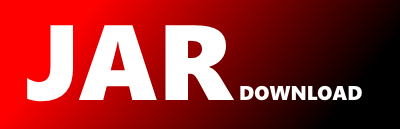
com.pulumi.cloudflare.kotlin.inputs.RulesetRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-cloudflare-kotlin Show documentation
Show all versions of pulumi-cloudflare-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.cloudflare.kotlin.inputs
import com.pulumi.cloudflare.inputs.RulesetRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property action Action to perform in the ruleset rule. Available values: `block`, `challenge`, `compress_response`, `ddos_dynamic`, `ddos_mitigation`, `execute`, `force_connection_close`, `js_challenge`, `log`, `log_custom_field`, `managed_challenge`, `redirect`, `rewrite`, `route`, `score`, `serve_error`, `set_cache_settings`, `set_config`, `skip`.
* @property actionParameters List of parameters that configure the behavior of the ruleset rule action.
* @property description Brief summary of the ruleset rule and its intended use.
* @property enabled Whether the rule is active.
* @property exposedCredentialCheck List of parameters that configure exposed credential checks.
* @property expression Criteria for an HTTP request to trigger the ruleset rule action. Uses the Firewall Rules expression language based on Wireshark display filters. Refer to the [Firewall Rules language](https://developers.cloudflare.com/firewall/cf-firewall-language) documentation for all available fields, operators, and functions.
* @property id Unique rule identifier.
* @property lastUpdated The most recent update to this rule.
* @property logging List parameters to configure how the rule generates logs. Only valid for skip action.
* @property ratelimit List of parameters that configure HTTP rate limiting behaviour.
* @property ref Rule reference.
* @property version Version of the ruleset to deploy.
*/
public data class RulesetRuleArgs(
public val action: Output? = null,
public val actionParameters: Output? = null,
public val description: Output? = null,
public val enabled: Output? = null,
public val exposedCredentialCheck: Output? = null,
public val expression: Output,
public val id: Output? = null,
public val lastUpdated: Output? = null,
public val logging: Output? = null,
public val ratelimit: Output? = null,
public val ref: Output? = null,
public val version: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.cloudflare.inputs.RulesetRuleArgs =
com.pulumi.cloudflare.inputs.RulesetRuleArgs.builder()
.action(action?.applyValue({ args0 -> args0 }))
.actionParameters(actionParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.exposedCredentialCheck(
exposedCredentialCheck?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.expression(expression.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.lastUpdated(lastUpdated?.applyValue({ args0 -> args0 }))
.logging(logging?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ratelimit(ratelimit?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ref(ref?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RulesetRuleArgs].
*/
@PulumiTagMarker
public class RulesetRuleArgsBuilder internal constructor() {
private var action: Output? = null
private var actionParameters: Output? = null
private var description: Output? = null
private var enabled: Output? = null
private var exposedCredentialCheck: Output? = null
private var expression: Output? = null
private var id: Output? = null
private var lastUpdated: Output? = null
private var logging: Output? = null
private var ratelimit: Output? = null
private var ref: Output? = null
private var version: Output? = null
/**
* @param value Action to perform in the ruleset rule. Available values: `block`, `challenge`, `compress_response`, `ddos_dynamic`, `ddos_mitigation`, `execute`, `force_connection_close`, `js_challenge`, `log`, `log_custom_field`, `managed_challenge`, `redirect`, `rewrite`, `route`, `score`, `serve_error`, `set_cache_settings`, `set_config`, `skip`.
*/
@JvmName("jakiblpwuwocdter")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value List of parameters that configure the behavior of the ruleset rule action.
*/
@JvmName("ttmburnksariqbgo")
public suspend fun actionParameters(`value`: Output) {
this.actionParameters = value
}
/**
* @param value Brief summary of the ruleset rule and its intended use.
*/
@JvmName("qsqeiprylbupsghu")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Whether the rule is active.
*/
@JvmName("brurvmxdfvsuffwv")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value List of parameters that configure exposed credential checks.
*/
@JvmName("pvcnhklarbkmjueq")
public suspend fun exposedCredentialCheck(`value`: Output) {
this.exposedCredentialCheck = value
}
/**
* @param value Criteria for an HTTP request to trigger the ruleset rule action. Uses the Firewall Rules expression language based on Wireshark display filters. Refer to the [Firewall Rules language](https://developers.cloudflare.com/firewall/cf-firewall-language) documentation for all available fields, operators, and functions.
*/
@JvmName("bmuvajchdjoekfyi")
public suspend fun expression(`value`: Output) {
this.expression = value
}
/**
* @param value Unique rule identifier.
*/
@JvmName("ealvngtwvtdxmokn")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The most recent update to this rule.
*/
@JvmName("esscxjxjwtiewfjc")
public suspend fun lastUpdated(`value`: Output) {
this.lastUpdated = value
}
/**
* @param value List parameters to configure how the rule generates logs. Only valid for skip action.
*/
@JvmName("byjhgyylqlqfkgis")
public suspend fun logging(`value`: Output) {
this.logging = value
}
/**
* @param value List of parameters that configure HTTP rate limiting behaviour.
*/
@JvmName("rxlwotushsygcgga")
public suspend fun ratelimit(`value`: Output) {
this.ratelimit = value
}
/**
* @param value Rule reference.
*/
@JvmName("verjhhawudciegbr")
public suspend fun ref(`value`: Output) {
this.ref = value
}
/**
* @param value Version of the ruleset to deploy.
*/
@JvmName("fwlkirwvfooqjxxo")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value Action to perform in the ruleset rule. Available values: `block`, `challenge`, `compress_response`, `ddos_dynamic`, `ddos_mitigation`, `execute`, `force_connection_close`, `js_challenge`, `log`, `log_custom_field`, `managed_challenge`, `redirect`, `rewrite`, `route`, `score`, `serve_error`, `set_cache_settings`, `set_config`, `skip`.
*/
@JvmName("vsadmfmrfowpepri")
public suspend fun action(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value List of parameters that configure the behavior of the ruleset rule action.
*/
@JvmName("timcxriwvyjtynqf")
public suspend fun actionParameters(`value`: RulesetRuleActionParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.actionParameters = mapped
}
/**
* @param argument List of parameters that configure the behavior of the ruleset rule action.
*/
@JvmName("ianikvtgfvqauqvm")
public suspend fun actionParameters(argument: suspend RulesetRuleActionParametersArgsBuilder.() -> Unit) {
val toBeMapped = RulesetRuleActionParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.actionParameters = mapped
}
/**
* @param value Brief summary of the ruleset rule and its intended use.
*/
@JvmName("hrdoomrcdphotaqy")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Whether the rule is active.
*/
@JvmName("vbfbyffcigewlrwu")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value List of parameters that configure exposed credential checks.
*/
@JvmName("qldmhnduuecwtmln")
public suspend fun exposedCredentialCheck(`value`: RulesetRuleExposedCredentialCheckArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exposedCredentialCheck = mapped
}
/**
* @param argument List of parameters that configure exposed credential checks.
*/
@JvmName("nnvrytaumbgpeurb")
public suspend fun exposedCredentialCheck(argument: suspend RulesetRuleExposedCredentialCheckArgsBuilder.() -> Unit) {
val toBeMapped = RulesetRuleExposedCredentialCheckArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.exposedCredentialCheck = mapped
}
/**
* @param value Criteria for an HTTP request to trigger the ruleset rule action. Uses the Firewall Rules expression language based on Wireshark display filters. Refer to the [Firewall Rules language](https://developers.cloudflare.com/firewall/cf-firewall-language) documentation for all available fields, operators, and functions.
*/
@JvmName("epxhikyaklptmpiy")
public suspend fun expression(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.expression = mapped
}
/**
* @param value Unique rule identifier.
*/
@JvmName("ywwpxuohqhfrwxjx")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value The most recent update to this rule.
*/
@JvmName("eqtkekseaobwlmyv")
public suspend fun lastUpdated(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastUpdated = mapped
}
/**
* @param value List parameters to configure how the rule generates logs. Only valid for skip action.
*/
@JvmName("ihwrvbpynanpjwky")
public suspend fun logging(`value`: RulesetRuleLoggingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logging = mapped
}
/**
* @param argument List parameters to configure how the rule generates logs. Only valid for skip action.
*/
@JvmName("hfvjxmahfdxdqtdq")
public suspend fun logging(argument: suspend RulesetRuleLoggingArgsBuilder.() -> Unit) {
val toBeMapped = RulesetRuleLoggingArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.logging = mapped
}
/**
* @param value List of parameters that configure HTTP rate limiting behaviour.
*/
@JvmName("oxcclfbgdxesoscb")
public suspend fun ratelimit(`value`: RulesetRuleRatelimitArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ratelimit = mapped
}
/**
* @param argument List of parameters that configure HTTP rate limiting behaviour.
*/
@JvmName("yxyjxqoyarqkqkxv")
public suspend fun ratelimit(argument: suspend RulesetRuleRatelimitArgsBuilder.() -> Unit) {
val toBeMapped = RulesetRuleRatelimitArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ratelimit = mapped
}
/**
* @param value Rule reference.
*/
@JvmName("kqumlovxybdqublf")
public suspend fun ref(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ref = mapped
}
/**
* @param value Version of the ruleset to deploy.
*/
@JvmName("hskewwrbocynuwrt")
public suspend fun version(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): RulesetRuleArgs = RulesetRuleArgs(
action = action,
actionParameters = actionParameters,
description = description,
enabled = enabled,
exposedCredentialCheck = exposedCredentialCheck,
expression = expression ?: throw PulumiNullFieldException("expression"),
id = id,
lastUpdated = lastUpdated,
logging = logging,
ratelimit = ratelimit,
ref = ref,
version = version,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy