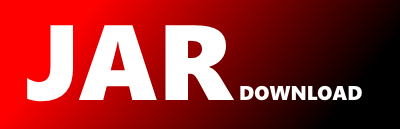
com.pulumi.azurenative.web.kotlin.WebAppDeploymentSlotArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.web.kotlin
import com.pulumi.azurenative.web.WebAppDeploymentSlotArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* User credentials used for publishing activity.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2020-12-01.
* Other available API versions: 2020-10-01, 2023-01-01, 2023-12-01.
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:web:WebAppDeploymentSlot myresource1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Web/sites/{name}/slots/{slot}/deployments/{id}
* ```
* @property active True if deployment is currently active, false if completed and null if not started.
* @property author Who authored the deployment.
* @property authorEmail Author email.
* @property deployer Who performed the deployment.
* @property details Details on deployment.
* @property endTime End time.
* @property id ID of an existing deployment.
* @property kind Kind of resource.
* @property message Details about deployment status.
* @property name Name of the app.
* @property resourceGroupName Name of the resource group to which the resource belongs.
* @property slot Name of the deployment slot. If a slot is not specified, the API creates a deployment for the production slot.
* @property startTime Start time.
* @property status Deployment status.
*/
public data class WebAppDeploymentSlotArgs(
public val active: Output? = null,
public val author: Output? = null,
public val authorEmail: Output? = null,
public val deployer: Output? = null,
public val details: Output? = null,
public val endTime: Output? = null,
public val id: Output? = null,
public val kind: Output? = null,
public val message: Output? = null,
public val name: Output? = null,
public val resourceGroupName: Output? = null,
public val slot: Output? = null,
public val startTime: Output? = null,
public val status: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.web.WebAppDeploymentSlotArgs =
com.pulumi.azurenative.web.WebAppDeploymentSlotArgs.builder()
.active(active?.applyValue({ args0 -> args0 }))
.author(author?.applyValue({ args0 -> args0 }))
.authorEmail(authorEmail?.applyValue({ args0 -> args0 }))
.deployer(deployer?.applyValue({ args0 -> args0 }))
.details(details?.applyValue({ args0 -> args0 }))
.endTime(endTime?.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.message(message?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.slot(slot?.applyValue({ args0 -> args0 }))
.startTime(startTime?.applyValue({ args0 -> args0 }))
.status(status?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WebAppDeploymentSlotArgs].
*/
@PulumiTagMarker
public class WebAppDeploymentSlotArgsBuilder internal constructor() {
private var active: Output? = null
private var author: Output? = null
private var authorEmail: Output? = null
private var deployer: Output? = null
private var details: Output? = null
private var endTime: Output? = null
private var id: Output? = null
private var kind: Output? = null
private var message: Output? = null
private var name: Output? = null
private var resourceGroupName: Output? = null
private var slot: Output? = null
private var startTime: Output? = null
private var status: Output? = null
/**
* @param value True if deployment is currently active, false if completed and null if not started.
*/
@JvmName("talgpkkrhvwrfdxm")
public suspend fun active(`value`: Output) {
this.active = value
}
/**
* @param value Who authored the deployment.
*/
@JvmName("mjiogddwusnlxxsv")
public suspend fun author(`value`: Output) {
this.author = value
}
/**
* @param value Author email.
*/
@JvmName("fwwykgxthoaslrap")
public suspend fun authorEmail(`value`: Output) {
this.authorEmail = value
}
/**
* @param value Who performed the deployment.
*/
@JvmName("hjffwnuecilnxowc")
public suspend fun deployer(`value`: Output) {
this.deployer = value
}
/**
* @param value Details on deployment.
*/
@JvmName("ytojjpgiupjldlbf")
public suspend fun details(`value`: Output) {
this.details = value
}
/**
* @param value End time.
*/
@JvmName("gopfmiseekgrpoin")
public suspend fun endTime(`value`: Output) {
this.endTime = value
}
/**
* @param value ID of an existing deployment.
*/
@JvmName("kstveprclklkhock")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value Kind of resource.
*/
@JvmName("kcaenbsvqfsdphfn")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Details about deployment status.
*/
@JvmName("ijexcrrjsanvpjpf")
public suspend fun message(`value`: Output) {
this.message = value
}
/**
* @param value Name of the app.
*/
@JvmName("fvlepsifqnckjuuq")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Name of the resource group to which the resource belongs.
*/
@JvmName("wfscqtplvuogmqgo")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Name of the deployment slot. If a slot is not specified, the API creates a deployment for the production slot.
*/
@JvmName("krsbchlyxqksymwi")
public suspend fun slot(`value`: Output) {
this.slot = value
}
/**
* @param value Start time.
*/
@JvmName("qeexgxnitfqlvfhn")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value Deployment status.
*/
@JvmName("vogkrkdkhlbqtiwa")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value True if deployment is currently active, false if completed and null if not started.
*/
@JvmName("wudmpkumehjhthce")
public suspend fun active(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.active = mapped
}
/**
* @param value Who authored the deployment.
*/
@JvmName("chbnosdtydeggswm")
public suspend fun author(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.author = mapped
}
/**
* @param value Author email.
*/
@JvmName("nxfrymrethtdmtna")
public suspend fun authorEmail(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authorEmail = mapped
}
/**
* @param value Who performed the deployment.
*/
@JvmName("ygrgqvjaimwusjdc")
public suspend fun deployer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deployer = mapped
}
/**
* @param value Details on deployment.
*/
@JvmName("ugnmnlgjwhmgqnqv")
public suspend fun details(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.details = mapped
}
/**
* @param value End time.
*/
@JvmName("wleocadcdddiodjs")
public suspend fun endTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTime = mapped
}
/**
* @param value ID of an existing deployment.
*/
@JvmName("bnjnrnldbfprknqs")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value Kind of resource.
*/
@JvmName("lvwwdksyaycunnkl")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Details about deployment status.
*/
@JvmName("yaxiqkwlrupbgeik")
public suspend fun message(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.message = mapped
}
/**
* @param value Name of the app.
*/
@JvmName("ahfewsanboctybkk")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Name of the resource group to which the resource belongs.
*/
@JvmName("pjuoewqsvddamjjy")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Name of the deployment slot. If a slot is not specified, the API creates a deployment for the production slot.
*/
@JvmName("oakkvesdtyeuhvvi")
public suspend fun slot(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.slot = mapped
}
/**
* @param value Start time.
*/
@JvmName("mhyrnurkxkvvxrki")
public suspend fun startTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTime = mapped
}
/**
* @param value Deployment status.
*/
@JvmName("qcbeqoqaiwwwenvx")
public suspend fun status(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
internal fun build(): WebAppDeploymentSlotArgs = WebAppDeploymentSlotArgs(
active = active,
author = author,
authorEmail = authorEmail,
deployer = deployer,
details = details,
endTime = endTime,
id = id,
kind = kind,
message = message,
name = name,
resourceGroupName = resourceGroupName,
slot = slot,
startTime = startTime,
status = status,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy