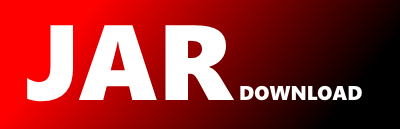
com.pulumi.azurenative.videoanalyzer.kotlin.inputs.RtspSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.videoanalyzer.kotlin.inputs
import com.pulumi.azurenative.videoanalyzer.inputs.RtspSourceArgs.builder
import com.pulumi.azurenative.videoanalyzer.kotlin.enums.RtspTransport
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* RTSP source allows for media from an RTSP camera or generic RTSP server to be ingested into a pipeline.
* @property endpoint RTSP endpoint information for Video Analyzer to connect to. This contains the required information for Video Analyzer to connect to RTSP cameras and/or generic RTSP servers.
* @property name Node name. Must be unique within the topology.
* @property transport Network transport utilized by the RTSP and RTP exchange: TCP or HTTP. When using TCP, the RTP packets are interleaved on the TCP RTSP connection. When using HTTP, the RTSP messages are exchanged through long lived HTTP connections, and the RTP packages are interleaved in the HTTP connections alongside the RTSP messages.
* @property type The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.RtspSource'.
*/
public data class RtspSourceArgs(
public val endpoint: Output>,
public val name: Output,
public val transport: Output>? = null,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.videoanalyzer.inputs.RtspSourceArgs =
com.pulumi.azurenative.videoanalyzer.inputs.RtspSourceArgs.builder()
.endpoint(
endpoint.applyValue({ args0 ->
args0.transform({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.name(name.applyValue({ args0 -> args0 }))
.transport(
transport?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RtspSourceArgs].
*/
@PulumiTagMarker
public class RtspSourceArgsBuilder internal constructor() {
private var endpoint: Output>? = null
private var name: Output? = null
private var transport: Output>? = null
private var type: Output? = null
/**
* @param value RTSP endpoint information for Video Analyzer to connect to. This contains the required information for Video Analyzer to connect to RTSP cameras and/or generic RTSP servers.
*/
@JvmName("cesailtrkyychdfn")
public suspend fun endpoint(`value`: Output>) {
this.endpoint = value
}
/**
* @param value Node name. Must be unique within the topology.
*/
@JvmName("slduonwkrreynbco")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Network transport utilized by the RTSP and RTP exchange: TCP or HTTP. When using TCP, the RTP packets are interleaved on the TCP RTSP connection. When using HTTP, the RTSP messages are exchanged through long lived HTTP connections, and the RTP packages are interleaved in the HTTP connections alongside the RTSP messages.
*/
@JvmName("dbvkhyuvpsqafygn")
public suspend fun transport(`value`: Output>) {
this.transport = value
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.RtspSource'.
*/
@JvmName("akpsmdbcaufibmif")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value RTSP endpoint information for Video Analyzer to connect to. This contains the required information for Video Analyzer to connect to RTSP cameras and/or generic RTSP servers.
*/
@JvmName("tqrylrsckyxxqtwl")
public suspend fun endpoint(`value`: Either) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value RTSP endpoint information for Video Analyzer to connect to. This contains the required information for Video Analyzer to connect to RTSP cameras and/or generic RTSP servers.
*/
@JvmName("fxvwnxwishhvsuyk")
public fun endpoint(`value`: TlsEndpointArgs) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value RTSP endpoint information for Video Analyzer to connect to. This contains the required information for Video Analyzer to connect to RTSP cameras and/or generic RTSP servers.
*/
@JvmName("dsxlgtbrjvvfekxb")
public fun endpoint(`value`: UnsecuredEndpointArgs) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value Node name. Must be unique within the topology.
*/
@JvmName("otepvlwyfhqbqvst")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Network transport utilized by the RTSP and RTP exchange: TCP or HTTP. When using TCP, the RTP packets are interleaved on the TCP RTSP connection. When using HTTP, the RTSP messages are exchanged through long lived HTTP connections, and the RTP packages are interleaved in the HTTP connections alongside the RTSP messages.
*/
@JvmName("matrnuhklqpeniqr")
public suspend fun transport(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.transport = mapped
}
/**
* @param value Network transport utilized by the RTSP and RTP exchange: TCP or HTTP. When using TCP, the RTP packets are interleaved on the TCP RTSP connection. When using HTTP, the RTSP messages are exchanged through long lived HTTP connections, and the RTP packages are interleaved in the HTTP connections alongside the RTSP messages.
*/
@JvmName("ltuxpchbgvxlwpdk")
public fun transport(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.transport = mapped
}
/**
* @param value Network transport utilized by the RTSP and RTP exchange: TCP or HTTP. When using TCP, the RTP packets are interleaved on the TCP RTSP connection. When using HTTP, the RTSP messages are exchanged through long lived HTTP connections, and the RTP packages are interleaved in the HTTP connections alongside the RTSP messages.
*/
@JvmName("helsmdcdlkijfoyd")
public fun transport(`value`: RtspTransport) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.transport = mapped
}
/**
* @param value The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.RtspSource'.
*/
@JvmName("jslukemsrgnyrlrh")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): RtspSourceArgs = RtspSourceArgs(
endpoint = endpoint ?: throw PulumiNullFieldException("endpoint"),
name = name ?: throw PulumiNullFieldException("name"),
transport = transport,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy