs.RsaTokenKeyArgs
* {
* Alg = AzureNative.VideoAnalyzer.AccessPolicyRsaAlgo.RS256,
* E = "ZLFzZTY0IQ==",
* Kid = "123",
* N = "YmFzZTY0IQ==",
* Type = "#Microsoft.VideoAnalyzer.RsaTokenKey",
* },
* new AzureNative.VideoAnalyzer.Inputs.EccTokenKeyArgs
* {
* Alg = AzureNative.VideoAnalyzer.AccessPolicyEccAlgo.ES256,
* Kid = "124",
* Type = "#Microsoft.VideoAnalyzer.EccTokenKey",
* X = "XX==",
* Y = "YY==",
* },
* },
* Type = "#Microsoft.VideoAnalyzer.JwtAuthentication",
* },
* ResourceGroupName = "testrg",
* });
* });
* ```
* ```go
* package main
* import (
* videoanalyzer "github.com/pulumi/pulumi-azure-native-sdk/videoanalyzer/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := videoanalyzer.NewAccessPolicy(ctx, "accessPolicy", &videoanalyzer.AccessPolicyArgs{
* AccessPolicyName: pulumi.String("accessPolicyName1"),
* AccountName: pulumi.String("testaccount2"),
* Authentication: &videoanalyzer.JwtAuthenticationArgs{
* Audiences: pulumi.StringArray{
* pulumi.String("audience1"),
* },
* Claims: videoanalyzer.TokenClaimArray{
* &videoanalyzer.TokenClaimArgs{
* Name: pulumi.String("claimname1"),
* Value: pulumi.String("claimvalue1"),
* },
* &videoanalyzer.TokenClaimArgs{
* Name: pulumi.String("claimname2"),
* Value: pulumi.String("claimvalue2"),
* },
* },
* Issuers: pulumi.StringArray{
* pulumi.String("issuer1"),
* pulumi.String("issuer2"),
* },
* Keys: pulumi.Array{
* videoanalyzer.RsaTokenKey{
* Alg: videoanalyzer.AccessPolicyRsaAlgoRS256,
* E: "ZLFzZTY0IQ==",
* Kid: "123",
* N: "YmFzZTY0IQ==",
* Type: "#Microsoft.VideoAnalyzer.RsaTokenKey",
* },
* videoanalyzer.EccTokenKey{
* Alg: videoanalyzer.AccessPolicyEccAlgoES256,
* Kid: "124",
* Type: "#Microsoft.VideoAnalyzer.EccTokenKey",
* X: "XX==",
* Y: "YY==",
* },
* },
* Type: pulumi.String("#Microsoft.VideoAnalyzer.JwtAuthentication"),
* },
* ResourceGroupName: pulumi.String("testrg"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.videoanalyzer.AccessPolicy;
* import com.pulumi.azurenative.videoanalyzer.AccessPolicyArgs;
* import com.pulumi.azurenative.videoanalyzer.inputs.JwtAuthenticationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var accessPolicy = new AccessPolicy("accessPolicy", AccessPolicyArgs.builder()
* .accessPolicyName("accessPolicyName1")
* .accountName("testaccount2")
* .authentication(JwtAuthenticationArgs.builder()
* .audiences("audience1")
* .claims(
* TokenClaimArgs.builder()
* .name("claimname1")
* .value("claimvalue1")
* .build(),
* TokenClaimArgs.builder()
* .name("claimname2")
* .value("claimvalue2")
* .build())
* .issuers(
* "issuer1",
* "issuer2")
* .keys(
* EccTokenKeyArgs.builder()
* .alg("RS256")
* .e("ZLFzZTY0IQ==")
* .kid("123")
* .n("YmFzZTY0IQ==")
* .type("#Microsoft.VideoAnalyzer.RsaTokenKey")
* .build(),
* EccTokenKeyArgs.builder()
* .alg("ES256")
* .kid("124")
* .type("#Microsoft.VideoAnalyzer.EccTokenKey")
* .x("XX==")
* .y("YY==")
* .build())
* .type("#Microsoft.VideoAnalyzer.JwtAuthentication")
* .build())
* .resourceGroupName("testrg")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:videoanalyzer:AccessPolicy accessPolicyName1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Media/videoAnalyzers/{accountName}/accessPolicies/{accessPolicyName}
* ```
* @property accessPolicyName The Access Policy name.
* @property accountName The Azure Video Analyzer account name.
* @property authentication Authentication method to be used when validating client API access.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property role Defines the access level granted by this policy.
*/
public data class AccessPolicyArgs(
public val accessPolicyName: Output? = null,
public val accountName: Output? = null,
public val authentication: Output? = null,
public val resourceGroupName: Output? = null,
public val role: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.videoanalyzer.AccessPolicyArgs =
com.pulumi.azurenative.videoanalyzer.AccessPolicyArgs.builder()
.accessPolicyName(accessPolicyName?.applyValue({ args0 -> args0 }))
.accountName(accountName?.applyValue({ args0 -> args0 }))
.authentication(authentication?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.role(
role?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [AccessPolicyArgs].
*/
@PulumiTagMarker
public class AccessPolicyArgsBuilder internal constructor() {
private var accessPolicyName: Output? = null
private var accountName: Output? = null
private var authentication: Output? = null
private var resourceGroupName: Output? = null
private var role: Output>? = null
/**
* @param value The Access Policy name.
*/
@JvmName("lfhxvryrjsyymxry")
public suspend fun accessPolicyName(`value`: Output) {
this.accessPolicyName = value
}
/**
* @param value The Azure Video Analyzer account name.
*/
@JvmName("djtinsnkhawmtuyu")
public suspend fun accountName(`value`: Output) {
this.accountName = value
}
/**
* @param value Authentication method to be used when validating client API access.
*/
@JvmName("glofrxkcdtqnemjr")
public suspend fun authentication(`value`: Output) {
this.authentication = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("hsmvkqbubxbskvis")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Defines the access level granted by this policy.
*/
@JvmName("owcpdttdwmihxdea")
public suspend fun role(`value`: Output>) {
this.role = value
}
/**
* @param value The Access Policy name.
*/
@JvmName("nrfddibfstqxpadk")
public suspend fun accessPolicyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessPolicyName = mapped
}
/**
* @param value The Azure Video Analyzer account name.
*/
@JvmName("pggdvjfnphfpprjf")
public suspend fun accountName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accountName = mapped
}
/**
* @param value Authentication method to be used when validating client API access.
*/
@JvmName("cqjmgvpakjdysrtv")
public suspend fun authentication(`value`: JwtAuthenticationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authentication = mapped
}
/**
* @param argument Authentication method to be used when validating client API access.
*/
@JvmName("ofnyrocsfvxacjfm")
public suspend fun authentication(argument: suspend JwtAuthenticationArgsBuilder.() -> Unit) {
val toBeMapped = JwtAuthenticationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.authentication = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("pdjglopmjrevaboh")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Defines the access level granted by this policy.
*/
@JvmName("itexvrmdtefhnbef")
public suspend fun role(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value Defines the access level granted by this policy.
*/
@JvmName("oknceiqtwpcedhwe")
public fun role(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.role = mapped
}
/**
* @param value Defines the access level granted by this policy.
*/
@JvmName("vhporpvawsxaiyvc")
public fun role(`value`: AccessPolicyRole) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.role = mapped
}
internal fun build(): AccessPolicyArgs = AccessPolicyArgs(
accessPolicyName = accessPolicyName,
accountName = accountName,
authentication = authentication,
resourceGroupName = resourceGroupName,
role = role,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy