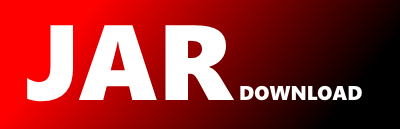
com.pulumi.cloudflare.kotlin.inputs.AccessApplicationLandingPageDesignArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-cloudflare-kotlin Show documentation
Show all versions of pulumi-cloudflare-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.cloudflare.kotlin.inputs
import com.pulumi.cloudflare.inputs.AccessApplicationLandingPageDesignArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property buttonColor The button color of the landing page.
* @property buttonTextColor The button text color of the landing page.
* @property imageUrl The URL of the image to be displayed in the landing page.
* @property message The message of the landing page.
* @property title The title of the landing page.
*/
public data class AccessApplicationLandingPageDesignArgs(
public val buttonColor: Output? = null,
public val buttonTextColor: Output? = null,
public val imageUrl: Output? = null,
public val message: Output? = null,
public val title: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.cloudflare.inputs.AccessApplicationLandingPageDesignArgs =
com.pulumi.cloudflare.inputs.AccessApplicationLandingPageDesignArgs.builder()
.buttonColor(buttonColor?.applyValue({ args0 -> args0 }))
.buttonTextColor(buttonTextColor?.applyValue({ args0 -> args0 }))
.imageUrl(imageUrl?.applyValue({ args0 -> args0 }))
.message(message?.applyValue({ args0 -> args0 }))
.title(title?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AccessApplicationLandingPageDesignArgs].
*/
@PulumiTagMarker
public class AccessApplicationLandingPageDesignArgsBuilder internal constructor() {
private var buttonColor: Output? = null
private var buttonTextColor: Output? = null
private var imageUrl: Output? = null
private var message: Output? = null
private var title: Output? = null
/**
* @param value The button color of the landing page.
*/
@JvmName("ktomekjsrkcyeftw")
public suspend fun buttonColor(`value`: Output) {
this.buttonColor = value
}
/**
* @param value The button text color of the landing page.
*/
@JvmName("osrdfnsvousiogdf")
public suspend fun buttonTextColor(`value`: Output) {
this.buttonTextColor = value
}
/**
* @param value The URL of the image to be displayed in the landing page.
*/
@JvmName("rearrvkflydpimyd")
public suspend fun imageUrl(`value`: Output) {
this.imageUrl = value
}
/**
* @param value The message of the landing page.
*/
@JvmName("ehkcpjqhqrvqqwxt")
public suspend fun message(`value`: Output) {
this.message = value
}
/**
* @param value The title of the landing page.
*/
@JvmName("qemexyqtcrvwtvve")
public suspend fun title(`value`: Output) {
this.title = value
}
/**
* @param value The button color of the landing page.
*/
@JvmName("lsgkxmtsklepdymp")
public suspend fun buttonColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buttonColor = mapped
}
/**
* @param value The button text color of the landing page.
*/
@JvmName("trxwapuicxoystyd")
public suspend fun buttonTextColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buttonTextColor = mapped
}
/**
* @param value The URL of the image to be displayed in the landing page.
*/
@JvmName("oxvpgigihfopnmef")
public suspend fun imageUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageUrl = mapped
}
/**
* @param value The message of the landing page.
*/
@JvmName("ofvxfglllhwpevww")
public suspend fun message(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.message = mapped
}
/**
* @param value The title of the landing page.
*/
@JvmName("bnrjucnstvwnqfru")
public suspend fun title(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.title = mapped
}
internal fun build(): AccessApplicationLandingPageDesignArgs =
AccessApplicationLandingPageDesignArgs(
buttonColor = buttonColor,
buttonTextColor = buttonTextColor,
imageUrl = imageUrl,
message = message,
title = title,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy