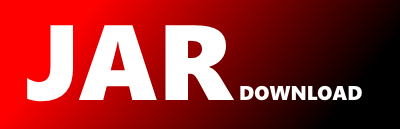
com.pulumi.azurenative.videoanalyzer.kotlin.VideoanalyzerFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.videoanalyzer.kotlin
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getAccessPolicyPlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getEdgeModulePlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getLivePipelinePlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getPipelineJobPlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getPipelineTopologyPlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getPrivateEndpointConnectionPlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getVideoAnalyzerPlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.getVideoPlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.listEdgeModuleProvisioningTokenPlain
import com.pulumi.azurenative.videoanalyzer.VideoanalyzerFunctions.listVideoContentTokenPlain
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetAccessPolicyPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetAccessPolicyPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetEdgeModulePlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetEdgeModulePlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetLivePipelinePlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetLivePipelinePlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPipelineJobPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPipelineJobPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPipelineTopologyPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPipelineTopologyPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPrivateEndpointConnectionPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetVideoAnalyzerPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetVideoAnalyzerPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetVideoPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetVideoPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.ListEdgeModuleProvisioningTokenPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.ListEdgeModuleProvisioningTokenPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.ListVideoContentTokenPlainArgs
import com.pulumi.azurenative.videoanalyzer.kotlin.inputs.ListVideoContentTokenPlainArgsBuilder
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetAccessPolicyResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetEdgeModuleResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetLivePipelineResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetPipelineJobResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetPipelineTopologyResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetPrivateEndpointConnectionResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetVideoAnalyzerResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetVideoResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.ListEdgeModuleProvisioningTokenResult
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.ListVideoContentTokenResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetAccessPolicyResult.Companion.toKotlin as getAccessPolicyResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetEdgeModuleResult.Companion.toKotlin as getEdgeModuleResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetLivePipelineResult.Companion.toKotlin as getLivePipelineResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetPipelineJobResult.Companion.toKotlin as getPipelineJobResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetPipelineTopologyResult.Companion.toKotlin as getPipelineTopologyResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetPrivateEndpointConnectionResult.Companion.toKotlin as getPrivateEndpointConnectionResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetVideoAnalyzerResult.Companion.toKotlin as getVideoAnalyzerResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.GetVideoResult.Companion.toKotlin as getVideoResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.ListEdgeModuleProvisioningTokenResult.Companion.toKotlin as listEdgeModuleProvisioningTokenResultToKotlin
import com.pulumi.azurenative.videoanalyzer.kotlin.outputs.ListVideoContentTokenResult.Companion.toKotlin as listVideoContentTokenResultToKotlin
public object VideoanalyzerFunctions {
/**
* Retrieves an existing access policy resource with the given name.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return Access policies help define the authentication rules, and control access to specific video resources.
*/
public suspend fun getAccessPolicy(argument: GetAccessPolicyPlainArgs): GetAccessPolicyResult =
getAccessPolicyResultToKotlin(getAccessPolicyPlain(argument.toJava()).await())
/**
* @see [getAccessPolicy].
* @param accessPolicyName The Access Policy name.
* @param accountName The Azure Video Analyzer account name.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return Access policies help define the authentication rules, and control access to specific video resources.
*/
public suspend fun getAccessPolicy(
accessPolicyName: String,
accountName: String,
resourceGroupName: String,
): GetAccessPolicyResult {
val argument = GetAccessPolicyPlainArgs(
accessPolicyName = accessPolicyName,
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return getAccessPolicyResultToKotlin(getAccessPolicyPlain(argument.toJava()).await())
}
/**
* @see [getAccessPolicy].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetAccessPolicyPlainArgs].
* @return Access policies help define the authentication rules, and control access to specific video resources.
*/
public suspend fun getAccessPolicy(argument: suspend GetAccessPolicyPlainArgsBuilder.() -> Unit): GetAccessPolicyResult {
val builder = GetAccessPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccessPolicyResultToKotlin(getAccessPolicyPlain(builtArgument.toJava()).await())
}
/**
* Retrieves an existing edge module resource with the given name.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return The representation of an edge module.
*/
public suspend fun getEdgeModule(argument: GetEdgeModulePlainArgs): GetEdgeModuleResult =
getEdgeModuleResultToKotlin(getEdgeModulePlain(argument.toJava()).await())
/**
* @see [getEdgeModule].
* @param accountName The Azure Video Analyzer account name.
* @param edgeModuleName The Edge Module name.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return The representation of an edge module.
*/
public suspend fun getEdgeModule(
accountName: String,
edgeModuleName: String,
resourceGroupName: String,
): GetEdgeModuleResult {
val argument = GetEdgeModulePlainArgs(
accountName = accountName,
edgeModuleName = edgeModuleName,
resourceGroupName = resourceGroupName,
)
return getEdgeModuleResultToKotlin(getEdgeModulePlain(argument.toJava()).await())
}
/**
* @see [getEdgeModule].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetEdgeModulePlainArgs].
* @return The representation of an edge module.
*/
public suspend fun getEdgeModule(argument: suspend GetEdgeModulePlainArgsBuilder.() -> Unit): GetEdgeModuleResult {
val builder = GetEdgeModulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getEdgeModuleResultToKotlin(getEdgeModulePlain(builtArgument.toJava()).await())
}
/**
* Retrieves a specific live pipeline by name. If a live pipeline with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return Live pipeline represents a unique instance of a live topology, used for real-time ingestion, archiving and publishing of content for a unique RTSP camera.
*/
public suspend fun getLivePipeline(argument: GetLivePipelinePlainArgs): GetLivePipelineResult =
getLivePipelineResultToKotlin(getLivePipelinePlain(argument.toJava()).await())
/**
* @see [getLivePipeline].
* @param accountName The Azure Video Analyzer account name.
* @param livePipelineName Live pipeline unique identifier.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return Live pipeline represents a unique instance of a live topology, used for real-time ingestion, archiving and publishing of content for a unique RTSP camera.
*/
public suspend fun getLivePipeline(
accountName: String,
livePipelineName: String,
resourceGroupName: String,
): GetLivePipelineResult {
val argument = GetLivePipelinePlainArgs(
accountName = accountName,
livePipelineName = livePipelineName,
resourceGroupName = resourceGroupName,
)
return getLivePipelineResultToKotlin(getLivePipelinePlain(argument.toJava()).await())
}
/**
* @see [getLivePipeline].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetLivePipelinePlainArgs].
* @return Live pipeline represents a unique instance of a live topology, used for real-time ingestion, archiving and publishing of content for a unique RTSP camera.
*/
public suspend fun getLivePipeline(argument: suspend GetLivePipelinePlainArgsBuilder.() -> Unit): GetLivePipelineResult {
val builder = GetLivePipelinePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLivePipelineResultToKotlin(getLivePipelinePlain(builtArgument.toJava()).await())
}
/**
* Retrieves a specific pipeline job by name. If a pipeline job with that name has been previously created, the call will return the JSON representation of that instance.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return Pipeline job represents a unique instance of a batch topology, used for offline processing of selected portions of archived content.
*/
public suspend fun getPipelineJob(argument: GetPipelineJobPlainArgs): GetPipelineJobResult =
getPipelineJobResultToKotlin(getPipelineJobPlain(argument.toJava()).await())
/**
* @see [getPipelineJob].
* @param accountName The Azure Video Analyzer account name.
* @param pipelineJobName The pipeline job name.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return Pipeline job represents a unique instance of a batch topology, used for offline processing of selected portions of archived content.
*/
public suspend fun getPipelineJob(
accountName: String,
pipelineJobName: String,
resourceGroupName: String,
): GetPipelineJobResult {
val argument = GetPipelineJobPlainArgs(
accountName = accountName,
pipelineJobName = pipelineJobName,
resourceGroupName = resourceGroupName,
)
return getPipelineJobResultToKotlin(getPipelineJobPlain(argument.toJava()).await())
}
/**
* @see [getPipelineJob].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPipelineJobPlainArgs].
* @return Pipeline job represents a unique instance of a batch topology, used for offline processing of selected portions of archived content.
*/
public suspend fun getPipelineJob(argument: suspend GetPipelineJobPlainArgsBuilder.() -> Unit): GetPipelineJobResult {
val builder = GetPipelineJobPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPipelineJobResultToKotlin(getPipelineJobPlain(builtArgument.toJava()).await())
}
/**
* Retrieves a specific pipeline topology by name. If a topology with that name has been previously created, the call will return the JSON representation of that topology.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return Pipeline topology describes the processing steps to be applied when processing content for a particular outcome. The topology should be defined according to the scenario to be achieved and can be reused across many pipeline instances which share the same processing characteristics. For instance, a pipeline topology which captures content from a RTSP camera and archives the content can be reused across many different cameras, as long as the same processing is to be applied across all the cameras. Individual instance properties can be defined through the use of user-defined parameters, which allow for a topology to be parameterized. This allows individual pipelines refer to different values, such as individual cameras' RTSP endpoints and credentials. Overall a topology is composed of the following:
* - Parameters: list of user defined parameters that can be references across the topology nodes.
* - Sources: list of one or more data sources nodes such as an RTSP source which allows for content to be ingested from cameras.
* - Processors: list of nodes which perform data analysis or transformations.
* - Sinks: list of one or more data sinks which allow for data to be stored or exported to other destinations.
*/
public suspend fun getPipelineTopology(argument: GetPipelineTopologyPlainArgs): GetPipelineTopologyResult =
getPipelineTopologyResultToKotlin(getPipelineTopologyPlain(argument.toJava()).await())
/**
* @see [getPipelineTopology].
* @param accountName The Azure Video Analyzer account name.
* @param pipelineTopologyName Pipeline topology unique identifier.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return Pipeline topology describes the processing steps to be applied when processing content for a particular outcome. The topology should be defined according to the scenario to be achieved and can be reused across many pipeline instances which share the same processing characteristics. For instance, a pipeline topology which captures content from a RTSP camera and archives the content can be reused across many different cameras, as long as the same processing is to be applied across all the cameras. Individual instance properties can be defined through the use of user-defined parameters, which allow for a topology to be parameterized. This allows individual pipelines refer to different values, such as individual cameras' RTSP endpoints and credentials. Overall a topology is composed of the following:
* - Parameters: list of user defined parameters that can be references across the topology nodes.
* - Sources: list of one or more data sources nodes such as an RTSP source which allows for content to be ingested from cameras.
* - Processors: list of nodes which perform data analysis or transformations.
* - Sinks: list of one or more data sinks which allow for data to be stored or exported to other destinations.
*/
public suspend fun getPipelineTopology(
accountName: String,
pipelineTopologyName: String,
resourceGroupName: String,
): GetPipelineTopologyResult {
val argument = GetPipelineTopologyPlainArgs(
accountName = accountName,
pipelineTopologyName = pipelineTopologyName,
resourceGroupName = resourceGroupName,
)
return getPipelineTopologyResultToKotlin(getPipelineTopologyPlain(argument.toJava()).await())
}
/**
* @see [getPipelineTopology].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPipelineTopologyPlainArgs].
* @return Pipeline topology describes the processing steps to be applied when processing content for a particular outcome. The topology should be defined according to the scenario to be achieved and can be reused across many pipeline instances which share the same processing characteristics. For instance, a pipeline topology which captures content from a RTSP camera and archives the content can be reused across many different cameras, as long as the same processing is to be applied across all the cameras. Individual instance properties can be defined through the use of user-defined parameters, which allow for a topology to be parameterized. This allows individual pipelines refer to different values, such as individual cameras' RTSP endpoints and credentials. Overall a topology is composed of the following:
* - Parameters: list of user defined parameters that can be references across the topology nodes.
* - Sources: list of one or more data sources nodes such as an RTSP source which allows for content to be ingested from cameras.
* - Processors: list of nodes which perform data analysis or transformations.
* - Sinks: list of one or more data sinks which allow for data to be stored or exported to other destinations.
*/
public suspend fun getPipelineTopology(argument: suspend GetPipelineTopologyPlainArgsBuilder.() -> Unit): GetPipelineTopologyResult {
val builder = GetPipelineTopologyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPipelineTopologyResultToKotlin(getPipelineTopologyPlain(builtArgument.toJava()).await())
}
/**
* Get private endpoint connection under video analyzer account.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return The Private Endpoint Connection resource.
*/
public suspend fun getPrivateEndpointConnection(argument: GetPrivateEndpointConnectionPlainArgs): GetPrivateEndpointConnectionResult =
getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
/**
* @see [getPrivateEndpointConnection].
* @param accountName The Video Analyzer account name.
* @param name Private endpoint connection name.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return The Private Endpoint Connection resource.
*/
public suspend fun getPrivateEndpointConnection(
accountName: String,
name: String,
resourceGroupName: String,
): GetPrivateEndpointConnectionResult {
val argument = GetPrivateEndpointConnectionPlainArgs(
accountName = accountName,
name = name,
resourceGroupName = resourceGroupName,
)
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(argument.toJava()).await())
}
/**
* @see [getPrivateEndpointConnection].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetPrivateEndpointConnectionPlainArgs].
* @return The Private Endpoint Connection resource.
*/
public suspend fun getPrivateEndpointConnection(argument: suspend GetPrivateEndpointConnectionPlainArgsBuilder.() -> Unit): GetPrivateEndpointConnectionResult {
val builder = GetPrivateEndpointConnectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPrivateEndpointConnectionResultToKotlin(getPrivateEndpointConnectionPlain(builtArgument.toJava()).await())
}
/**
* Retrieves an existing video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
* Other available API versions: 2021-05-01-preview.
* @param argument null
* @return Represents a video resource within Azure Video Analyzer. Videos can be ingested from RTSP cameras through live pipelines or can be created by exporting sequences from existing captured video through a pipeline job. Videos ingested through live pipelines can be streamed through Azure Video Analyzer Player Widget or compatible players. Exported videos can be downloaded as MP4 files.
*/
public suspend fun getVideo(argument: GetVideoPlainArgs): GetVideoResult =
getVideoResultToKotlin(getVideoPlain(argument.toJava()).await())
/**
* @see [getVideo].
* @param accountName The Azure Video Analyzer account name.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param videoName The Video name.
* @return Represents a video resource within Azure Video Analyzer. Videos can be ingested from RTSP cameras through live pipelines or can be created by exporting sequences from existing captured video through a pipeline job. Videos ingested through live pipelines can be streamed through Azure Video Analyzer Player Widget or compatible players. Exported videos can be downloaded as MP4 files.
*/
public suspend fun getVideo(
accountName: String,
resourceGroupName: String,
videoName: String,
): GetVideoResult {
val argument = GetVideoPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
videoName = videoName,
)
return getVideoResultToKotlin(getVideoPlain(argument.toJava()).await())
}
/**
* @see [getVideo].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetVideoPlainArgs].
* @return Represents a video resource within Azure Video Analyzer. Videos can be ingested from RTSP cameras through live pipelines or can be created by exporting sequences from existing captured video through a pipeline job. Videos ingested through live pipelines can be streamed through Azure Video Analyzer Player Widget or compatible players. Exported videos can be downloaded as MP4 files.
*/
public suspend fun getVideo(argument: suspend GetVideoPlainArgsBuilder.() -> Unit): GetVideoResult {
val builder = GetVideoPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVideoResultToKotlin(getVideoPlain(builtArgument.toJava()).await())
}
/**
* Get the details of the specified Video Analyzer account
* Azure REST API version: 2021-11-01-preview.
* Other available API versions: 2021-05-01-preview.
* @param argument null
* @return The Video Analyzer account.
*/
public suspend fun getVideoAnalyzer(argument: GetVideoAnalyzerPlainArgs): GetVideoAnalyzerResult =
getVideoAnalyzerResultToKotlin(getVideoAnalyzerPlain(argument.toJava()).await())
/**
* @see [getVideoAnalyzer].
* @param accountName The Video Analyzer account name.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return The Video Analyzer account.
*/
public suspend fun getVideoAnalyzer(accountName: String, resourceGroupName: String): GetVideoAnalyzerResult {
val argument = GetVideoAnalyzerPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return getVideoAnalyzerResultToKotlin(getVideoAnalyzerPlain(argument.toJava()).await())
}
/**
* @see [getVideoAnalyzer].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.GetVideoAnalyzerPlainArgs].
* @return The Video Analyzer account.
*/
public suspend fun getVideoAnalyzer(argument: suspend GetVideoAnalyzerPlainArgsBuilder.() -> Unit): GetVideoAnalyzerResult {
val builder = GetVideoAnalyzerPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVideoAnalyzerResultToKotlin(getVideoAnalyzerPlain(builtArgument.toJava()).await())
}
/**
* Creates a new provisioning token. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return Provisioning token properties. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
*/
public suspend fun listEdgeModuleProvisioningToken(argument: ListEdgeModuleProvisioningTokenPlainArgs): ListEdgeModuleProvisioningTokenResult =
listEdgeModuleProvisioningTokenResultToKotlin(listEdgeModuleProvisioningTokenPlain(argument.toJava()).await())
/**
* @see [listEdgeModuleProvisioningToken].
* @param accountName The Azure Video Analyzer account name.
* @param edgeModuleName The Edge Module name.
* @param expirationDate The desired expiration date of the registration token. The Azure Video Analyzer IoT edge module must be initialized and connected to the Internet prior to the token expiration date.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return Provisioning token properties. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
*/
public suspend fun listEdgeModuleProvisioningToken(
accountName: String,
edgeModuleName: String,
expirationDate: String,
resourceGroupName: String,
): ListEdgeModuleProvisioningTokenResult {
val argument = ListEdgeModuleProvisioningTokenPlainArgs(
accountName = accountName,
edgeModuleName = edgeModuleName,
expirationDate = expirationDate,
resourceGroupName = resourceGroupName,
)
return listEdgeModuleProvisioningTokenResultToKotlin(listEdgeModuleProvisioningTokenPlain(argument.toJava()).await())
}
/**
* @see [listEdgeModuleProvisioningToken].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.ListEdgeModuleProvisioningTokenPlainArgs].
* @return Provisioning token properties. A provisioning token allows for a single instance of Azure Video analyzer IoT edge module to be initialized and authorized to the cloud account. The provisioning token itself is short lived and it is only used for the initial handshake between IoT edge module and the cloud. After the initial handshake, the IoT edge module will agree on a set of authentication keys which will be auto-rotated as long as the module is able to periodically connect to the cloud. A new provisioning token can be generated for the same IoT edge module in case the module state lost or reset.
*/
public suspend fun listEdgeModuleProvisioningToken(argument: suspend ListEdgeModuleProvisioningTokenPlainArgsBuilder.() -> Unit): ListEdgeModuleProvisioningTokenResult {
val builder = ListEdgeModuleProvisioningTokenPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listEdgeModuleProvisioningTokenResultToKotlin(listEdgeModuleProvisioningTokenPlain(builtArgument.toJava()).await())
}
/**
* Generates a streaming token which can be used for accessing content from video content URLs, for a video resource with the given name.
* Azure REST API version: 2021-11-01-preview.
* @param argument null
* @return "Video content token grants access to the video content URLs."
*/
public suspend fun listVideoContentToken(argument: ListVideoContentTokenPlainArgs): ListVideoContentTokenResult =
listVideoContentTokenResultToKotlin(listVideoContentTokenPlain(argument.toJava()).await())
/**
* @see [listVideoContentToken].
* @param accountName The Azure Video Analyzer account name.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param videoName The Video name.
* @return "Video content token grants access to the video content URLs."
*/
public suspend fun listVideoContentToken(
accountName: String,
resourceGroupName: String,
videoName: String,
): ListVideoContentTokenResult {
val argument = ListVideoContentTokenPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
videoName = videoName,
)
return listVideoContentTokenResultToKotlin(listVideoContentTokenPlain(argument.toJava()).await())
}
/**
* @see [listVideoContentToken].
* @param argument Builder for [com.pulumi.azurenative.videoanalyzer.kotlin.inputs.ListVideoContentTokenPlainArgs].
* @return "Video content token grants access to the video content URLs."
*/
public suspend fun listVideoContentToken(argument: suspend ListVideoContentTokenPlainArgsBuilder.() -> Unit): ListVideoContentTokenResult {
val builder = ListVideoContentTokenPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listVideoContentTokenResultToKotlin(listVideoContentTokenPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy