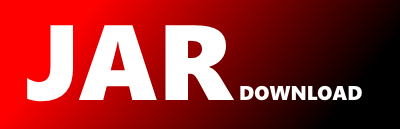
com.pulumi.azurenative.web.kotlin.outputs.GetWebAppSlotResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.web.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A web app, a mobile app backend, or an API app.
* @property availabilityState Management information availability state for the app.
* @property clientAffinityEnabled true
to enable client affinity; false
to stop sending session affinity cookies, which route client requests in the same session to the same instance. Default is true
.
* @property clientCertEnabled true
to enable client certificate authentication (TLS mutual authentication); otherwise, false
. Default is false
.
* @property clientCertExclusionPaths client certificate authentication comma-separated exclusion paths
* @property clientCertMode This composes with ClientCertEnabled setting.
* - ClientCertEnabled: false means ClientCert is ignored.
* - ClientCertEnabled: true and ClientCertMode: Required means ClientCert is required.
* - ClientCertEnabled: true and ClientCertMode: Optional means ClientCert is optional or accepted.
* @property containerSize Size of the function container.
* @property customDomainVerificationId Unique identifier that verifies the custom domains assigned to the app. Customer will add this id to a txt record for verification.
* @property dailyMemoryTimeQuota Maximum allowed daily memory-time quota (applicable on dynamic apps only).
* @property defaultHostName Default hostname of the app. Read-only.
* @property enabled true
if the app is enabled; otherwise, false
. Setting this value to false disables the app (takes the app offline).
* @property enabledHostNames Enabled hostnames for the app.Hostnames need to be assigned (see HostNames) AND enabled. Otherwise,
* the app is not served on those hostnames.
* @property extendedLocation Extended Location.
* @property hostNameSslStates Hostname SSL states are used to manage the SSL bindings for app's hostnames.
* @property hostNames Hostnames associated with the app.
* @property hostNamesDisabled true
to disable the public hostnames of the app; otherwise, false
.
* If true
, the app is only accessible via API management process.
* @property hostingEnvironmentProfile App Service Environment to use for the app.
* @property httpsOnly HttpsOnly: configures a web site to accept only https requests. Issues redirect for
* http requests
* @property hyperV Hyper-V sandbox.
* @property id Resource Id.
* @property identity Managed service identity.
* @property inProgressOperationId Specifies an operation id if this site has a pending operation.
* @property isDefaultContainer true
if the app is a default container; otherwise, false
.
* @property isXenon Obsolete: Hyper-V sandbox.
* @property keyVaultReferenceIdentity Identity to use for Key Vault Reference authentication.
* @property kind Kind of resource.
* @property lastModifiedTimeUtc Last time the app was modified, in UTC. Read-only.
* @property location Resource Location.
* @property managedEnvironmentId Azure Resource Manager ID of the customer's selected Managed Environment on which to host this app. This must be of the form /subscriptions/{subscriptionId}/resourceGroups/{resourceGroup}/providers/Microsoft.App/managedEnvironments/{managedEnvironmentName}
* @property maxNumberOfWorkers Maximum number of workers.
* This only applies to Functions container.
* @property name Resource Name.
* @property outboundIpAddresses List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from tenants that site can be hosted with current settings. Read-only.
* @property possibleOutboundIpAddresses List of IP addresses that the app uses for outbound connections (e.g. database access). Includes VIPs from all tenants except dataComponent. Read-only.
* @property publicNetworkAccess Property to allow or block all public traffic. Allowed Values: 'Enabled', 'Disabled' or an empty string.
* @property redundancyMode Site redundancy mode
* @property repositorySiteName Name of the repository site.
* @property reserved true
if reserved; otherwise, false
.
* @property resourceGroup Name of the resource group the app belongs to. Read-only.
* @property scmSiteAlsoStopped true
to stop SCM (KUDU) site when the app is stopped; otherwise, false
. The default is false
.
* @property serverFarmId Resource ID of the associated App Service plan, formatted as: "/subscriptions/{subscriptionID}/resourceGroups/{groupName}/providers/Microsoft.Web/serverfarms/{appServicePlanName}".
* @property siteConfig Configuration of the app.
* @property slotSwapStatus Status of the last deployment slot swap operation.
* @property state Current state of the app.
* @property storageAccountRequired Checks if Customer provided storage account is required
* @property suspendedTill App suspended till in case memory-time quota is exceeded.
* @property tags Resource tags.
* @property targetSwapSlot Specifies which deployment slot this app will swap into. Read-only.
* @property trafficManagerHostNames Azure Traffic Manager hostnames associated with the app. Read-only.
* @property type Resource type.
* @property usageState State indicating whether the app has exceeded its quota usage. Read-only.
* @property virtualNetworkSubnetId Azure Resource Manager ID of the Virtual network and subnet to be joined by Regional VNET Integration.
* This must be of the form /subscriptions/{subscriptionName}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualNetworks/{vnetName}/subnets/{subnetName}
* @property vnetContentShareEnabled To enable accessing content over virtual network
* @property vnetImagePullEnabled To enable pulling image over Virtual Network
* @property vnetRouteAllEnabled Virtual Network Route All enabled. This causes all outbound traffic to have Virtual Network Security Groups and User Defined Routes applied.
*/
public data class GetWebAppSlotResult(
public val availabilityState: String,
public val clientAffinityEnabled: Boolean? = null,
public val clientCertEnabled: Boolean? = null,
public val clientCertExclusionPaths: String? = null,
public val clientCertMode: String? = null,
public val containerSize: Int? = null,
public val customDomainVerificationId: String? = null,
public val dailyMemoryTimeQuota: Int? = null,
public val defaultHostName: String,
public val enabled: Boolean? = null,
public val enabledHostNames: List,
public val extendedLocation: ExtendedLocationResponse? = null,
public val hostNameSslStates: List? = null,
public val hostNames: List,
public val hostNamesDisabled: Boolean? = null,
public val hostingEnvironmentProfile: HostingEnvironmentProfileResponse? = null,
public val httpsOnly: Boolean? = null,
public val hyperV: Boolean? = null,
public val id: String,
public val identity: ManagedServiceIdentityResponse? = null,
public val inProgressOperationId: String,
public val isDefaultContainer: Boolean,
public val isXenon: Boolean? = null,
public val keyVaultReferenceIdentity: String? = null,
public val kind: String? = null,
public val lastModifiedTimeUtc: String,
public val location: String,
public val managedEnvironmentId: String? = null,
public val maxNumberOfWorkers: Int,
public val name: String,
public val outboundIpAddresses: String,
public val possibleOutboundIpAddresses: String,
public val publicNetworkAccess: String? = null,
public val redundancyMode: String? = null,
public val repositorySiteName: String,
public val reserved: Boolean? = null,
public val resourceGroup: String,
public val scmSiteAlsoStopped: Boolean? = null,
public val serverFarmId: String? = null,
public val siteConfig: SiteConfigResponse? = null,
public val slotSwapStatus: SlotSwapStatusResponse,
public val state: String,
public val storageAccountRequired: Boolean? = null,
public val suspendedTill: String,
public val tags: Map? = null,
public val targetSwapSlot: String,
public val trafficManagerHostNames: List,
public val type: String,
public val usageState: String,
public val virtualNetworkSubnetId: String? = null,
public val vnetContentShareEnabled: Boolean? = null,
public val vnetImagePullEnabled: Boolean? = null,
public val vnetRouteAllEnabled: Boolean? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.web.outputs.GetWebAppSlotResult): GetWebAppSlotResult = GetWebAppSlotResult(
availabilityState = javaType.availabilityState(),
clientAffinityEnabled = javaType.clientAffinityEnabled().map({ args0 -> args0 }).orElse(null),
clientCertEnabled = javaType.clientCertEnabled().map({ args0 -> args0 }).orElse(null),
clientCertExclusionPaths = javaType.clientCertExclusionPaths().map({ args0 -> args0 }).orElse(null),
clientCertMode = javaType.clientCertMode().map({ args0 -> args0 }).orElse(null),
containerSize = javaType.containerSize().map({ args0 -> args0 }).orElse(null),
customDomainVerificationId = javaType.customDomainVerificationId().map({ args0 ->
args0
}).orElse(null),
dailyMemoryTimeQuota = javaType.dailyMemoryTimeQuota().map({ args0 -> args0 }).orElse(null),
defaultHostName = javaType.defaultHostName(),
enabled = javaType.enabled().map({ args0 -> args0 }).orElse(null),
enabledHostNames = javaType.enabledHostNames().map({ args0 -> args0 }),
extendedLocation = javaType.extendedLocation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.ExtendedLocationResponse.Companion.toKotlin(args0)
})
}).orElse(null),
hostNameSslStates = javaType.hostNameSslStates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.HostNameSslStateResponse.Companion.toKotlin(args0)
})
}),
hostNames = javaType.hostNames().map({ args0 -> args0 }),
hostNamesDisabled = javaType.hostNamesDisabled().map({ args0 -> args0 }).orElse(null),
hostingEnvironmentProfile = javaType.hostingEnvironmentProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.HostingEnvironmentProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
httpsOnly = javaType.httpsOnly().map({ args0 -> args0 }).orElse(null),
hyperV = javaType.hyperV().map({ args0 -> args0 }).orElse(null),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.ManagedServiceIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
inProgressOperationId = javaType.inProgressOperationId(),
isDefaultContainer = javaType.isDefaultContainer(),
isXenon = javaType.isXenon().map({ args0 -> args0 }).orElse(null),
keyVaultReferenceIdentity = javaType.keyVaultReferenceIdentity().map({ args0 ->
args0
}).orElse(null),
kind = javaType.kind().map({ args0 -> args0 }).orElse(null),
lastModifiedTimeUtc = javaType.lastModifiedTimeUtc(),
location = javaType.location(),
managedEnvironmentId = javaType.managedEnvironmentId().map({ args0 -> args0 }).orElse(null),
maxNumberOfWorkers = javaType.maxNumberOfWorkers(),
name = javaType.name(),
outboundIpAddresses = javaType.outboundIpAddresses(),
possibleOutboundIpAddresses = javaType.possibleOutboundIpAddresses(),
publicNetworkAccess = javaType.publicNetworkAccess().map({ args0 -> args0 }).orElse(null),
redundancyMode = javaType.redundancyMode().map({ args0 -> args0 }).orElse(null),
repositorySiteName = javaType.repositorySiteName(),
reserved = javaType.reserved().map({ args0 -> args0 }).orElse(null),
resourceGroup = javaType.resourceGroup(),
scmSiteAlsoStopped = javaType.scmSiteAlsoStopped().map({ args0 -> args0 }).orElse(null),
serverFarmId = javaType.serverFarmId().map({ args0 -> args0 }).orElse(null),
siteConfig = javaType.siteConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.SiteConfigResponse.Companion.toKotlin(args0)
})
}).orElse(null),
slotSwapStatus = javaType.slotSwapStatus().let({ args0 ->
com.pulumi.azurenative.web.kotlin.outputs.SlotSwapStatusResponse.Companion.toKotlin(args0)
}),
state = javaType.state(),
storageAccountRequired = javaType.storageAccountRequired().map({ args0 -> args0 }).orElse(null),
suspendedTill = javaType.suspendedTill(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
targetSwapSlot = javaType.targetSwapSlot(),
trafficManagerHostNames = javaType.trafficManagerHostNames().map({ args0 -> args0 }),
type = javaType.type(),
usageState = javaType.usageState(),
virtualNetworkSubnetId = javaType.virtualNetworkSubnetId().map({ args0 -> args0 }).orElse(null),
vnetContentShareEnabled = javaType.vnetContentShareEnabled().map({ args0 -> args0 }).orElse(null),
vnetImagePullEnabled = javaType.vnetImagePullEnabled().map({ args0 -> args0 }).orElse(null),
vnetRouteAllEnabled = javaType.vnetRouteAllEnabled().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy