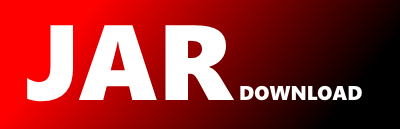
com.pulumi.azurenative.web.kotlin.outputs.GetAppServiceEnvironmentResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.web.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* App Service Environment ARM resource.
* @property clusterSettings Custom settings for changing the behavior of the App Service Environment.
* @property customDnsSuffixConfiguration Full view of the custom domain suffix configuration for ASEv3.
* @property dedicatedHostCount Dedicated Host Count
* @property dnsSuffix DNS suffix of the App Service Environment.
* @property frontEndScaleFactor Scale factor for front-ends.
* @property hasLinuxWorkers Flag that displays whether an ASE has linux workers or not
* @property id Resource Id.
* @property internalLoadBalancingMode Specifies which endpoints to serve internally in the Virtual Network for the App Service Environment.
* @property ipsslAddressCount Number of IP SSL addresses reserved for the App Service Environment.
* @property kind Kind of resource.
* @property location Resource Location.
* @property maximumNumberOfMachines Maximum number of VMs in the App Service Environment.
* @property multiRoleCount Number of front-end instances.
* @property multiSize Front-end VM size, e.g. "Medium", "Large".
* @property name Resource Name.
* @property networkingConfiguration Full view of networking configuration for an ASE.
* @property provisioningState Provisioning state of the App Service Environment.
* @property status Current status of the App Service Environment.
* @property suspended true
if the App Service Environment is suspended; otherwise, false
. The environment can be suspended, e.g. when the management endpoint is no longer available
* (most likely because NSG blocked the incoming traffic).
* @property tags Resource tags.
* @property type Resource type.
* @property upgradeAvailability Whether an upgrade is available for this App Service Environment.
* @property upgradePreference Upgrade Preference
* @property userWhitelistedIpRanges User added ip ranges to whitelist on ASE db
* @property virtualNetwork Description of the Virtual Network.
* @property zoneRedundant Whether or not this App Service Environment is zone-redundant.
*/
public data class GetAppServiceEnvironmentResult(
public val clusterSettings: List? = null,
public val customDnsSuffixConfiguration: CustomDnsSuffixConfigurationResponse? = null,
public val dedicatedHostCount: Int? = null,
public val dnsSuffix: String? = null,
public val frontEndScaleFactor: Int? = null,
public val hasLinuxWorkers: Boolean,
public val id: String,
public val internalLoadBalancingMode: String? = null,
public val ipsslAddressCount: Int? = null,
public val kind: String? = null,
public val location: String,
public val maximumNumberOfMachines: Int,
public val multiRoleCount: Int,
public val multiSize: String? = null,
public val name: String,
public val networkingConfiguration: AseV3NetworkingConfigurationResponse? = null,
public val provisioningState: String,
public val status: String,
public val suspended: Boolean,
public val tags: Map? = null,
public val type: String,
public val upgradeAvailability: String,
public val upgradePreference: String? = null,
public val userWhitelistedIpRanges: List? = null,
public val virtualNetwork: VirtualNetworkProfileResponse,
public val zoneRedundant: Boolean? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.web.outputs.GetAppServiceEnvironmentResult): GetAppServiceEnvironmentResult = GetAppServiceEnvironmentResult(
clusterSettings = javaType.clusterSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.NameValuePairResponse.Companion.toKotlin(args0)
})
}),
customDnsSuffixConfiguration = javaType.customDnsSuffixConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.CustomDnsSuffixConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
dedicatedHostCount = javaType.dedicatedHostCount().map({ args0 -> args0 }).orElse(null),
dnsSuffix = javaType.dnsSuffix().map({ args0 -> args0 }).orElse(null),
frontEndScaleFactor = javaType.frontEndScaleFactor().map({ args0 -> args0 }).orElse(null),
hasLinuxWorkers = javaType.hasLinuxWorkers(),
id = javaType.id(),
internalLoadBalancingMode = javaType.internalLoadBalancingMode().map({ args0 ->
args0
}).orElse(null),
ipsslAddressCount = javaType.ipsslAddressCount().map({ args0 -> args0 }).orElse(null),
kind = javaType.kind().map({ args0 -> args0 }).orElse(null),
location = javaType.location(),
maximumNumberOfMachines = javaType.maximumNumberOfMachines(),
multiRoleCount = javaType.multiRoleCount(),
multiSize = javaType.multiSize().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
networkingConfiguration = javaType.networkingConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.AseV3NetworkingConfigurationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
provisioningState = javaType.provisioningState(),
status = javaType.status(),
suspended = javaType.suspended(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
upgradeAvailability = javaType.upgradeAvailability(),
upgradePreference = javaType.upgradePreference().map({ args0 -> args0 }).orElse(null),
userWhitelistedIpRanges = javaType.userWhitelistedIpRanges().map({ args0 -> args0 }),
virtualNetwork = javaType.virtualNetwork().let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.VirtualNetworkProfileResponse.Companion.toKotlin(args0)
}),
zoneRedundant = javaType.zoneRedundant().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy