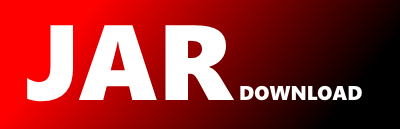
com.pulumi.cloudflare.kotlin.inputs.GetLoadBalancerPoolsPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-cloudflare-kotlin Show documentation
Show all versions of pulumi-cloudflare-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.cloudflare.kotlin.inputs
import com.pulumi.cloudflare.inputs.GetLoadBalancerPoolsPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getLoadBalancerPools.
* @property accountId The account identifier to target for the datasource lookups.
* @property filter One or more values used to look up Load Balancer pools. If more than one value is given all values must match in order to be included.
* @property pools A list of Load Balancer Pools details.
*/
public data class GetLoadBalancerPoolsPlainArgs(
public val accountId: String,
public val filter: GetLoadBalancerPoolsFilter? = null,
public val pools: List? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.cloudflare.inputs.GetLoadBalancerPoolsPlainArgs =
com.pulumi.cloudflare.inputs.GetLoadBalancerPoolsPlainArgs.builder()
.accountId(accountId.let({ args0 -> args0 }))
.filter(filter?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.pools(pools?.let({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) })).build()
}
/**
* Builder for [GetLoadBalancerPoolsPlainArgs].
*/
@PulumiTagMarker
public class GetLoadBalancerPoolsPlainArgsBuilder internal constructor() {
private var accountId: String? = null
private var filter: GetLoadBalancerPoolsFilter? = null
private var pools: List? = null
/**
* @param value The account identifier to target for the datasource lookups.
*/
@JvmName("ytmhpddrefaqlrje")
public suspend fun accountId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.accountId = mapped
}
/**
* @param value One or more values used to look up Load Balancer pools. If more than one value is given all values must match in order to be included.
*/
@JvmName("lpjidfgjjbtpwond")
public suspend fun filter(`value`: GetLoadBalancerPoolsFilter?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.filter = mapped
}
/**
* @param argument One or more values used to look up Load Balancer pools. If more than one value is given all values must match in order to be included.
*/
@JvmName("dgenrggbbeanquti")
public suspend fun filter(argument: suspend GetLoadBalancerPoolsFilterBuilder.() -> Unit) {
val toBeMapped = GetLoadBalancerPoolsFilterBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.filter = mapped
}
/**
* @param value A list of Load Balancer Pools details.
*/
@JvmName("gqgxyvrhmysjnckd")
public suspend fun pools(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.pools = mapped
}
/**
* @param argument A list of Load Balancer Pools details.
*/
@JvmName("eofrblesyeyahaxq")
public suspend fun pools(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetLoadBalancerPoolsPoolBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.pools = mapped
}
/**
* @param argument A list of Load Balancer Pools details.
*/
@JvmName("dyaymfwvdaajindc")
public suspend fun pools(vararg argument: suspend GetLoadBalancerPoolsPoolBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetLoadBalancerPoolsPoolBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.pools = mapped
}
/**
* @param argument A list of Load Balancer Pools details.
*/
@JvmName("tqxsxbuyaxfmehod")
public suspend fun pools(argument: suspend GetLoadBalancerPoolsPoolBuilder.() -> Unit) {
val toBeMapped = listOf(GetLoadBalancerPoolsPoolBuilder().applySuspend { argument() }.build())
val mapped = toBeMapped
this.pools = mapped
}
/**
* @param values A list of Load Balancer Pools details.
*/
@JvmName("bfxfdhrwklhcmgiw")
public suspend fun pools(vararg values: GetLoadBalancerPoolsPool) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.pools = mapped
}
internal fun build(): GetLoadBalancerPoolsPlainArgs = GetLoadBalancerPoolsPlainArgs(
accountId = accountId ?: throw PulumiNullFieldException("accountId"),
filter = filter,
pools = pools,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy