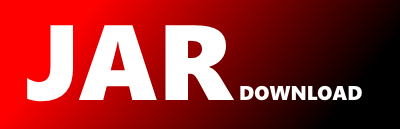
com.pulumi.digitalocean.kotlin.KubernetesClusterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-digitalocean-kotlin Show documentation
Show all versions of pulumi-digitalocean-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.digitalocean.kotlin
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.digitalocean.KubernetesClusterArgs.builder
import com.pulumi.digitalocean.kotlin.enums.Region
import com.pulumi.digitalocean.kotlin.inputs.KubernetesClusterMaintenancePolicyArgs
import com.pulumi.digitalocean.kotlin.inputs.KubernetesClusterMaintenancePolicyArgsBuilder
import com.pulumi.digitalocean.kotlin.inputs.KubernetesClusterNodePoolArgs
import com.pulumi.digitalocean.kotlin.inputs.KubernetesClusterNodePoolArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* ## Import
* Before importing a Kubernetes cluster, the cluster's default node pool must be tagged with
* the `terraform:default-node-pool` tag. The provider will automatically add this tag if
* the cluster only has a single node pool. Clusters with more than one node pool, however, will require
* that you manually add the `terraform:default-node-pool` tag to the node pool that you intend to be
* the default node pool.
* Then the Kubernetes cluster and its default node pool can be imported using the cluster's `id`, e.g.
* ```sh
* $ pulumi import digitalocean:index/kubernetesCluster:KubernetesCluster mycluster 1b8b2100-0e9f-4e8f-ad78-9eb578c2a0af
* ```
* Additional node pools must be imported separately as `digitalocean_kubernetes_cluster`
* resources, e.g.
* ```sh
* $ pulumi import digitalocean:index/kubernetesCluster:KubernetesCluster mynodepool 9d76f410-9284-4436-9633-4066852442c8
* ```
* @property autoUpgrade A boolean value indicating whether the cluster will be automatically upgraded to new patch releases during its maintenance window.
* @property destroyAllAssociatedResources **Use with caution.** When set to true, all associated DigitalOcean resources created via the Kubernetes API (load balancers, volumes, and volume snapshots) will be destroyed along with the cluster when it is destroyed.
* This resource supports customized create timeouts. The default timeout is 30 minutes.
* @property ha Enable/disable the high availability control plane for a cluster. Once enabled for a cluster, high availability cannot be disabled. Default: false
* @property maintenancePolicy A block representing the cluster's maintenance window. Updates will be applied within this window. If not specified, a default maintenance window will be chosen. `auto_upgrade` must be set to `true` for this to have an effect.
* @property name A name for the Kubernetes cluster.
* @property nodePool A block representing the cluster's default node pool. Additional node pools may be added to the cluster using the `digitalocean.KubernetesNodePool` resource. The following arguments may be specified:
* @property region The slug identifier for the region where the Kubernetes cluster will be created.
* @property registryIntegration Enables or disables the DigitalOcean container registry integration for the cluster. This requires that a container registry has first been created for the account. Default: false
* @property surgeUpgrade Enable/disable surge upgrades for a cluster. Default: true
* @property tags A list of tag names to be applied to the Kubernetes cluster.
* @property version The slug identifier for the version of Kubernetes used for the cluster. Use [doctl](https://github.com/digitalocean/doctl) to find the available versions `doctl kubernetes options versions`. (**Note:** A cluster may only be upgraded to newer versions in-place. If the version is decreased, a new resource will be created.)
* @property vpcUuid The ID of the VPC where the Kubernetes cluster will be located.
*/
public data class KubernetesClusterArgs(
public val autoUpgrade: Output? = null,
public val destroyAllAssociatedResources: Output? = null,
public val ha: Output? = null,
public val maintenancePolicy: Output? = null,
public val name: Output? = null,
public val nodePool: Output? = null,
public val region: Output>? = null,
public val registryIntegration: Output? = null,
public val surgeUpgrade: Output? = null,
public val tags: Output>? = null,
public val version: Output? = null,
public val vpcUuid: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.digitalocean.KubernetesClusterArgs =
com.pulumi.digitalocean.KubernetesClusterArgs.builder()
.autoUpgrade(autoUpgrade?.applyValue({ args0 -> args0 }))
.destroyAllAssociatedResources(destroyAllAssociatedResources?.applyValue({ args0 -> args0 }))
.ha(ha?.applyValue({ args0 -> args0 }))
.maintenancePolicy(maintenancePolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 }))
.nodePool(nodePool?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.region(
region?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.registryIntegration(registryIntegration?.applyValue({ args0 -> args0 }))
.surgeUpgrade(surgeUpgrade?.applyValue({ args0 -> args0 }))
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.version(version?.applyValue({ args0 -> args0 }))
.vpcUuid(vpcUuid?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [KubernetesClusterArgs].
*/
@PulumiTagMarker
public class KubernetesClusterArgsBuilder internal constructor() {
private var autoUpgrade: Output? = null
private var destroyAllAssociatedResources: Output? = null
private var ha: Output? = null
private var maintenancePolicy: Output? = null
private var name: Output? = null
private var nodePool: Output? = null
private var region: Output>? = null
private var registryIntegration: Output? = null
private var surgeUpgrade: Output? = null
private var tags: Output>? = null
private var version: Output? = null
private var vpcUuid: Output? = null
/**
* @param value A boolean value indicating whether the cluster will be automatically upgraded to new patch releases during its maintenance window.
*/
@JvmName("sdupqspqmhdjdfal")
public suspend fun autoUpgrade(`value`: Output) {
this.autoUpgrade = value
}
/**
* @param value **Use with caution.** When set to true, all associated DigitalOcean resources created via the Kubernetes API (load balancers, volumes, and volume snapshots) will be destroyed along with the cluster when it is destroyed.
* This resource supports customized create timeouts. The default timeout is 30 minutes.
*/
@JvmName("exkqwiptorasikei")
public suspend fun destroyAllAssociatedResources(`value`: Output) {
this.destroyAllAssociatedResources = value
}
/**
* @param value Enable/disable the high availability control plane for a cluster. Once enabled for a cluster, high availability cannot be disabled. Default: false
*/
@JvmName("hjibgimpcuqduuhw")
public suspend fun ha(`value`: Output) {
this.ha = value
}
/**
* @param value A block representing the cluster's maintenance window. Updates will be applied within this window. If not specified, a default maintenance window will be chosen. `auto_upgrade` must be set to `true` for this to have an effect.
*/
@JvmName("limlomixadpsejxp")
public suspend fun maintenancePolicy(`value`: Output) {
this.maintenancePolicy = value
}
/**
* @param value A name for the Kubernetes cluster.
*/
@JvmName("wnmlddusvopbglps")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A block representing the cluster's default node pool. Additional node pools may be added to the cluster using the `digitalocean.KubernetesNodePool` resource. The following arguments may be specified:
*/
@JvmName("astfrvpjouvgapwa")
public suspend fun nodePool(`value`: Output) {
this.nodePool = value
}
/**
* @param value The slug identifier for the region where the Kubernetes cluster will be created.
*/
@JvmName("ffvjbvacgpvsjxyh")
public suspend fun region(`value`: Output>) {
this.region = value
}
/**
* @param value Enables or disables the DigitalOcean container registry integration for the cluster. This requires that a container registry has first been created for the account. Default: false
*/
@JvmName("fheafbquspxrvlbn")
public suspend fun registryIntegration(`value`: Output) {
this.registryIntegration = value
}
/**
* @param value Enable/disable surge upgrades for a cluster. Default: true
*/
@JvmName("pxcyhrbveaiqiieh")
public suspend fun surgeUpgrade(`value`: Output) {
this.surgeUpgrade = value
}
/**
* @param value A list of tag names to be applied to the Kubernetes cluster.
*/
@JvmName("xrrcaomyyxlifopj")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("djgmdijewqhayucp")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values A list of tag names to be applied to the Kubernetes cluster.
*/
@JvmName("gxkkwrlkyvfhlheh")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy