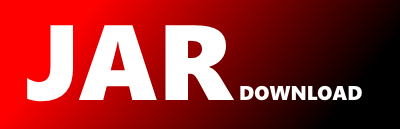
com.pulumi.cloudflare.kotlin.inputs.GetInfrastructureAccessTargetsPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-cloudflare-kotlin Show documentation
Show all versions of pulumi-cloudflare-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.cloudflare.kotlin.inputs
import com.pulumi.cloudflare.inputs.GetInfrastructureAccessTargetsPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getInfrastructureAccessTargets.
* @property accountId The account identifier to target for the resource.
* @property createdAfter A date and time after a target was created to filter on.
* @property hostname The name of the app type.
* @property hostnameContains The name of the app type.
* @property ipv4 The name of the app type.
* @property ipv6 The name of the app type.
* @property modifiedAfter A date and time after a target was modified to filter on.
* @property virtualNetworkId The name of the app type.
*/
public data class GetInfrastructureAccessTargetsPlainArgs(
public val accountId: String,
public val createdAfter: String? = null,
public val hostname: String? = null,
public val hostnameContains: String? = null,
public val ipv4: String? = null,
public val ipv6: String? = null,
public val modifiedAfter: String? = null,
public val virtualNetworkId: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.cloudflare.inputs.GetInfrastructureAccessTargetsPlainArgs =
com.pulumi.cloudflare.inputs.GetInfrastructureAccessTargetsPlainArgs.builder()
.accountId(accountId.let({ args0 -> args0 }))
.createdAfter(createdAfter?.let({ args0 -> args0 }))
.hostname(hostname?.let({ args0 -> args0 }))
.hostnameContains(hostnameContains?.let({ args0 -> args0 }))
.ipv4(ipv4?.let({ args0 -> args0 }))
.ipv6(ipv6?.let({ args0 -> args0 }))
.modifiedAfter(modifiedAfter?.let({ args0 -> args0 }))
.virtualNetworkId(virtualNetworkId?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetInfrastructureAccessTargetsPlainArgs].
*/
@PulumiTagMarker
public class GetInfrastructureAccessTargetsPlainArgsBuilder internal constructor() {
private var accountId: String? = null
private var createdAfter: String? = null
private var hostname: String? = null
private var hostnameContains: String? = null
private var ipv4: String? = null
private var ipv6: String? = null
private var modifiedAfter: String? = null
private var virtualNetworkId: String? = null
/**
* @param value The account identifier to target for the resource.
*/
@JvmName("joiymlwovgdfoqbf")
public suspend fun accountId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.accountId = mapped
}
/**
* @param value A date and time after a target was created to filter on.
*/
@JvmName("gymhwwbqpakmxnat")
public suspend fun createdAfter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.createdAfter = mapped
}
/**
* @param value The name of the app type.
*/
@JvmName("fonbjfrdotdlhklo")
public suspend fun hostname(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.hostname = mapped
}
/**
* @param value The name of the app type.
*/
@JvmName("qhenqeguswqhhwbk")
public suspend fun hostnameContains(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.hostnameContains = mapped
}
/**
* @param value The name of the app type.
*/
@JvmName("ahkoxoqodtnxpnco")
public suspend fun ipv4(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.ipv4 = mapped
}
/**
* @param value The name of the app type.
*/
@JvmName("axuhkhhdbdgnhmwu")
public suspend fun ipv6(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.ipv6 = mapped
}
/**
* @param value A date and time after a target was modified to filter on.
*/
@JvmName("attmtxrppfydtekg")
public suspend fun modifiedAfter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.modifiedAfter = mapped
}
/**
* @param value The name of the app type.
*/
@JvmName("nfwrgjofxielxang")
public suspend fun virtualNetworkId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.virtualNetworkId = mapped
}
internal fun build(): GetInfrastructureAccessTargetsPlainArgs =
GetInfrastructureAccessTargetsPlainArgs(
accountId = accountId ?: throw PulumiNullFieldException("accountId"),
createdAfter = createdAfter,
hostname = hostname,
hostnameContains = hostnameContains,
ipv4 = ipv4,
ipv6 = ipv6,
modifiedAfter = modifiedAfter,
virtualNetworkId = virtualNetworkId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy