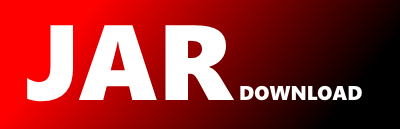
com.pulumi.azurenative.workloads.kotlin.inputs.SimpleSchedulePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.workloads.kotlin.inputs
import com.pulumi.azurenative.workloads.inputs.SimpleSchedulePolicyArgs.builder
import com.pulumi.azurenative.workloads.kotlin.enums.DayOfWeek
import com.pulumi.azurenative.workloads.kotlin.enums.ScheduleRunType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Simple policy schedule.
* @property hourlySchedule Hourly Schedule of this Policy
* @property schedulePolicyType This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'SimpleSchedulePolicy'.
* @property scheduleRunDays List of days of week this schedule has to be run.
* @property scheduleRunFrequency Frequency of the schedule operation of this policy.
* @property scheduleRunTimes List of times of day this schedule has to be run.
* @property scheduleWeeklyFrequency At every number weeks this schedule has to be run.
*/
public data class SimpleSchedulePolicyArgs(
public val hourlySchedule: Output? = null,
public val schedulePolicyType: Output,
public val scheduleRunDays: Output>? = null,
public val scheduleRunFrequency: Output>? = null,
public val scheduleRunTimes: Output>? = null,
public val scheduleWeeklyFrequency: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.workloads.inputs.SimpleSchedulePolicyArgs =
com.pulumi.azurenative.workloads.inputs.SimpleSchedulePolicyArgs.builder()
.hourlySchedule(hourlySchedule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.schedulePolicyType(schedulePolicyType.applyValue({ args0 -> args0 }))
.scheduleRunDays(
scheduleRunDays?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.scheduleRunFrequency(
scheduleRunFrequency?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.scheduleRunTimes(scheduleRunTimes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.scheduleWeeklyFrequency(scheduleWeeklyFrequency?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SimpleSchedulePolicyArgs].
*/
@PulumiTagMarker
public class SimpleSchedulePolicyArgsBuilder internal constructor() {
private var hourlySchedule: Output? = null
private var schedulePolicyType: Output? = null
private var scheduleRunDays: Output>? = null
private var scheduleRunFrequency: Output>? = null
private var scheduleRunTimes: Output>? = null
private var scheduleWeeklyFrequency: Output? = null
/**
* @param value Hourly Schedule of this Policy
*/
@JvmName("utntganihnojbldh")
public suspend fun hourlySchedule(`value`: Output) {
this.hourlySchedule = value
}
/**
* @param value This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'SimpleSchedulePolicy'.
*/
@JvmName("dglnxabarkqttrqj")
public suspend fun schedulePolicyType(`value`: Output) {
this.schedulePolicyType = value
}
/**
* @param value List of days of week this schedule has to be run.
*/
@JvmName("rxmnpkjodehaujua")
public suspend fun scheduleRunDays(`value`: Output>) {
this.scheduleRunDays = value
}
@JvmName("ppqrgvgukiciurlf")
public suspend fun scheduleRunDays(vararg values: Output) {
this.scheduleRunDays = Output.all(values.asList())
}
/**
* @param values List of days of week this schedule has to be run.
*/
@JvmName("xbqsvftxblcrpapl")
public suspend fun scheduleRunDays(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy