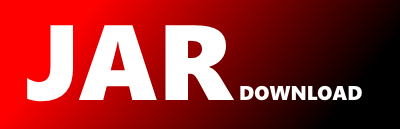
com.pulumi.azurenative.web.kotlin.inputs.CustomApiPropertiesDefinitionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.web.kotlin.inputs
import com.pulumi.azurenative.web.inputs.CustomApiPropertiesDefinitionArgs.builder
import com.pulumi.azurenative.web.kotlin.enums.ApiType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Custom API properties
* @property apiDefinitions API Definitions
* @property apiType The API type
* @property backendService The API backend service
* @property brandColor Brand color
* @property capabilities The custom API capabilities
* @property connectionParameters Connection parameters
* @property description The custom API description
* @property displayName The display name
* @property iconUri The icon URI
* @property runtimeUrls Runtime URLs
* @property swagger The JSON representation of the swagger
* @property wsdlDefinition The WSDL definition
*/
public data class CustomApiPropertiesDefinitionArgs(
public val apiDefinitions: Output? = null,
public val apiType: Output>? = null,
public val backendService: Output? = null,
public val brandColor: Output? = null,
public val capabilities: Output>? = null,
public val connectionParameters: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy