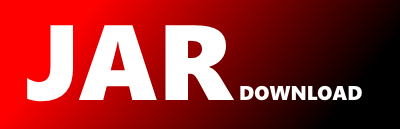
com.pulumi.azurenative.webpubsub.kotlin.WebpubsubFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.webpubsub.kotlin
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.getWebPubSubCustomCertificatePlain
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.getWebPubSubCustomDomainPlain
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.getWebPubSubHubPlain
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.getWebPubSubPlain
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.getWebPubSubPrivateEndpointConnectionPlain
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.getWebPubSubReplicaPlain
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.getWebPubSubSharedPrivateLinkResourcePlain
import com.pulumi.azurenative.webpubsub.WebpubsubFunctions.listWebPubSubKeysPlain
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubCustomCertificatePlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubCustomCertificatePlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubCustomDomainPlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubCustomDomainPlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubHubPlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubHubPlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubPlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubPlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubPrivateEndpointConnectionPlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubPrivateEndpointConnectionPlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubReplicaPlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubReplicaPlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubSharedPrivateLinkResourcePlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubSharedPrivateLinkResourcePlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.inputs.ListWebPubSubKeysPlainArgs
import com.pulumi.azurenative.webpubsub.kotlin.inputs.ListWebPubSubKeysPlainArgsBuilder
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubCustomCertificateResult
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubCustomDomainResult
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubHubResult
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubPrivateEndpointConnectionResult
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubReplicaResult
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubResult
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubSharedPrivateLinkResourceResult
import com.pulumi.azurenative.webpubsub.kotlin.outputs.ListWebPubSubKeysResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubCustomCertificateResult.Companion.toKotlin as getWebPubSubCustomCertificateResultToKotlin
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubCustomDomainResult.Companion.toKotlin as getWebPubSubCustomDomainResultToKotlin
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubHubResult.Companion.toKotlin as getWebPubSubHubResultToKotlin
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubPrivateEndpointConnectionResult.Companion.toKotlin as getWebPubSubPrivateEndpointConnectionResultToKotlin
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubReplicaResult.Companion.toKotlin as getWebPubSubReplicaResultToKotlin
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubResult.Companion.toKotlin as getWebPubSubResultToKotlin
import com.pulumi.azurenative.webpubsub.kotlin.outputs.GetWebPubSubSharedPrivateLinkResourceResult.Companion.toKotlin as getWebPubSubSharedPrivateLinkResourceResultToKotlin
import com.pulumi.azurenative.webpubsub.kotlin.outputs.ListWebPubSubKeysResult.Companion.toKotlin as listWebPubSubKeysResultToKotlin
public object WebpubsubFunctions {
/**
* Get the resource and its properties.
* Azure REST API version: 2023-02-01.
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return A class represent a resource.
*/
public suspend fun getWebPubSub(argument: GetWebPubSubPlainArgs): GetWebPubSubResult =
getWebPubSubResultToKotlin(getWebPubSubPlain(argument.toJava()).await())
/**
* @see [getWebPubSub].
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param resourceName The name of the resource.
* @return A class represent a resource.
*/
public suspend fun getWebPubSub(resourceGroupName: String, resourceName: String): GetWebPubSubResult {
val argument = GetWebPubSubPlainArgs(
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getWebPubSubResultToKotlin(getWebPubSubPlain(argument.toJava()).await())
}
/**
* @see [getWebPubSub].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubPlainArgs].
* @return A class represent a resource.
*/
public suspend fun getWebPubSub(argument: suspend GetWebPubSubPlainArgsBuilder.() -> Unit): GetWebPubSubResult {
val builder = GetWebPubSubPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebPubSubResultToKotlin(getWebPubSubPlain(builtArgument.toJava()).await())
}
/**
* Get a custom certificate.
* Azure REST API version: 2023-02-01.
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return A custom certificate.
*/
public suspend fun getWebPubSubCustomCertificate(argument: GetWebPubSubCustomCertificatePlainArgs): GetWebPubSubCustomCertificateResult =
getWebPubSubCustomCertificateResultToKotlin(getWebPubSubCustomCertificatePlain(argument.toJava()).await())
/**
* @see [getWebPubSubCustomCertificate].
* @param certificateName Custom certificate name
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param resourceName The name of the resource.
* @return A custom certificate.
*/
public suspend fun getWebPubSubCustomCertificate(
certificateName: String,
resourceGroupName: String,
resourceName: String,
): GetWebPubSubCustomCertificateResult {
val argument = GetWebPubSubCustomCertificatePlainArgs(
certificateName = certificateName,
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getWebPubSubCustomCertificateResultToKotlin(getWebPubSubCustomCertificatePlain(argument.toJava()).await())
}
/**
* @see [getWebPubSubCustomCertificate].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubCustomCertificatePlainArgs].
* @return A custom certificate.
*/
public suspend fun getWebPubSubCustomCertificate(argument: suspend GetWebPubSubCustomCertificatePlainArgsBuilder.() -> Unit): GetWebPubSubCustomCertificateResult {
val builder = GetWebPubSubCustomCertificatePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebPubSubCustomCertificateResultToKotlin(getWebPubSubCustomCertificatePlain(builtArgument.toJava()).await())
}
/**
* Get a custom domain.
* Azure REST API version: 2023-02-01.
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return A custom domain
*/
public suspend fun getWebPubSubCustomDomain(argument: GetWebPubSubCustomDomainPlainArgs): GetWebPubSubCustomDomainResult =
getWebPubSubCustomDomainResultToKotlin(getWebPubSubCustomDomainPlain(argument.toJava()).await())
/**
* @see [getWebPubSubCustomDomain].
* @param name Custom domain name.
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param resourceName The name of the resource.
* @return A custom domain
*/
public suspend fun getWebPubSubCustomDomain(
name: String,
resourceGroupName: String,
resourceName: String,
): GetWebPubSubCustomDomainResult {
val argument = GetWebPubSubCustomDomainPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getWebPubSubCustomDomainResultToKotlin(getWebPubSubCustomDomainPlain(argument.toJava()).await())
}
/**
* @see [getWebPubSubCustomDomain].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubCustomDomainPlainArgs].
* @return A custom domain
*/
public suspend fun getWebPubSubCustomDomain(argument: suspend GetWebPubSubCustomDomainPlainArgsBuilder.() -> Unit): GetWebPubSubCustomDomainResult {
val builder = GetWebPubSubCustomDomainPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebPubSubCustomDomainResultToKotlin(getWebPubSubCustomDomainPlain(builtArgument.toJava()).await())
}
/**
* Get a hub setting.
* Azure REST API version: 2023-02-01.
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return A hub setting
*/
public suspend fun getWebPubSubHub(argument: GetWebPubSubHubPlainArgs): GetWebPubSubHubResult =
getWebPubSubHubResultToKotlin(getWebPubSubHubPlain(argument.toJava()).await())
/**
* @see [getWebPubSubHub].
* @param hubName The hub name.
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param resourceName The name of the resource.
* @return A hub setting
*/
public suspend fun getWebPubSubHub(
hubName: String,
resourceGroupName: String,
resourceName: String,
): GetWebPubSubHubResult {
val argument = GetWebPubSubHubPlainArgs(
hubName = hubName,
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getWebPubSubHubResultToKotlin(getWebPubSubHubPlain(argument.toJava()).await())
}
/**
* @see [getWebPubSubHub].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubHubPlainArgs].
* @return A hub setting
*/
public suspend fun getWebPubSubHub(argument: suspend GetWebPubSubHubPlainArgsBuilder.() -> Unit): GetWebPubSubHubResult {
val builder = GetWebPubSubHubPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebPubSubHubResultToKotlin(getWebPubSubHubPlain(builtArgument.toJava()).await())
}
/**
* Get the specified private endpoint connection
* Azure REST API version: 2023-02-01.
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return A private endpoint connection to an azure resource
*/
public suspend fun getWebPubSubPrivateEndpointConnection(argument: GetWebPubSubPrivateEndpointConnectionPlainArgs): GetWebPubSubPrivateEndpointConnectionResult =
getWebPubSubPrivateEndpointConnectionResultToKotlin(getWebPubSubPrivateEndpointConnectionPlain(argument.toJava()).await())
/**
* @see [getWebPubSubPrivateEndpointConnection].
* @param privateEndpointConnectionName The name of the private endpoint connection
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param resourceName The name of the resource.
* @return A private endpoint connection to an azure resource
*/
public suspend fun getWebPubSubPrivateEndpointConnection(
privateEndpointConnectionName: String,
resourceGroupName: String,
resourceName: String,
): GetWebPubSubPrivateEndpointConnectionResult {
val argument = GetWebPubSubPrivateEndpointConnectionPlainArgs(
privateEndpointConnectionName = privateEndpointConnectionName,
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getWebPubSubPrivateEndpointConnectionResultToKotlin(getWebPubSubPrivateEndpointConnectionPlain(argument.toJava()).await())
}
/**
* @see [getWebPubSubPrivateEndpointConnection].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubPrivateEndpointConnectionPlainArgs].
* @return A private endpoint connection to an azure resource
*/
public suspend fun getWebPubSubPrivateEndpointConnection(argument: suspend GetWebPubSubPrivateEndpointConnectionPlainArgsBuilder.() -> Unit): GetWebPubSubPrivateEndpointConnectionResult {
val builder = GetWebPubSubPrivateEndpointConnectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebPubSubPrivateEndpointConnectionResultToKotlin(getWebPubSubPrivateEndpointConnectionPlain(builtArgument.toJava()).await())
}
/**
* Get the replica and its properties.
* Azure REST API version: 2023-03-01-preview.
* Other available API versions: 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return A class represent a replica resource.
*/
public suspend fun getWebPubSubReplica(argument: GetWebPubSubReplicaPlainArgs): GetWebPubSubReplicaResult =
getWebPubSubReplicaResultToKotlin(getWebPubSubReplicaPlain(argument.toJava()).await())
/**
* @see [getWebPubSubReplica].
* @param replicaName The name of the replica.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param resourceName The name of the resource.
* @return A class represent a replica resource.
*/
public suspend fun getWebPubSubReplica(
replicaName: String,
resourceGroupName: String,
resourceName: String,
): GetWebPubSubReplicaResult {
val argument = GetWebPubSubReplicaPlainArgs(
replicaName = replicaName,
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return getWebPubSubReplicaResultToKotlin(getWebPubSubReplicaPlain(argument.toJava()).await())
}
/**
* @see [getWebPubSubReplica].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubReplicaPlainArgs].
* @return A class represent a replica resource.
*/
public suspend fun getWebPubSubReplica(argument: suspend GetWebPubSubReplicaPlainArgsBuilder.() -> Unit): GetWebPubSubReplicaResult {
val builder = GetWebPubSubReplicaPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebPubSubReplicaResultToKotlin(getWebPubSubReplicaPlain(builtArgument.toJava()).await())
}
/**
* Get the specified shared private link resource
* Azure REST API version: 2023-02-01.
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return Describes a Shared Private Link Resource
*/
public suspend fun getWebPubSubSharedPrivateLinkResource(argument: GetWebPubSubSharedPrivateLinkResourcePlainArgs): GetWebPubSubSharedPrivateLinkResourceResult =
getWebPubSubSharedPrivateLinkResourceResultToKotlin(getWebPubSubSharedPrivateLinkResourcePlain(argument.toJava()).await())
/**
* @see [getWebPubSubSharedPrivateLinkResource].
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param resourceName The name of the resource.
* @param sharedPrivateLinkResourceName The name of the shared private link resource
* @return Describes a Shared Private Link Resource
*/
public suspend fun getWebPubSubSharedPrivateLinkResource(
resourceGroupName: String,
resourceName: String,
sharedPrivateLinkResourceName: String,
): GetWebPubSubSharedPrivateLinkResourceResult {
val argument = GetWebPubSubSharedPrivateLinkResourcePlainArgs(
resourceGroupName = resourceGroupName,
resourceName = resourceName,
sharedPrivateLinkResourceName = sharedPrivateLinkResourceName,
)
return getWebPubSubSharedPrivateLinkResourceResultToKotlin(getWebPubSubSharedPrivateLinkResourcePlain(argument.toJava()).await())
}
/**
* @see [getWebPubSubSharedPrivateLinkResource].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.GetWebPubSubSharedPrivateLinkResourcePlainArgs].
* @return Describes a Shared Private Link Resource
*/
public suspend fun getWebPubSubSharedPrivateLinkResource(argument: suspend GetWebPubSubSharedPrivateLinkResourcePlainArgsBuilder.() -> Unit): GetWebPubSubSharedPrivateLinkResourceResult {
val builder = GetWebPubSubSharedPrivateLinkResourcePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getWebPubSubSharedPrivateLinkResourceResultToKotlin(getWebPubSubSharedPrivateLinkResourcePlain(builtArgument.toJava()).await())
}
/**
* Get the access keys of the resource.
* Azure REST API version: 2023-02-01.
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview.
* @param argument null
* @return A class represents the access keys of the resource.
*/
public suspend fun listWebPubSubKeys(argument: ListWebPubSubKeysPlainArgs): ListWebPubSubKeysResult =
listWebPubSubKeysResultToKotlin(listWebPubSubKeysPlain(argument.toJava()).await())
/**
* @see [listWebPubSubKeys].
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value from the Azure Resource Manager API or the portal.
* @param resourceName The name of the resource.
* @return A class represents the access keys of the resource.
*/
public suspend fun listWebPubSubKeys(resourceGroupName: String, resourceName: String): ListWebPubSubKeysResult {
val argument = ListWebPubSubKeysPlainArgs(
resourceGroupName = resourceGroupName,
resourceName = resourceName,
)
return listWebPubSubKeysResultToKotlin(listWebPubSubKeysPlain(argument.toJava()).await())
}
/**
* @see [listWebPubSubKeys].
* @param argument Builder for [com.pulumi.azurenative.webpubsub.kotlin.inputs.ListWebPubSubKeysPlainArgs].
* @return A class represents the access keys of the resource.
*/
public suspend fun listWebPubSubKeys(argument: suspend ListWebPubSubKeysPlainArgsBuilder.() -> Unit): ListWebPubSubKeysResult {
val builder = ListWebPubSubKeysPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listWebPubSubKeysResultToKotlin(listWebPubSubKeysPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy