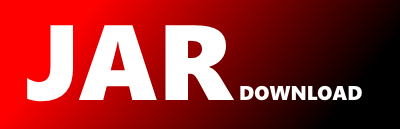
com.pulumi.cloudflare.kotlin.RulesetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-cloudflare-kotlin Show documentation
Show all versions of pulumi-cloudflare-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.cloudflare.kotlin
import com.pulumi.cloudflare.RulesetArgs.builder
import com.pulumi.cloudflare.kotlin.inputs.RulesetRuleArgs
import com.pulumi.cloudflare.kotlin.inputs.RulesetRuleArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The [Cloudflare Ruleset Engine](https://developers.cloudflare.com/firewall/cf-rulesets)
* allows you to create and deploy rules and rulesets.
* The engine syntax, inspired by the Wireshark Display Filter language, is the
* same syntax used in custom Firewall Rules. Cloudflare uses the Ruleset Engine
* in different products, allowing you to configure several products using the same
* basic syntax.
* ## Import
* Import an account scoped Ruleset configuration.
* ```sh
* $ pulumi import cloudflare:index/ruleset:Ruleset example account//
* ```
* Import a zone scoped Ruleset configuration.
* ```sh
* $ pulumi import cloudflare:index/ruleset:Ruleset example zone//
* ```
* @property accountId The account identifier to target for the resource.
* @property description Brief summary of the ruleset and its intended use.
* @property kind Type of Ruleset to create. Available values: `custom`, `managed`, `root`, `zone`.
* @property name Name of the ruleset.
* @property phase Point in the request/response lifecycle where the ruleset will be created. Available values: `ddos_l4`, `ddos_l7`, `http_config_settings`, `http_custom_errors`, `http_log_custom_fields`, `http_ratelimit`, `http_request_cache_settings`, `http_request_dynamic_redirect`, `http_request_firewall_custom`, `http_request_firewall_managed`, `http_request_late_transform`, `http_request_origin`, `http_request_redirect`, `http_request_sanitize`, `http_request_sbfm`, `http_request_transform`, `http_response_compression`, `http_response_firewall_managed`, `http_response_headers_transform`, `magic_transit`.
* @property rules List of rules to apply to the ruleset.
* @property zoneId The zone identifier to target for the resource.
*/
public data class RulesetArgs(
public val accountId: Output? = null,
public val description: Output? = null,
public val kind: Output? = null,
public val name: Output? = null,
public val phase: Output? = null,
public val rules: Output>? = null,
public val zoneId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.cloudflare.RulesetArgs =
com.pulumi.cloudflare.RulesetArgs.builder()
.accountId(accountId?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.phase(phase?.applyValue({ args0 -> args0 }))
.rules(rules?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.zoneId(zoneId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RulesetArgs].
*/
@PulumiTagMarker
public class RulesetArgsBuilder internal constructor() {
private var accountId: Output? = null
private var description: Output? = null
private var kind: Output? = null
private var name: Output? = null
private var phase: Output? = null
private var rules: Output>? = null
private var zoneId: Output? = null
/**
* @param value The account identifier to target for the resource.
*/
@JvmName("dkchjjkbiddnrcln")
public suspend fun accountId(`value`: Output) {
this.accountId = value
}
/**
* @param value Brief summary of the ruleset and its intended use.
*/
@JvmName("uptdkaqbeqxevaiw")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Type of Ruleset to create. Available values: `custom`, `managed`, `root`, `zone`.
*/
@JvmName("ubslapoaqgnkteah")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Name of the ruleset.
*/
@JvmName("yniagxmvjcctvpvi")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Point in the request/response lifecycle where the ruleset will be created. Available values: `ddos_l4`, `ddos_l7`, `http_config_settings`, `http_custom_errors`, `http_log_custom_fields`, `http_ratelimit`, `http_request_cache_settings`, `http_request_dynamic_redirect`, `http_request_firewall_custom`, `http_request_firewall_managed`, `http_request_late_transform`, `http_request_origin`, `http_request_redirect`, `http_request_sanitize`, `http_request_sbfm`, `http_request_transform`, `http_response_compression`, `http_response_firewall_managed`, `http_response_headers_transform`, `magic_transit`.
*/
@JvmName("pjmigauhpeheprvj")
public suspend fun phase(`value`: Output) {
this.phase = value
}
/**
* @param value List of rules to apply to the ruleset.
*/
@JvmName("lniumqoiypqskeck")
public suspend fun rules(`value`: Output>) {
this.rules = value
}
@JvmName("tgghfbflopkmjyjf")
public suspend fun rules(vararg values: Output) {
this.rules = Output.all(values.asList())
}
/**
* @param values List of rules to apply to the ruleset.
*/
@JvmName("ejfuxqttuepheemw")
public suspend fun rules(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy