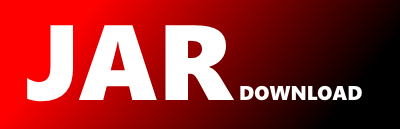
com.pulumi.azurenative.workloads.kotlin.inputs.DB2ProviderInstancePropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.workloads.kotlin.inputs
import com.pulumi.azurenative.workloads.inputs.DB2ProviderInstancePropertiesArgs.builder
import com.pulumi.azurenative.workloads.kotlin.enums.SslPreference
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Gets or sets the DB2 provider properties.
* @property dbName Gets or sets the db2 database name.
* @property dbPassword Gets or sets the db2 database password.
* @property dbPasswordUri Gets or sets the key vault URI to secret with the database password.
* @property dbPort Gets or sets the db2 database sql port.
* @property dbUsername Gets or sets the db2 database user name.
* @property hostname Gets or sets the target virtual machine name.
* @property providerType The provider type. For example, the value can be SapHana.
* Expected value is 'Db2'.
* @property sapSid Gets or sets the SAP System Identifier
* @property sslCertificateUri Gets or sets the blob URI to SSL certificate for the DB2 Database.
* @property sslPreference Gets or sets certificate preference if secure communication is enabled.
*/
public data class DB2ProviderInstancePropertiesArgs(
public val dbName: Output? = null,
public val dbPassword: Output? = null,
public val dbPasswordUri: Output? = null,
public val dbPort: Output? = null,
public val dbUsername: Output? = null,
public val hostname: Output? = null,
public val providerType: Output,
public val sapSid: Output? = null,
public val sslCertificateUri: Output? = null,
public val sslPreference: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.workloads.inputs.DB2ProviderInstancePropertiesArgs =
com.pulumi.azurenative.workloads.inputs.DB2ProviderInstancePropertiesArgs.builder()
.dbName(dbName?.applyValue({ args0 -> args0 }))
.dbPassword(dbPassword?.applyValue({ args0 -> args0 }))
.dbPasswordUri(dbPasswordUri?.applyValue({ args0 -> args0 }))
.dbPort(dbPort?.applyValue({ args0 -> args0 }))
.dbUsername(dbUsername?.applyValue({ args0 -> args0 }))
.hostname(hostname?.applyValue({ args0 -> args0 }))
.providerType(providerType.applyValue({ args0 -> args0 }))
.sapSid(sapSid?.applyValue({ args0 -> args0 }))
.sslCertificateUri(sslCertificateUri?.applyValue({ args0 -> args0 }))
.sslPreference(
sslPreference?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [DB2ProviderInstancePropertiesArgs].
*/
@PulumiTagMarker
public class DB2ProviderInstancePropertiesArgsBuilder internal constructor() {
private var dbName: Output? = null
private var dbPassword: Output? = null
private var dbPasswordUri: Output? = null
private var dbPort: Output? = null
private var dbUsername: Output? = null
private var hostname: Output? = null
private var providerType: Output? = null
private var sapSid: Output? = null
private var sslCertificateUri: Output? = null
private var sslPreference: Output>? = null
/**
* @param value Gets or sets the db2 database name.
*/
@JvmName("ijotmluvnufnkoje")
public suspend fun dbName(`value`: Output) {
this.dbName = value
}
/**
* @param value Gets or sets the db2 database password.
*/
@JvmName("vcmjhgvbcrxyfrul")
public suspend fun dbPassword(`value`: Output) {
this.dbPassword = value
}
/**
* @param value Gets or sets the key vault URI to secret with the database password.
*/
@JvmName("nwxossvpkeawilfa")
public suspend fun dbPasswordUri(`value`: Output) {
this.dbPasswordUri = value
}
/**
* @param value Gets or sets the db2 database sql port.
*/
@JvmName("uueigsqdoghecmym")
public suspend fun dbPort(`value`: Output) {
this.dbPort = value
}
/**
* @param value Gets or sets the db2 database user name.
*/
@JvmName("vdhwfohldljufjvc")
public suspend fun dbUsername(`value`: Output) {
this.dbUsername = value
}
/**
* @param value Gets or sets the target virtual machine name.
*/
@JvmName("jbehldrpmsmvpqkv")
public suspend fun hostname(`value`: Output) {
this.hostname = value
}
/**
* @param value The provider type. For example, the value can be SapHana.
* Expected value is 'Db2'.
*/
@JvmName("jenjfjsetsstqhsd")
public suspend fun providerType(`value`: Output) {
this.providerType = value
}
/**
* @param value Gets or sets the SAP System Identifier
*/
@JvmName("xxivblychagnvghs")
public suspend fun sapSid(`value`: Output) {
this.sapSid = value
}
/**
* @param value Gets or sets the blob URI to SSL certificate for the DB2 Database.
*/
@JvmName("fekexevadghbhmri")
public suspend fun sslCertificateUri(`value`: Output) {
this.sslCertificateUri = value
}
/**
* @param value Gets or sets certificate preference if secure communication is enabled.
*/
@JvmName("saqqfhvylgdasusn")
public suspend fun sslPreference(`value`: Output>) {
this.sslPreference = value
}
/**
* @param value Gets or sets the db2 database name.
*/
@JvmName("ucmxydiqgppilrhw")
public suspend fun dbName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbName = mapped
}
/**
* @param value Gets or sets the db2 database password.
*/
@JvmName("bbguwasyhqtahcsk")
public suspend fun dbPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbPassword = mapped
}
/**
* @param value Gets or sets the key vault URI to secret with the database password.
*/
@JvmName("opwchvikumnvbysy")
public suspend fun dbPasswordUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbPasswordUri = mapped
}
/**
* @param value Gets or sets the db2 database sql port.
*/
@JvmName("jwmkvnubtnfarpcc")
public suspend fun dbPort(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbPort = mapped
}
/**
* @param value Gets or sets the db2 database user name.
*/
@JvmName("fdqvqgagkbqkrrux")
public suspend fun dbUsername(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbUsername = mapped
}
/**
* @param value Gets or sets the target virtual machine name.
*/
@JvmName("bnytcuemwyichxfo")
public suspend fun hostname(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hostname = mapped
}
/**
* @param value The provider type. For example, the value can be SapHana.
* Expected value is 'Db2'.
*/
@JvmName("jtogecqeadylccbs")
public suspend fun providerType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.providerType = mapped
}
/**
* @param value Gets or sets the SAP System Identifier
*/
@JvmName("poiispwqeccllfbj")
public suspend fun sapSid(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sapSid = mapped
}
/**
* @param value Gets or sets the blob URI to SSL certificate for the DB2 Database.
*/
@JvmName("mgrcnkgomcxcuigj")
public suspend fun sslCertificateUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sslCertificateUri = mapped
}
/**
* @param value Gets or sets certificate preference if secure communication is enabled.
*/
@JvmName("ulrvhfivopxfbiiq")
public suspend fun sslPreference(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sslPreference = mapped
}
/**
* @param value Gets or sets certificate preference if secure communication is enabled.
*/
@JvmName("cebnsehknntndfxp")
public fun sslPreference(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sslPreference = mapped
}
/**
* @param value Gets or sets certificate preference if secure communication is enabled.
*/
@JvmName("yhkyrhymyquauycs")
public fun sslPreference(`value`: SslPreference) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sslPreference = mapped
}
internal fun build(): DB2ProviderInstancePropertiesArgs = DB2ProviderInstancePropertiesArgs(
dbName = dbName,
dbPassword = dbPassword,
dbPasswordUri = dbPasswordUri,
dbPort = dbPort,
dbUsername = dbUsername,
hostname = hostname,
providerType = providerType ?: throw PulumiNullFieldException("providerType"),
sapSid = sapSid,
sslCertificateUri = sslCertificateUri,
sslPreference = sslPreference,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy