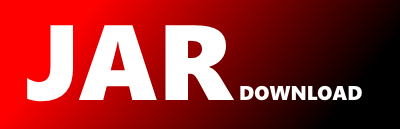
com.pulumi.cloudflare.kotlin.inputs.PagesProjectSourceConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-cloudflare-kotlin Show documentation
Show all versions of pulumi-cloudflare-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.cloudflare.kotlin.inputs
import com.pulumi.cloudflare.inputs.PagesProjectSourceConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property deploymentsEnabled Toggle deployments on this repo. Defaults to `true`.
* @property owner Project owner username. **Modifying this attribute will force creation of a new resource.**
* @property prCommentsEnabled Enable Pages to comment on Pull Requests. Defaults to `true`.
* @property previewBranchExcludes Branches will be excluded from automatic deployment.
* @property previewBranchIncludes Branches will be included for automatic deployment.
* @property previewDeploymentSetting Preview Deployment Setting. Available values: `custom`, `all`, `none`. Defaults to `all`.
* @property productionBranch Project production branch name.
* @property productionDeploymentEnabled Enable production deployments. Defaults to `true`.
* @property repoName Project repository name. **Modifying this attribute will force creation of a new resource.**
*/
public data class PagesProjectSourceConfigArgs(
public val deploymentsEnabled: Output? = null,
public val owner: Output? = null,
public val prCommentsEnabled: Output? = null,
public val previewBranchExcludes: Output>? = null,
public val previewBranchIncludes: Output>? = null,
public val previewDeploymentSetting: Output? = null,
public val productionBranch: Output,
public val productionDeploymentEnabled: Output? = null,
public val repoName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.cloudflare.inputs.PagesProjectSourceConfigArgs =
com.pulumi.cloudflare.inputs.PagesProjectSourceConfigArgs.builder()
.deploymentsEnabled(deploymentsEnabled?.applyValue({ args0 -> args0 }))
.owner(owner?.applyValue({ args0 -> args0 }))
.prCommentsEnabled(prCommentsEnabled?.applyValue({ args0 -> args0 }))
.previewBranchExcludes(previewBranchExcludes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.previewBranchIncludes(previewBranchIncludes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.previewDeploymentSetting(previewDeploymentSetting?.applyValue({ args0 -> args0 }))
.productionBranch(productionBranch.applyValue({ args0 -> args0 }))
.productionDeploymentEnabled(productionDeploymentEnabled?.applyValue({ args0 -> args0 }))
.repoName(repoName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PagesProjectSourceConfigArgs].
*/
@PulumiTagMarker
public class PagesProjectSourceConfigArgsBuilder internal constructor() {
private var deploymentsEnabled: Output? = null
private var owner: Output? = null
private var prCommentsEnabled: Output? = null
private var previewBranchExcludes: Output>? = null
private var previewBranchIncludes: Output>? = null
private var previewDeploymentSetting: Output? = null
private var productionBranch: Output? = null
private var productionDeploymentEnabled: Output? = null
private var repoName: Output? = null
/**
* @param value Toggle deployments on this repo. Defaults to `true`.
*/
@JvmName("tnedxrvtgxlhcmjc")
public suspend fun deploymentsEnabled(`value`: Output) {
this.deploymentsEnabled = value
}
/**
* @param value Project owner username. **Modifying this attribute will force creation of a new resource.**
*/
@JvmName("pwhpiunllakbfwyh")
public suspend fun owner(`value`: Output) {
this.owner = value
}
/**
* @param value Enable Pages to comment on Pull Requests. Defaults to `true`.
*/
@JvmName("votheifptcsgxfue")
public suspend fun prCommentsEnabled(`value`: Output) {
this.prCommentsEnabled = value
}
/**
* @param value Branches will be excluded from automatic deployment.
*/
@JvmName("cuccqkobfkfbndjx")
public suspend fun previewBranchExcludes(`value`: Output>) {
this.previewBranchExcludes = value
}
@JvmName("ysialjcbokvokxwm")
public suspend fun previewBranchExcludes(vararg values: Output) {
this.previewBranchExcludes = Output.all(values.asList())
}
/**
* @param values Branches will be excluded from automatic deployment.
*/
@JvmName("xrkseuwbqytnsjeo")
public suspend fun previewBranchExcludes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy