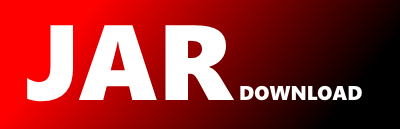
com.pulumi.azurenative.workloads.kotlin.inputs.TieringPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.workloads.kotlin.inputs
import com.pulumi.azurenative.workloads.inputs.TieringPolicyArgs.builder
import com.pulumi.azurenative.workloads.kotlin.enums.RetentionDurationType
import com.pulumi.azurenative.workloads.kotlin.enums.TieringMode
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Tiering Policy for a target tier.
* If the policy is not specified for a given target tier, service retains the existing configured tiering policy for that tier
* @property duration Number of days/weeks/months/years to retain backups in current tier before tiering.
* Used only if TieringMode is set to TierAfter
* @property durationType Retention duration type: days/weeks/months/years
* Used only if TieringMode is set to TierAfter
* @property tieringMode Tiering Mode to control automatic tiering of recovery points. Supported values are:
* 1. TierRecommended: Tier all recovery points recommended to be tiered
* 2. TierAfter: Tier all recovery points after a fixed period, as specified in duration + durationType below.
* 3. DoNotTier: Do not tier any recovery points
*/
public data class TieringPolicyArgs(
public val duration: Output? = null,
public val durationType: Output>? = null,
public val tieringMode: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.workloads.inputs.TieringPolicyArgs =
com.pulumi.azurenative.workloads.inputs.TieringPolicyArgs.builder()
.duration(duration?.applyValue({ args0 -> args0 }))
.durationType(
durationType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.tieringMode(
tieringMode?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
).build()
}
/**
* Builder for [TieringPolicyArgs].
*/
@PulumiTagMarker
public class TieringPolicyArgsBuilder internal constructor() {
private var duration: Output? = null
private var durationType: Output>? = null
private var tieringMode: Output>? = null
/**
* @param value Number of days/weeks/months/years to retain backups in current tier before tiering.
* Used only if TieringMode is set to TierAfter
*/
@JvmName("hrjbvoqvfimfmdnf")
public suspend fun duration(`value`: Output) {
this.duration = value
}
/**
* @param value Retention duration type: days/weeks/months/years
* Used only if TieringMode is set to TierAfter
*/
@JvmName("isffibshokjfrsty")
public suspend fun durationType(`value`: Output>) {
this.durationType = value
}
/**
* @param value Tiering Mode to control automatic tiering of recovery points. Supported values are:
* 1. TierRecommended: Tier all recovery points recommended to be tiered
* 2. TierAfter: Tier all recovery points after a fixed period, as specified in duration + durationType below.
* 3. DoNotTier: Do not tier any recovery points
*/
@JvmName("krwbcktrmdpqhequ")
public suspend fun tieringMode(`value`: Output>) {
this.tieringMode = value
}
/**
* @param value Number of days/weeks/months/years to retain backups in current tier before tiering.
* Used only if TieringMode is set to TierAfter
*/
@JvmName("kglttvixyrcehpcm")
public suspend fun duration(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.duration = mapped
}
/**
* @param value Retention duration type: days/weeks/months/years
* Used only if TieringMode is set to TierAfter
*/
@JvmName("qaulrnotidyfrwnk")
public suspend fun durationType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.durationType = mapped
}
/**
* @param value Retention duration type: days/weeks/months/years
* Used only if TieringMode is set to TierAfter
*/
@JvmName("eaakuatgpfitybog")
public fun durationType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.durationType = mapped
}
/**
* @param value Retention duration type: days/weeks/months/years
* Used only if TieringMode is set to TierAfter
*/
@JvmName("crvolpvjymtvuuua")
public fun durationType(`value`: RetentionDurationType) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.durationType = mapped
}
/**
* @param value Tiering Mode to control automatic tiering of recovery points. Supported values are:
* 1. TierRecommended: Tier all recovery points recommended to be tiered
* 2. TierAfter: Tier all recovery points after a fixed period, as specified in duration + durationType below.
* 3. DoNotTier: Do not tier any recovery points
*/
@JvmName("voqunmcqfbdaosvr")
public suspend fun tieringMode(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tieringMode = mapped
}
/**
* @param value Tiering Mode to control automatic tiering of recovery points. Supported values are:
* 1. TierRecommended: Tier all recovery points recommended to be tiered
* 2. TierAfter: Tier all recovery points after a fixed period, as specified in duration + durationType below.
* 3. DoNotTier: Do not tier any recovery points
*/
@JvmName("wccrilnsqgheewva")
public fun tieringMode(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tieringMode = mapped
}
/**
* @param value Tiering Mode to control automatic tiering of recovery points. Supported values are:
* 1. TierRecommended: Tier all recovery points recommended to be tiered
* 2. TierAfter: Tier all recovery points after a fixed period, as specified in duration + durationType below.
* 3. DoNotTier: Do not tier any recovery points
*/
@JvmName("hjxibespioaxxhww")
public fun tieringMode(`value`: TieringMode) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tieringMode = mapped
}
internal fun build(): TieringPolicyArgs = TieringPolicyArgs(
duration = duration,
durationType = durationType,
tieringMode = tieringMode,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy