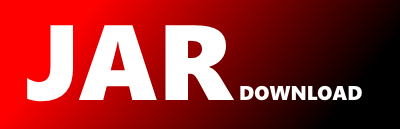
com.pulumi.azurenative.voiceservices.kotlin.VoiceservicesFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.voiceservices.kotlin
import com.pulumi.azurenative.voiceservices.VoiceservicesFunctions.getCommunicationsGatewayPlain
import com.pulumi.azurenative.voiceservices.VoiceservicesFunctions.getContactPlain
import com.pulumi.azurenative.voiceservices.VoiceservicesFunctions.getTestLinePlain
import com.pulumi.azurenative.voiceservices.kotlin.inputs.GetCommunicationsGatewayPlainArgs
import com.pulumi.azurenative.voiceservices.kotlin.inputs.GetCommunicationsGatewayPlainArgsBuilder
import com.pulumi.azurenative.voiceservices.kotlin.inputs.GetContactPlainArgs
import com.pulumi.azurenative.voiceservices.kotlin.inputs.GetContactPlainArgsBuilder
import com.pulumi.azurenative.voiceservices.kotlin.inputs.GetTestLinePlainArgs
import com.pulumi.azurenative.voiceservices.kotlin.inputs.GetTestLinePlainArgsBuilder
import com.pulumi.azurenative.voiceservices.kotlin.outputs.GetCommunicationsGatewayResult
import com.pulumi.azurenative.voiceservices.kotlin.outputs.GetContactResult
import com.pulumi.azurenative.voiceservices.kotlin.outputs.GetTestLineResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.voiceservices.kotlin.outputs.GetCommunicationsGatewayResult.Companion.toKotlin as getCommunicationsGatewayResultToKotlin
import com.pulumi.azurenative.voiceservices.kotlin.outputs.GetContactResult.Companion.toKotlin as getContactResultToKotlin
import com.pulumi.azurenative.voiceservices.kotlin.outputs.GetTestLineResult.Companion.toKotlin as getTestLineResultToKotlin
public object VoiceservicesFunctions {
/**
* Get a CommunicationsGateway
* Azure REST API version: 2023-04-03.
* Other available API versions: 2023-09-01.
* @param argument null
* @return A CommunicationsGateway resource
*/
public suspend fun getCommunicationsGateway(argument: GetCommunicationsGatewayPlainArgs): GetCommunicationsGatewayResult =
getCommunicationsGatewayResultToKotlin(getCommunicationsGatewayPlain(argument.toJava()).await())
/**
* @see [getCommunicationsGateway].
* @param communicationsGatewayName Unique identifier for this deployment
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return A CommunicationsGateway resource
*/
public suspend fun getCommunicationsGateway(
communicationsGatewayName: String,
resourceGroupName: String,
): GetCommunicationsGatewayResult {
val argument = GetCommunicationsGatewayPlainArgs(
communicationsGatewayName = communicationsGatewayName,
resourceGroupName = resourceGroupName,
)
return getCommunicationsGatewayResultToKotlin(getCommunicationsGatewayPlain(argument.toJava()).await())
}
/**
* @see [getCommunicationsGateway].
* @param argument Builder for [com.pulumi.azurenative.voiceservices.kotlin.inputs.GetCommunicationsGatewayPlainArgs].
* @return A CommunicationsGateway resource
*/
public suspend fun getCommunicationsGateway(argument: suspend GetCommunicationsGatewayPlainArgsBuilder.() -> Unit): GetCommunicationsGatewayResult {
val builder = GetCommunicationsGatewayPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCommunicationsGatewayResultToKotlin(getCommunicationsGatewayPlain(builtArgument.toJava()).await())
}
/**
* Get a Contact
* Azure REST API version: 2022-12-01-preview.
* @param argument null
* @return A Contact resource
*/
public suspend fun getContact(argument: GetContactPlainArgs): GetContactResult =
getContactResultToKotlin(getContactPlain(argument.toJava()).await())
/**
* @see [getContact].
* @param communicationsGatewayName Unique identifier for this deployment
* @param contactName Unique identifier for this contact
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return A Contact resource
*/
public suspend fun getContact(
communicationsGatewayName: String,
contactName: String,
resourceGroupName: String,
): GetContactResult {
val argument = GetContactPlainArgs(
communicationsGatewayName = communicationsGatewayName,
contactName = contactName,
resourceGroupName = resourceGroupName,
)
return getContactResultToKotlin(getContactPlain(argument.toJava()).await())
}
/**
* @see [getContact].
* @param argument Builder for [com.pulumi.azurenative.voiceservices.kotlin.inputs.GetContactPlainArgs].
* @return A Contact resource
*/
public suspend fun getContact(argument: suspend GetContactPlainArgsBuilder.() -> Unit): GetContactResult {
val builder = GetContactPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getContactResultToKotlin(getContactPlain(builtArgument.toJava()).await())
}
/**
* Get a TestLine
* Azure REST API version: 2023-04-03.
* Other available API versions: 2022-12-01-preview, 2023-09-01.
* @param argument null
* @return A TestLine resource
*/
public suspend fun getTestLine(argument: GetTestLinePlainArgs): GetTestLineResult =
getTestLineResultToKotlin(getTestLinePlain(argument.toJava()).await())
/**
* @see [getTestLine].
* @param communicationsGatewayName Unique identifier for this deployment
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param testLineName Unique identifier for this test line
* @return A TestLine resource
*/
public suspend fun getTestLine(
communicationsGatewayName: String,
resourceGroupName: String,
testLineName: String,
): GetTestLineResult {
val argument = GetTestLinePlainArgs(
communicationsGatewayName = communicationsGatewayName,
resourceGroupName = resourceGroupName,
testLineName = testLineName,
)
return getTestLineResultToKotlin(getTestLinePlain(argument.toJava()).await())
}
/**
* @see [getTestLine].
* @param argument Builder for [com.pulumi.azurenative.voiceservices.kotlin.inputs.GetTestLinePlainArgs].
* @return A TestLine resource
*/
public suspend fun getTestLine(argument: suspend GetTestLinePlainArgsBuilder.() -> Unit): GetTestLineResult {
val builder = GetTestLinePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTestLineResultToKotlin(getTestLinePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy