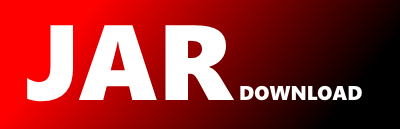
com.pulumi.digitalocean.kotlin.DnsRecord.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-digitalocean-kotlin Show documentation
Show all versions of pulumi-digitalocean-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.digitalocean.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [DnsRecord].
*/
@PulumiTagMarker
public class DnsRecordResourceBuilder internal constructor() {
public var name: String? = null
public var args: DnsRecordArgs = DnsRecordArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DnsRecordArgsBuilder.() -> Unit) {
val builder = DnsRecordArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): DnsRecord {
val builtJavaResource = com.pulumi.digitalocean.DnsRecord(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return DnsRecord(builtJavaResource)
}
}
/**
* Provides a DigitalOcean DNS record resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as digitalocean from "@pulumi/digitalocean";
* const _default = new digitalocean.Domain("default", {name: "example.com"});
* // Add an A record to the domain for www.example.com.
* const www = new digitalocean.DnsRecord("www", {
* domain: _default.id,
* type: digitalocean.RecordType.A,
* name: "www",
* value: "192.168.0.11",
* });
* // Add a MX record for the example.com domain itself.
* const mx = new digitalocean.DnsRecord("mx", {
* domain: _default.id,
* type: digitalocean.RecordType.MX,
* name: "@",
* priority: 10,
* value: "mail.example.com.",
* });
* export const wwwFqdn = www.fqdn;
* export const mxFqdn = mx.fqdn;
* ```
* ```python
* import pulumi
* import pulumi_digitalocean as digitalocean
* default = digitalocean.Domain("default", name="example.com")
* # Add an A record to the domain for www.example.com.
* www = digitalocean.DnsRecord("www",
* domain=default.id,
* type=digitalocean.RecordType.A,
* name="www",
* value="192.168.0.11")
* # Add a MX record for the example.com domain itself.
* mx = digitalocean.DnsRecord("mx",
* domain=default.id,
* type=digitalocean.RecordType.MX,
* name="@",
* priority=10,
* value="mail.example.com.")
* pulumi.export("wwwFqdn", www.fqdn)
* pulumi.export("mxFqdn", mx.fqdn)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using DigitalOcean = Pulumi.DigitalOcean;
* return await Deployment.RunAsync(() =>
* {
* var @default = new DigitalOcean.Domain("default", new()
* {
* Name = "example.com",
* });
* // Add an A record to the domain for www.example.com.
* var www = new DigitalOcean.DnsRecord("www", new()
* {
* Domain = @default.Id,
* Type = DigitalOcean.RecordType.A,
* Name = "www",
* Value = "192.168.0.11",
* });
* // Add a MX record for the example.com domain itself.
* var mx = new DigitalOcean.DnsRecord("mx", new()
* {
* Domain = @default.Id,
* Type = DigitalOcean.RecordType.MX,
* Name = "@",
* Priority = 10,
* Value = "mail.example.com.",
* });
* return new Dictionary
* {
* ["wwwFqdn"] = www.Fqdn,
* ["mxFqdn"] = mx.Fqdn,
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-digitalocean/sdk/v4/go/digitalocean"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := digitalocean.NewDomain(ctx, "default", &digitalocean.DomainArgs{
* Name: pulumi.String("example.com"),
* })
* if err != nil {
* return err
* }
* // Add an A record to the domain for www.example.com.
* www, err := digitalocean.NewDnsRecord(ctx, "www", &digitalocean.DnsRecordArgs{
* Domain: _default.ID(),
* Type: pulumi.String(digitalocean.RecordTypeA),
* Name: pulumi.String("www"),
* Value: pulumi.String("192.168.0.11"),
* })
* if err != nil {
* return err
* }
* // Add a MX record for the example.com domain itself.
* mx, err := digitalocean.NewDnsRecord(ctx, "mx", &digitalocean.DnsRecordArgs{
* Domain: _default.ID(),
* Type: pulumi.String(digitalocean.RecordTypeMX),
* Name: pulumi.String("@"),
* Priority: pulumi.Int(10),
* Value: pulumi.String("mail.example.com."),
* })
* if err != nil {
* return err
* }
* ctx.Export("wwwFqdn", www.Fqdn)
* ctx.Export("mxFqdn", mx.Fqdn)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.digitalocean.Domain;
* import com.pulumi.digitalocean.DomainArgs;
* import com.pulumi.digitalocean.DnsRecord;
* import com.pulumi.digitalocean.DnsRecordArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Domain("default", DomainArgs.builder()
* .name("example.com")
* .build());
* // Add an A record to the domain for www.example.com.
* var www = new DnsRecord("www", DnsRecordArgs.builder()
* .domain(default_.id())
* .type("A")
* .name("www")
* .value("192.168.0.11")
* .build());
* // Add a MX record for the example.com domain itself.
* var mx = new DnsRecord("mx", DnsRecordArgs.builder()
* .domain(default_.id())
* .type("MX")
* .name("@")
* .priority(10)
* .value("mail.example.com.")
* .build());
* ctx.export("wwwFqdn", www.fqdn());
* ctx.export("mxFqdn", mx.fqdn());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: digitalocean:Domain
* properties:
* name: example.com
* # Add an A record to the domain for www.example.com.
* www:
* type: digitalocean:DnsRecord
* properties:
* domain: ${default.id}
* type: A
* name: www
* value: 192.168.0.11
* # Add a MX record for the example.com domain itself.
* mx:
* type: digitalocean:DnsRecord
* properties:
* domain: ${default.id}
* type: MX
* name: '@'
* priority: 10
* value: mail.example.com.
* outputs:
* # Output the FQDN for the www A record.
* wwwFqdn: ${www.fqdn}
* # Output the FQDN for the MX record.
* mxFqdn: ${mx.fqdn}
* ```
*
* ## Import
* Records can be imported using the domain name and record `id` when joined with a comma. See the following example:
* ```sh
* $ pulumi import digitalocean:index/dnsRecord:DnsRecord example_record example.com,12345678
* ```
* ~> You find the `id` of the records [using the DigitalOcean API](https://docs.digitalocean.com/reference/api/api-reference/#operation/domains_list_records) or CLI. Run the follow command to list the IDs for all DNS records on a domain: `doctl compute domain records list `
*/
public class DnsRecord internal constructor(
override val javaResource: com.pulumi.digitalocean.DnsRecord,
) : KotlinCustomResource(javaResource, DnsRecordMapper) {
/**
* The domain to add the record to.
*/
public val domain: Output
get() = javaResource.domain().applyValue({ args0 -> args0 })
/**
* The flags of the record. Only valid when type is `CAA`. Must be between 0 and 255.
*/
public val flags: Output?
get() = javaResource.flags().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The FQDN of the record
*/
public val fqdn: Output
get() = javaResource.fqdn().applyValue({ args0 -> args0 })
/**
* The hostname of the record. Use `@` for records on domain's name itself.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The port of the record. Only valid when type is `SRV`. Must be between 1 and 65535.
*/
public val port: Output?
get() = javaResource.port().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The priority of the record. Only valid when type is `MX` or `SRV`. Must be between 0 and 65535.
*/
public val priority: Output?
get() = javaResource.priority().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The tag of the record. Only valid when type is `CAA`. Must be one of `issue`, `issuewild`, or `iodef`.
*/
public val tag: Output?
get() = javaResource.tag().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The time to live for the record, in seconds. Must be at least 0. Defaults to 1800.
*/
public val ttl: Output
get() = javaResource.ttl().applyValue({ args0 -> args0 })
/**
* The type of record. Must be one of `A`, `AAAA`, `CAA`, `CNAME`, `MX`, `NS`, `TXT`, or `SRV`.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* The value of the record.
*/
public val `value`: Output
get() = javaResource.`value`().applyValue({ args0 -> args0 })
/**
* The weight of the record. Only valid when type is `SRV`. Must be between 0 and 65535.
*/
public val weight: Output?
get() = javaResource.weight().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object DnsRecordMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.digitalocean.DnsRecord::class == javaResource::class
override fun map(javaResource: Resource): DnsRecord = DnsRecord(
javaResource as
com.pulumi.digitalocean.DnsRecord,
)
}
/**
* @see [DnsRecord].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [DnsRecord].
*/
public suspend fun dnsRecord(name: String, block: suspend DnsRecordResourceBuilder.() -> Unit): DnsRecord {
val builder = DnsRecordResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [DnsRecord].
* @param name The _unique_ name of the resulting resource.
*/
public fun dnsRecord(name: String): DnsRecord {
val builder = DnsRecordResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy