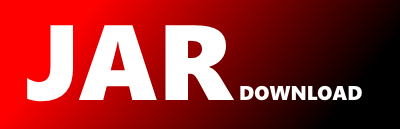
com.pulumi.azurenative.workloads.kotlin.inputs.ThreeTierConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.workloads.kotlin.inputs
import com.pulumi.azurenative.workloads.inputs.ThreeTierConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Gets or sets the three tier SAP configuration. For prerequisites for creating the infrastructure, please see [here](https://go.microsoft.com/fwlink/?linkid=2212611&clcid=0x409)
* @property appResourceGroup The application resource group where SAP system resources will be deployed.
* @property applicationServer The application server configuration.
* @property centralServer The central server configuration.
* @property customResourceNames The set of custom names to be used for underlying azure resources that are part of the SAP system.
* @property databaseServer The database configuration.
* @property deploymentType The type of SAP deployment, single server or Three tier.
* Expected value is 'ThreeTier'.
* @property highAvailabilityConfig The high availability configuration.
* @property networkConfiguration Network configuration common to all servers
* @property storageConfiguration The storage configuration.
*/
public data class ThreeTierConfigurationArgs(
public val appResourceGroup: Output,
public val applicationServer: Output,
public val centralServer: Output,
public val customResourceNames: Output? = null,
public val databaseServer: Output,
public val deploymentType: Output,
public val highAvailabilityConfig: Output? = null,
public val networkConfiguration: Output? = null,
public val storageConfiguration: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.workloads.inputs.ThreeTierConfigurationArgs =
com.pulumi.azurenative.workloads.inputs.ThreeTierConfigurationArgs.builder()
.appResourceGroup(appResourceGroup.applyValue({ args0 -> args0 }))
.applicationServer(applicationServer.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.centralServer(centralServer.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.customResourceNames(
customResourceNames?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.databaseServer(databaseServer.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.deploymentType(deploymentType.applyValue({ args0 -> args0 }))
.highAvailabilityConfig(
highAvailabilityConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.networkConfiguration(
networkConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.storageConfiguration(
storageConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ThreeTierConfigurationArgs].
*/
@PulumiTagMarker
public class ThreeTierConfigurationArgsBuilder internal constructor() {
private var appResourceGroup: Output? = null
private var applicationServer: Output? = null
private var centralServer: Output? = null
private var customResourceNames: Output? = null
private var databaseServer: Output? = null
private var deploymentType: Output? = null
private var highAvailabilityConfig: Output? = null
private var networkConfiguration: Output? = null
private var storageConfiguration: Output? = null
/**
* @param value The application resource group where SAP system resources will be deployed.
*/
@JvmName("nmvlmxjnxiipfahd")
public suspend fun appResourceGroup(`value`: Output) {
this.appResourceGroup = value
}
/**
* @param value The application server configuration.
*/
@JvmName("uqhsbckydgqeheqf")
public suspend fun applicationServer(`value`: Output) {
this.applicationServer = value
}
/**
* @param value The central server configuration.
*/
@JvmName("qxoigjfrlfbyuhpm")
public suspend fun centralServer(`value`: Output) {
this.centralServer = value
}
/**
* @param value The set of custom names to be used for underlying azure resources that are part of the SAP system.
*/
@JvmName("feildtfqsluinlaw")
public suspend fun customResourceNames(`value`: Output) {
this.customResourceNames = value
}
/**
* @param value The database configuration.
*/
@JvmName("jpyfvyqnurulejdr")
public suspend fun databaseServer(`value`: Output) {
this.databaseServer = value
}
/**
* @param value The type of SAP deployment, single server or Three tier.
* Expected value is 'ThreeTier'.
*/
@JvmName("xsfgfwnpoytjqyyg")
public suspend fun deploymentType(`value`: Output) {
this.deploymentType = value
}
/**
* @param value The high availability configuration.
*/
@JvmName("ceypjtnmsaktprrb")
public suspend fun highAvailabilityConfig(`value`: Output) {
this.highAvailabilityConfig = value
}
/**
* @param value Network configuration common to all servers
*/
@JvmName("oeecjooppmspnioi")
public suspend fun networkConfiguration(`value`: Output) {
this.networkConfiguration = value
}
/**
* @param value The storage configuration.
*/
@JvmName("tbhuwqvswbsfgifn")
public suspend fun storageConfiguration(`value`: Output) {
this.storageConfiguration = value
}
/**
* @param value The application resource group where SAP system resources will be deployed.
*/
@JvmName("mjsragilgdlakfxx")
public suspend fun appResourceGroup(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.appResourceGroup = mapped
}
/**
* @param value The application server configuration.
*/
@JvmName("wckkylpsiabkvvgb")
public suspend fun applicationServer(`value`: ApplicationServerConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.applicationServer = mapped
}
/**
* @param argument The application server configuration.
*/
@JvmName("llvawektsraxkjrm")
public suspend fun applicationServer(argument: suspend ApplicationServerConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = ApplicationServerConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.applicationServer = mapped
}
/**
* @param value The central server configuration.
*/
@JvmName("ycxyckcpipylbhnv")
public suspend fun centralServer(`value`: CentralServerConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.centralServer = mapped
}
/**
* @param argument The central server configuration.
*/
@JvmName("aobbcaffrwqrvise")
public suspend fun centralServer(argument: suspend CentralServerConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = CentralServerConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.centralServer = mapped
}
/**
* @param value The set of custom names to be used for underlying azure resources that are part of the SAP system.
*/
@JvmName("hsjjfcgxrvnwdpdk")
public suspend fun customResourceNames(`value`: ThreeTierFullResourceNamesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customResourceNames = mapped
}
/**
* @param argument The set of custom names to be used for underlying azure resources that are part of the SAP system.
*/
@JvmName("kbvlrbnwgsxupggv")
public suspend fun customResourceNames(argument: suspend ThreeTierFullResourceNamesArgsBuilder.() -> Unit) {
val toBeMapped = ThreeTierFullResourceNamesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.customResourceNames = mapped
}
/**
* @param value The database configuration.
*/
@JvmName("hkfawwwdmqvwpkpw")
public suspend fun databaseServer(`value`: DatabaseConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.databaseServer = mapped
}
/**
* @param argument The database configuration.
*/
@JvmName("ycerlgnuhxcuvnuj")
public suspend fun databaseServer(argument: suspend DatabaseConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DatabaseConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.databaseServer = mapped
}
/**
* @param value The type of SAP deployment, single server or Three tier.
* Expected value is 'ThreeTier'.
*/
@JvmName("haxqchivhijhmnsb")
public suspend fun deploymentType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.deploymentType = mapped
}
/**
* @param value The high availability configuration.
*/
@JvmName("uksfiuxdjnovnjpl")
public suspend fun highAvailabilityConfig(`value`: HighAvailabilityConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.highAvailabilityConfig = mapped
}
/**
* @param argument The high availability configuration.
*/
@JvmName("oxhxrrihtahpdnna")
public suspend fun highAvailabilityConfig(argument: suspend HighAvailabilityConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = HighAvailabilityConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.highAvailabilityConfig = mapped
}
/**
* @param value Network configuration common to all servers
*/
@JvmName("fwhwqvmorlnijuch")
public suspend fun networkConfiguration(`value`: NetworkConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.networkConfiguration = mapped
}
/**
* @param argument Network configuration common to all servers
*/
@JvmName("uqgaisyqmrwxovlg")
public suspend fun networkConfiguration(argument: suspend NetworkConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = NetworkConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.networkConfiguration = mapped
}
/**
* @param value The storage configuration.
*/
@JvmName("jxcvkjonbwlbpcoa")
public suspend fun storageConfiguration(`value`: StorageConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageConfiguration = mapped
}
/**
* @param argument The storage configuration.
*/
@JvmName("gfxymvesslnjnqkl")
public suspend fun storageConfiguration(argument: suspend StorageConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = StorageConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.storageConfiguration = mapped
}
internal fun build(): ThreeTierConfigurationArgs = ThreeTierConfigurationArgs(
appResourceGroup = appResourceGroup ?: throw PulumiNullFieldException("appResourceGroup"),
applicationServer = applicationServer ?: throw PulumiNullFieldException("applicationServer"),
centralServer = centralServer ?: throw PulumiNullFieldException("centralServer"),
customResourceNames = customResourceNames,
databaseServer = databaseServer ?: throw PulumiNullFieldException("databaseServer"),
deploymentType = deploymentType ?: throw PulumiNullFieldException("deploymentType"),
highAvailabilityConfig = highAvailabilityConfig,
networkConfiguration = networkConfiguration,
storageConfiguration = storageConfiguration,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy