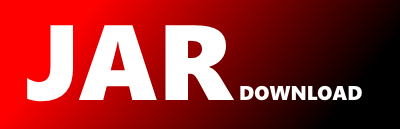
com.pulumi.azurenative.workloads.kotlin.inputs.DBBackupPolicyPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.workloads.kotlin.inputs
import com.pulumi.azurenative.workloads.inputs.DBBackupPolicyPropertiesArgs.builder
import com.pulumi.azurenative.workloads.kotlin.enums.WorkloadType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Defines the policy properties for database backup.
* @property backupManagementType This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureWorkload'.
* @property makePolicyConsistent Fix the policy inconsistency
* @property name The name of the DB backup policy.
* @property protectedItemsCount Number of items associated with this policy.
* @property resourceGuardOperationRequests ResourceGuard Operation Requests
* @property settings Common settings for the backup management
* @property subProtectionPolicy List of sub-protection policies which includes schedule and retention
* @property workLoadType Type of workload for the backup management
*/
public data class DBBackupPolicyPropertiesArgs(
public val backupManagementType: Output,
public val makePolicyConsistent: Output? = null,
public val name: Output,
public val protectedItemsCount: Output? = null,
public val resourceGuardOperationRequests: Output>? = null,
public val settings: Output? = null,
public val subProtectionPolicy: Output>? = null,
public val workLoadType: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.workloads.inputs.DBBackupPolicyPropertiesArgs =
com.pulumi.azurenative.workloads.inputs.DBBackupPolicyPropertiesArgs.builder()
.backupManagementType(backupManagementType.applyValue({ args0 -> args0 }))
.makePolicyConsistent(makePolicyConsistent?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.protectedItemsCount(protectedItemsCount?.applyValue({ args0 -> args0 }))
.resourceGuardOperationRequests(
resourceGuardOperationRequests?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.settings(settings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.subProtectionPolicy(
subProtectionPolicy?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.workLoadType(
workLoadType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [DBBackupPolicyPropertiesArgs].
*/
@PulumiTagMarker
public class DBBackupPolicyPropertiesArgsBuilder internal constructor() {
private var backupManagementType: Output? = null
private var makePolicyConsistent: Output? = null
private var name: Output? = null
private var protectedItemsCount: Output? = null
private var resourceGuardOperationRequests: Output>? = null
private var settings: Output? = null
private var subProtectionPolicy: Output>? = null
private var workLoadType: Output>? = null
/**
* @param value This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureWorkload'.
*/
@JvmName("bkthbptqipkenruf")
public suspend fun backupManagementType(`value`: Output) {
this.backupManagementType = value
}
/**
* @param value Fix the policy inconsistency
*/
@JvmName("plmrurugcbmrokby")
public suspend fun makePolicyConsistent(`value`: Output) {
this.makePolicyConsistent = value
}
/**
* @param value The name of the DB backup policy.
*/
@JvmName("igypqkiqwnbrdfuw")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Number of items associated with this policy.
*/
@JvmName("xasgpaojklxvsbmn")
public suspend fun protectedItemsCount(`value`: Output) {
this.protectedItemsCount = value
}
/**
* @param value ResourceGuard Operation Requests
*/
@JvmName("fsopgbrciqqkndga")
public suspend fun resourceGuardOperationRequests(`value`: Output>) {
this.resourceGuardOperationRequests = value
}
@JvmName("qhtrijcnajiuitad")
public suspend fun resourceGuardOperationRequests(vararg values: Output) {
this.resourceGuardOperationRequests = Output.all(values.asList())
}
/**
* @param values ResourceGuard Operation Requests
*/
@JvmName("ebfxlmnbniotikbb")
public suspend fun resourceGuardOperationRequests(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy