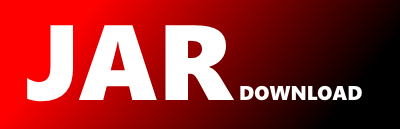
com.pulumi.cloudflare.kotlin.LoadBalancerMonitorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-cloudflare-kotlin Show documentation
Show all versions of pulumi-cloudflare-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.cloudflare.kotlin
import com.pulumi.cloudflare.LoadBalancerMonitorArgs.builder
import com.pulumi.cloudflare.kotlin.inputs.LoadBalancerMonitorHeaderArgs
import com.pulumi.cloudflare.kotlin.inputs.LoadBalancerMonitorHeaderArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* If Cloudflare's Load Balancing to load-balance across multiple
* origin servers or data centers, you configure one of these Monitors
* to actively check the availability of those servers over HTTP(S) or
* TCP.
* ## Import
* ```sh
* $ pulumi import cloudflare:index/loadBalancerMonitor:LoadBalancerMonitor example /
* ```
* @property accountId The account identifier to target for the resource.
* @property allowInsecure Do not validate the certificate when monitor use HTTPS. Only valid if `type` is "http" or "https".
* @property consecutiveDown To be marked unhealthy the monitored origin must fail this healthcheck N consecutive times. Defaults to `0`.
* @property consecutiveUp To be marked healthy the monitored origin must pass this healthcheck N consecutive times. Defaults to `0`.
* @property description Free text description.
* @property expectedBody A case-insensitive sub-string to look for in the response body. If this string is not found, the origin will be marked as unhealthy. Only valid if `type` is "http" or "https".
* @property expectedCodes The expected HTTP response code or code range of the health check. Eg `2xx`. Only valid and required if `type` is "http" or "https".
* @property followRedirects Follow redirects if returned by the origin. Only valid if `type` is "http" or "https".
* @property headers The HTTP request headers to send in the health check. It is recommended you set a Host header by default. The User-Agent header cannot be overridden.
* @property interval The interval between each health check. Shorter intervals may improve failover time, but will increase load on the origins as we check from multiple locations. Defaults to `60`.
* @property method The method to use for the health check.
* @property path The endpoint path to health check against.
* @property port The port number to use for the healthcheck, required when creating a TCP monitor.
* @property probeZone Assign this monitor to emulate the specified zone while probing. Only valid if `type` is "http" or "https".
* @property retries The number of retries to attempt in case of a timeout before marking the origin as unhealthy. Retries are attempted immediately. Defaults to `2`.
* @property timeout The timeout (in seconds) before marking the health check as failed. Defaults to `5`.
* @property type The protocol to use for the healthcheck. Available values: `http`, `https`, `tcp`, `udp_icmp`, `icmp_ping`, `smtp`. Defaults to `http`.
*/
public data class LoadBalancerMonitorArgs(
public val accountId: Output? = null,
public val allowInsecure: Output? = null,
public val consecutiveDown: Output? = null,
public val consecutiveUp: Output? = null,
public val description: Output? = null,
public val expectedBody: Output? = null,
public val expectedCodes: Output? = null,
public val followRedirects: Output? = null,
public val headers: Output>? = null,
public val interval: Output? = null,
public val method: Output? = null,
public val path: Output? = null,
public val port: Output? = null,
public val probeZone: Output? = null,
public val retries: Output? = null,
public val timeout: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.cloudflare.LoadBalancerMonitorArgs =
com.pulumi.cloudflare.LoadBalancerMonitorArgs.builder()
.accountId(accountId?.applyValue({ args0 -> args0 }))
.allowInsecure(allowInsecure?.applyValue({ args0 -> args0 }))
.consecutiveDown(consecutiveDown?.applyValue({ args0 -> args0 }))
.consecutiveUp(consecutiveUp?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.expectedBody(expectedBody?.applyValue({ args0 -> args0 }))
.expectedCodes(expectedCodes?.applyValue({ args0 -> args0 }))
.followRedirects(followRedirects?.applyValue({ args0 -> args0 }))
.headers(
headers?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.interval(interval?.applyValue({ args0 -> args0 }))
.method(method?.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.port(port?.applyValue({ args0 -> args0 }))
.probeZone(probeZone?.applyValue({ args0 -> args0 }))
.retries(retries?.applyValue({ args0 -> args0 }))
.timeout(timeout?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LoadBalancerMonitorArgs].
*/
@PulumiTagMarker
public class LoadBalancerMonitorArgsBuilder internal constructor() {
private var accountId: Output? = null
private var allowInsecure: Output? = null
private var consecutiveDown: Output? = null
private var consecutiveUp: Output? = null
private var description: Output? = null
private var expectedBody: Output? = null
private var expectedCodes: Output? = null
private var followRedirects: Output? = null
private var headers: Output>? = null
private var interval: Output? = null
private var method: Output? = null
private var path: Output? = null
private var port: Output? = null
private var probeZone: Output? = null
private var retries: Output? = null
private var timeout: Output? = null
private var type: Output? = null
/**
* @param value The account identifier to target for the resource.
*/
@JvmName("gleqwmansyhikbmb")
public suspend fun accountId(`value`: Output) {
this.accountId = value
}
/**
* @param value Do not validate the certificate when monitor use HTTPS. Only valid if `type` is "http" or "https".
*/
@JvmName("cqtxrgpicuxsjwbw")
public suspend fun allowInsecure(`value`: Output) {
this.allowInsecure = value
}
/**
* @param value To be marked unhealthy the monitored origin must fail this healthcheck N consecutive times. Defaults to `0`.
*/
@JvmName("lyndjxxfkbbklllx")
public suspend fun consecutiveDown(`value`: Output) {
this.consecutiveDown = value
}
/**
* @param value To be marked healthy the monitored origin must pass this healthcheck N consecutive times. Defaults to `0`.
*/
@JvmName("bcpnuwfspocwefoo")
public suspend fun consecutiveUp(`value`: Output) {
this.consecutiveUp = value
}
/**
* @param value Free text description.
*/
@JvmName("mkqfyiumvkiuttne")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value A case-insensitive sub-string to look for in the response body. If this string is not found, the origin will be marked as unhealthy. Only valid if `type` is "http" or "https".
*/
@JvmName("wexjoichbrkljrhy")
public suspend fun expectedBody(`value`: Output) {
this.expectedBody = value
}
/**
* @param value The expected HTTP response code or code range of the health check. Eg `2xx`. Only valid and required if `type` is "http" or "https".
*/
@JvmName("lxxwvdwnpvkswuou")
public suspend fun expectedCodes(`value`: Output) {
this.expectedCodes = value
}
/**
* @param value Follow redirects if returned by the origin. Only valid if `type` is "http" or "https".
*/
@JvmName("jylmiiukxldaiunt")
public suspend fun followRedirects(`value`: Output) {
this.followRedirects = value
}
/**
* @param value The HTTP request headers to send in the health check. It is recommended you set a Host header by default. The User-Agent header cannot be overridden.
*/
@JvmName("jaesnougvcbcalmt")
public suspend fun headers(`value`: Output>) {
this.headers = value
}
@JvmName("mkjwkjftgjxfxxtm")
public suspend fun headers(vararg values: Output) {
this.headers = Output.all(values.asList())
}
/**
* @param values The HTTP request headers to send in the health check. It is recommended you set a Host header by default. The User-Agent header cannot be overridden.
*/
@JvmName("cyjreiuaqoildafl")
public suspend fun headers(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy