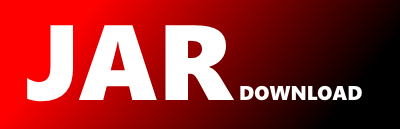
com.pulumi.azurenative.web.kotlin.KubeEnvironment.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.web.kotlin
import com.pulumi.azurenative.web.kotlin.outputs.AppLogsConfigurationResponse
import com.pulumi.azurenative.web.kotlin.outputs.ArcConfigurationResponse
import com.pulumi.azurenative.web.kotlin.outputs.ContainerAppsConfigurationResponse
import com.pulumi.azurenative.web.kotlin.outputs.ExtendedLocationResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.azurenative.web.kotlin.outputs.AppLogsConfigurationResponse.Companion.toKotlin as appLogsConfigurationResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.ArcConfigurationResponse.Companion.toKotlin as arcConfigurationResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.ContainerAppsConfigurationResponse.Companion.toKotlin as containerAppsConfigurationResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.ExtendedLocationResponse.Companion.toKotlin as extendedLocationResponseToKotlin
/**
* Builder for [KubeEnvironment].
*/
@PulumiTagMarker
public class KubeEnvironmentResourceBuilder internal constructor() {
public var name: String? = null
public var args: KubeEnvironmentArgs = KubeEnvironmentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend KubeEnvironmentArgsBuilder.() -> Unit) {
val builder = KubeEnvironmentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): KubeEnvironment {
val builtJavaResource = com.pulumi.azurenative.web.KubeEnvironment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return KubeEnvironment(builtJavaResource)
}
}
/**
* A Kubernetes cluster specialized for web workloads by Azure App Service
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2021-01-01.
* Other available API versions: 2023-01-01, 2023-12-01.
* ## Example Usage
* ### Create kube environments
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var kubeEnvironment = new AzureNative.Web.KubeEnvironment("kubeEnvironment", new()
* {
* Location = "East US",
* Name = "testkubeenv",
* ResourceGroupName = "examplerg",
* StaticIp = "1.2.3.4",
* });
* });
* ```
* ```go
* package main
* import (
* web "github.com/pulumi/pulumi-azure-native-sdk/web/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := web.NewKubeEnvironment(ctx, "kubeEnvironment", &web.KubeEnvironmentArgs{
* Location: pulumi.String("East US"),
* Name: pulumi.String("testkubeenv"),
* ResourceGroupName: pulumi.String("examplerg"),
* StaticIp: pulumi.String("1.2.3.4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.web.KubeEnvironment;
* import com.pulumi.azurenative.web.KubeEnvironmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var kubeEnvironment = new KubeEnvironment("kubeEnvironment", KubeEnvironmentArgs.builder()
* .location("East US")
* .name("testkubeenv")
* .resourceGroupName("examplerg")
* .staticIp("1.2.3.4")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:web:KubeEnvironment testkubeenv /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Web/kubeEnvironments/{name}
* ```
*/
public class KubeEnvironment internal constructor(
override val javaResource: com.pulumi.azurenative.web.KubeEnvironment,
) : KotlinCustomResource(javaResource, KubeEnvironmentMapper) {
public val aksResourceID: Output?
get() = javaResource.aksResourceID().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Cluster configuration which enables the log daemon to export
* app logs to a destination. Currently only "log-analytics" is
* supported
*/
public val appLogsConfiguration: Output?
get() = javaResource.appLogsConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> appLogsConfigurationResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Cluster configuration which determines the ARC cluster
* components types. Eg: Choosing between BuildService kind,
* FrontEnd Service ArtifactsStorageType etc.
*/
public val arcConfiguration: Output?
get() = javaResource.arcConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> arcConfigurationResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Cluster configuration for Container Apps Environments to configure Dapr Instrumentation Key and VNET Configuration
*/
public val containerAppsConfiguration: Output?
get() = javaResource.containerAppsConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> containerAppsConfigurationResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Default Domain Name for the cluster
*/
public val defaultDomain: Output
get() = javaResource.defaultDomain().applyValue({ args0 -> args0 })
/**
* Any errors that occurred during deployment or deployment validation
*/
public val deploymentErrors: Output
get() = javaResource.deploymentErrors().applyValue({ args0 -> args0 })
/**
* Type of Kubernetes Environment. Only supported for Container App Environments with value as Managed
*/
public val environmentType: Output?
get() = javaResource.environmentType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Extended Location.
*/
public val extendedLocation: Output?
get() = javaResource.extendedLocation().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> extendedLocationResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Only visible within Vnet/Subnet
*/
public val internalLoadBalancerEnabled: Output?
get() = javaResource.internalLoadBalancerEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Kind of resource.
*/
public val kind: Output?
get() = javaResource.kind().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Resource Location.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Resource Name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Provisioning state of the Kubernetes Environment.
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* Static IP of the KubeEnvironment
*/
public val staticIp: Output?
get() = javaResource.staticIp().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy