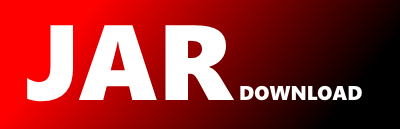
com.pulumi.azurenative.web.kotlin.WebApp.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.web.kotlin
import com.pulumi.azurenative.web.kotlin.outputs.ExtendedLocationResponse
import com.pulumi.azurenative.web.kotlin.outputs.HostNameSslStateResponse
import com.pulumi.azurenative.web.kotlin.outputs.HostingEnvironmentProfileResponse
import com.pulumi.azurenative.web.kotlin.outputs.ManagedServiceIdentityResponse
import com.pulumi.azurenative.web.kotlin.outputs.SiteConfigResponse
import com.pulumi.azurenative.web.kotlin.outputs.SlotSwapStatusResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.azurenative.web.kotlin.outputs.ExtendedLocationResponse.Companion.toKotlin as extendedLocationResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.HostNameSslStateResponse.Companion.toKotlin as hostNameSslStateResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.HostingEnvironmentProfileResponse.Companion.toKotlin as hostingEnvironmentProfileResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.ManagedServiceIdentityResponse.Companion.toKotlin as managedServiceIdentityResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.SiteConfigResponse.Companion.toKotlin as siteConfigResponseToKotlin
import com.pulumi.azurenative.web.kotlin.outputs.SlotSwapStatusResponse.Companion.toKotlin as slotSwapStatusResponseToKotlin
/**
* Builder for [WebApp].
*/
@PulumiTagMarker
public class WebAppResourceBuilder internal constructor() {
public var name: String? = null
public var args: WebAppArgs = WebAppArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend WebAppArgsBuilder.() -> Unit) {
val builder = WebAppArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): WebApp {
val builtJavaResource = com.pulumi.azurenative.web.WebApp(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return WebApp(builtJavaResource)
}
}
/**
* A web app, a mobile app backend, or an API app.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2020-12-01.
* Other available API versions: 2016-08-01, 2018-11-01, 2020-10-01, 2023-01-01, 2023-12-01.
* ## Example Usage
* ### Clone web app
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var webApp = new AzureNative.Web.WebApp("webApp", new()
* {
* CloningInfo = new AzureNative.Web.Inputs.CloningInfoArgs
* {
* AppSettingsOverrides =
* {
* { "Setting1", "NewValue1" },
* { "Setting3", "NewValue5" },
* },
* CloneCustomHostNames = true,
* CloneSourceControl = true,
* ConfigureLoadBalancing = false,
* HostingEnvironment = "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg456/providers/Microsoft.Web/hostingenvironments/aseforsites",
* Overwrite = false,
* SourceWebAppId = "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg456/providers/Microsoft.Web/sites/srcsiteg478",
* SourceWebAppLocation = "West Europe",
* },
* Kind = "app",
* Location = "East US",
* Name = "sitef6141",
* ResourceGroupName = "testrg123",
* });
* });
* ```
* ```go
* package main
* import (
* web "github.com/pulumi/pulumi-azure-native-sdk/web/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := web.NewWebApp(ctx, "webApp", &web.WebAppArgs{
* CloningInfo: &web.CloningInfoArgs{
* AppSettingsOverrides: pulumi.StringMap{
* "Setting1": pulumi.String("NewValue1"),
* "Setting3": pulumi.String("NewValue5"),
* },
* CloneCustomHostNames: pulumi.Bool(true),
* CloneSourceControl: pulumi.Bool(true),
* ConfigureLoadBalancing: pulumi.Bool(false),
* HostingEnvironment: pulumi.String("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg456/providers/Microsoft.Web/hostingenvironments/aseforsites"),
* Overwrite: pulumi.Bool(false),
* SourceWebAppId: pulumi.String("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg456/providers/Microsoft.Web/sites/srcsiteg478"),
* SourceWebAppLocation: pulumi.String("West Europe"),
* },
* Kind: pulumi.String("app"),
* Location: pulumi.String("East US"),
* Name: pulumi.String("sitef6141"),
* ResourceGroupName: pulumi.String("testrg123"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.web.WebApp;
* import com.pulumi.azurenative.web.WebAppArgs;
* import com.pulumi.azurenative.web.inputs.CloningInfoArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var webApp = new WebApp("webApp", WebAppArgs.builder()
* .cloningInfo(CloningInfoArgs.builder()
* .appSettingsOverrides(Map.ofEntries(
* Map.entry("Setting1", "NewValue1"),
* Map.entry("Setting3", "NewValue5")
* ))
* .cloneCustomHostNames(true)
* .cloneSourceControl(true)
* .configureLoadBalancing(false)
* .hostingEnvironment("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg456/providers/Microsoft.Web/hostingenvironments/aseforsites")
* .overwrite(false)
* .sourceWebAppId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg456/providers/Microsoft.Web/sites/srcsiteg478")
* .sourceWebAppLocation("West Europe")
* .build())
* .kind("app")
* .location("East US")
* .name("sitef6141")
* .resourceGroupName("testrg123")
* .build());
* }
* }
* ```
* ### Create or Update web app
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var webApp = new AzureNative.Web.WebApp("webApp", new()
* {
* Kind = "app",
* Location = "East US",
* Name = "sitef6141",
* ResourceGroupName = "testrg123",
* ServerFarmId = "/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg123/providers/Microsoft.Web/serverfarms/DefaultAsp",
* });
* });
* ```
* ```go
* package main
* import (
* web "github.com/pulumi/pulumi-azure-native-sdk/web/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := web.NewWebApp(ctx, "webApp", &web.WebAppArgs{
* Kind: pulumi.String("app"),
* Location: pulumi.String("East US"),
* Name: pulumi.String("sitef6141"),
* ResourceGroupName: pulumi.String("testrg123"),
* ServerFarmId: pulumi.String("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg123/providers/Microsoft.Web/serverfarms/DefaultAsp"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.web.WebApp;
* import com.pulumi.azurenative.web.WebAppArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var webApp = new WebApp("webApp", WebAppArgs.builder()
* .kind("app")
* .location("East US")
* .name("sitef6141")
* .resourceGroupName("testrg123")
* .serverFarmId("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/testrg123/providers/Microsoft.Web/serverfarms/DefaultAsp")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:web:WebApp sitef6141 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Web/sites/{name}
* ```
*/
public class WebApp internal constructor(
override val javaResource: com.pulumi.azurenative.web.WebApp,
) : KotlinCustomResource(javaResource, WebAppMapper) {
/**
* Management information availability state for the app.
*/
public val availabilityState: Output
get() = javaResource.availabilityState().applyValue({ args0 -> args0 })
/**
* true
to enable client affinity; false
to stop sending session affinity cookies, which route client requests in the same session to the same instance. Default is true
.
*/
public val clientAffinityEnabled: Output?
get() = javaResource.clientAffinityEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* true
to enable client certificate authentication (TLS mutual authentication); otherwise, false
. Default is false
.
*/
public val clientCertEnabled: Output?
get() = javaResource.clientCertEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* client certificate authentication comma-separated exclusion paths
*/
public val clientCertExclusionPaths: Output?
get() = javaResource.clientCertExclusionPaths().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* This composes with ClientCertEnabled setting.
* - ClientCertEnabled: false means ClientCert is ignored.
* - ClientCertEnabled: true and ClientCertMode: Required means ClientCert is required.
* - ClientCertEnabled: true and ClientCertMode: Optional means ClientCert is optional or accepted.
*/
public val clientCertMode: Output?
get() = javaResource.clientCertMode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Size of the function container.
*/
public val containerSize: Output?
get() = javaResource.containerSize().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Unique identifier that verifies the custom domains assigned to the app. Customer will add this id to a txt record for verification.
*/
public val customDomainVerificationId: Output?
get() = javaResource.customDomainVerificationId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Maximum allowed daily memory-time quota (applicable on dynamic apps only).
*/
public val dailyMemoryTimeQuota: Output?
get() = javaResource.dailyMemoryTimeQuota().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Default hostname of the app. Read-only.
*/
public val defaultHostName: Output
get() = javaResource.defaultHostName().applyValue({ args0 -> args0 })
/**
* true
if the app is enabled; otherwise, false
. Setting this value to false disables the app (takes the app offline).
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Enabled hostnames for the app.Hostnames need to be assigned (see HostNames) AND enabled. Otherwise,
* the app is not served on those hostnames.
*/
public val enabledHostNames: Output>
get() = javaResource.enabledHostNames().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Extended Location.
*/
public val extendedLocation: Output?
get() = javaResource.extendedLocation().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> extendedLocationResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Hostname SSL states are used to manage the SSL bindings for app's hostnames.
*/
public val hostNameSslStates: Output>?
get() = javaResource.hostNameSslStates().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
hostNameSslStateResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Hostnames associated with the app.
*/
public val hostNames: Output>
get() = javaResource.hostNames().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* true
to disable the public hostnames of the app; otherwise, false
.
* If true
, the app is only accessible via API management process.
*/
public val hostNamesDisabled: Output?
get() = javaResource.hostNamesDisabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* App Service Environment to use for the app.
*/
public val hostingEnvironmentProfile: Output?
get() = javaResource.hostingEnvironmentProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> hostingEnvironmentProfileResponseToKotlin(args0) })
}).orElse(null)
})
/**
* HttpsOnly: configures a web site to accept only https requests. Issues redirect for
* http requests
*/
public val httpsOnly: Output?
get() = javaResource.httpsOnly().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Hyper-V sandbox.
*/
public val hyperV: Output?
get() = javaResource.hyperV().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Managed service identity.
*/
public val identity: Output?
get() = javaResource.identity().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
managedServiceIdentityResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Specifies an operation id if this site has a pending operation.
*/
public val inProgressOperationId: Output
get() = javaResource.inProgressOperationId().applyValue({ args0 -> args0 })
/**
* true
if the app is a default container; otherwise, false
.
*/
public val isDefaultContainer: Output
get() = javaResource.isDefaultContainer().applyValue({ args0 -> args0 })
/**
* Obsolete: Hyper-V sandbox.
*/
public val isXenon: Output?
get() = javaResource.isXenon().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Identity to use for Key Vault Reference authentication.
*/
public val keyVaultReferenceIdentity: Output?
get() = javaResource.keyVaultReferenceIdentity().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Kind of resource.
*/
public val kind: Output?
get() = javaResource.kind().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Last time the app was modified, in UTC. Read-only.
*/
public val lastModifiedTimeUtc: Output
get() = javaResource.lastModifiedTimeUtc().applyValue({ args0 -> args0 })
/**
* Resource Location.
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* Azure Resource Manager ID of the customer's selected Managed Environment on which to host this app. This must be of the form /subscriptions/{subscriptionId}/resourceGroups/{resourceGroup}/providers/Microsoft.App/managedEnvironments/{managedEnvironmentName}
*/
public val managedEnvironmentId: Output?
get() = javaResource.managedEnvironmentId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Maximum number of workers.
* This only applies to Functions container.
*/
public val maxNumberOfWorkers: Output
get() = javaResource.maxNumberOfWorkers().applyValue({ args0 -> args0 })
/**
* Resource Name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from tenants that site can be hosted with current settings. Read-only.
*/
public val outboundIpAddresses: Output
get() = javaResource.outboundIpAddresses().applyValue({ args0 -> args0 })
/**
* List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from all tenants except dataComponent. Read-only.
*/
public val possibleOutboundIpAddresses: Output
get() = javaResource.possibleOutboundIpAddresses().applyValue({ args0 -> args0 })
/**
* Property to allow or block all public traffic. Allowed Values: 'Enabled', 'Disabled' or an empty string.
*/
public val publicNetworkAccess: Output?
get() = javaResource.publicNetworkAccess().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Site redundancy mode
*/
public val redundancyMode: Output?
get() = javaResource.redundancyMode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Name of the repository site.
*/
public val repositorySiteName: Output
get() = javaResource.repositorySiteName().applyValue({ args0 -> args0 })
/**
* true
if reserved; otherwise, false
.
*/
public val reserved: Output?
get() = javaResource.reserved().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Name of the resource group the app belongs to. Read-only.
*/
public val resourceGroup: Output
get() = javaResource.resourceGroup().applyValue({ args0 -> args0 })
/**
* true
to stop SCM (KUDU) site when the app is stopped; otherwise, false
. The default is false
.
*/
public val scmSiteAlsoStopped: Output?
get() = javaResource.scmSiteAlsoStopped().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Resource ID of the associated App Service plan, formatted as: "/subscriptions/{subscriptionID}/resourceGroups/{groupName}/providers/Microsoft.Web/serverfarms/{appServicePlanName}".
*/
public val serverFarmId: Output?
get() = javaResource.serverFarmId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Configuration of the app.
*/
public val siteConfig: Output?
get() = javaResource.siteConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
siteConfigResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Status of the last deployment slot swap operation.
*/
public val slotSwapStatus: Output
get() = javaResource.slotSwapStatus().applyValue({ args0 ->
args0.let({ args0 ->
slotSwapStatusResponseToKotlin(args0)
})
})
/**
* Current state of the app.
*/
public val state: Output
get() = javaResource.state().applyValue({ args0 -> args0 })
/**
* Checks if Customer provided storage account is required
*/
public val storageAccountRequired: Output?
get() = javaResource.storageAccountRequired().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* App suspended till in case memory-time quota is exceeded.
*/
public val suspendedTill: Output
get() = javaResource.suspendedTill().applyValue({ args0 -> args0 })
/**
* Resource tags.
*/
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy