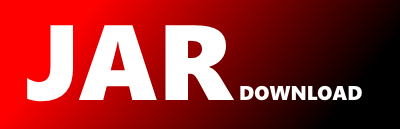
com.pulumi.digitalocean.kotlin.Vpc.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-digitalocean-kotlin Show documentation
Show all versions of pulumi-digitalocean-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.digitalocean.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Vpc].
*/
@PulumiTagMarker
public class VpcResourceBuilder internal constructor() {
public var name: String? = null
public var args: VpcArgs = VpcArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend VpcArgsBuilder.() -> Unit) {
val builder = VpcArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Vpc {
val builtJavaResource = com.pulumi.digitalocean.Vpc(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Vpc(builtJavaResource)
}
}
/**
* Provides a [DigitalOcean VPC](https://docs.digitalocean.com/reference/api/api-reference/#tag/VPCs) resource.
* VPCs are virtual networks containing resources that can communicate with each
* other in full isolation, using private IP addresses.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as digitalocean from "@pulumi/digitalocean";
* const example = new digitalocean.Vpc("example", {
* name: "example-project-network",
* region: "nyc3",
* ipRange: "10.10.10.0/24",
* });
* ```
* ```python
* import pulumi
* import pulumi_digitalocean as digitalocean
* example = digitalocean.Vpc("example",
* name="example-project-network",
* region="nyc3",
* ip_range="10.10.10.0/24")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using DigitalOcean = Pulumi.DigitalOcean;
* return await Deployment.RunAsync(() =>
* {
* var example = new DigitalOcean.Vpc("example", new()
* {
* Name = "example-project-network",
* Region = "nyc3",
* IpRange = "10.10.10.0/24",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-digitalocean/sdk/v4/go/digitalocean"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := digitalocean.NewVpc(ctx, "example", &digitalocean.VpcArgs{
* Name: pulumi.String("example-project-network"),
* Region: pulumi.String("nyc3"),
* IpRange: pulumi.String("10.10.10.0/24"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.digitalocean.Vpc;
* import com.pulumi.digitalocean.VpcArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Vpc("example", VpcArgs.builder()
* .name("example-project-network")
* .region("nyc3")
* .ipRange("10.10.10.0/24")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: digitalocean:Vpc
* properties:
* name: example-project-network
* region: nyc3
* ipRange: 10.10.10.0/24
* ```
*
* ### Resource Assignment
* `digitalocean.Droplet`, `digitalocean.KubernetesCluster`,
* `digitalocean_load_balancer`, and `digitalocean.DatabaseCluster` resources
* may be assigned to a VPC by referencing its `id`. For example:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as digitalocean from "@pulumi/digitalocean";
* const example = new digitalocean.Vpc("example", {
* name: "example-project-network",
* region: "nyc3",
* });
* const exampleDroplet = new digitalocean.Droplet("example", {
* name: "example-01",
* size: digitalocean.DropletSlug.DropletS1VCPU1GB,
* image: "ubuntu-18-04-x64",
* region: digitalocean.Region.NYC3,
* vpcUuid: example.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_digitalocean as digitalocean
* example = digitalocean.Vpc("example",
* name="example-project-network",
* region="nyc3")
* example_droplet = digitalocean.Droplet("example",
* name="example-01",
* size=digitalocean.DropletSlug.DROPLET_S1_VCPU1_GB,
* image="ubuntu-18-04-x64",
* region=digitalocean.Region.NYC3,
* vpc_uuid=example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using DigitalOcean = Pulumi.DigitalOcean;
* return await Deployment.RunAsync(() =>
* {
* var example = new DigitalOcean.Vpc("example", new()
* {
* Name = "example-project-network",
* Region = "nyc3",
* });
* var exampleDroplet = new DigitalOcean.Droplet("example", new()
* {
* Name = "example-01",
* Size = DigitalOcean.DropletSlug.DropletS1VCPU1GB,
* Image = "ubuntu-18-04-x64",
* Region = DigitalOcean.Region.NYC3,
* VpcUuid = example.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-digitalocean/sdk/v4/go/digitalocean"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := digitalocean.NewVpc(ctx, "example", &digitalocean.VpcArgs{
* Name: pulumi.String("example-project-network"),
* Region: pulumi.String("nyc3"),
* })
* if err != nil {
* return err
* }
* _, err = digitalocean.NewDroplet(ctx, "example", &digitalocean.DropletArgs{
* Name: pulumi.String("example-01"),
* Size: pulumi.String(digitalocean.DropletSlugDropletS1VCPU1GB),
* Image: pulumi.String("ubuntu-18-04-x64"),
* Region: pulumi.String(digitalocean.RegionNYC3),
* VpcUuid: example.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.digitalocean.Vpc;
* import com.pulumi.digitalocean.VpcArgs;
* import com.pulumi.digitalocean.Droplet;
* import com.pulumi.digitalocean.DropletArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Vpc("example", VpcArgs.builder()
* .name("example-project-network")
* .region("nyc3")
* .build());
* var exampleDroplet = new Droplet("exampleDroplet", DropletArgs.builder()
* .name("example-01")
* .size("s-1vcpu-1gb")
* .image("ubuntu-18-04-x64")
* .region("nyc3")
* .vpcUuid(example.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: digitalocean:Vpc
* properties:
* name: example-project-network
* region: nyc3
* exampleDroplet:
* type: digitalocean:Droplet
* name: example
* properties:
* name: example-01
* size: s-1vcpu-1gb
* image: ubuntu-18-04-x64
* region: nyc3
* vpcUuid: ${example.id}
* ```
*
* ## Import
* A VPC can be imported using its `id`, e.g.
* ```sh
* $ pulumi import digitalocean:index/vpc:Vpc example 506f78a4-e098-11e5-ad9f-000f53306ae1
* ```
*/
public class Vpc internal constructor(
override val javaResource: com.pulumi.digitalocean.Vpc,
) : KotlinCustomResource(javaResource, VpcMapper) {
/**
* The date and time of when the VPC was created.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* A boolean indicating whether or not the VPC is the default one for the region.
*/
public val default: Output
get() = javaResource.default_().applyValue({ args0 -> args0 })
/**
* A free-form text field up to a limit of 255 characters to describe the VPC.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The range of IP addresses for the VPC in CIDR notation. Network ranges cannot overlap with other networks in the same account and must be in range of private addresses as defined in RFC1918. It may not be larger than `/16` or smaller than `/24`.
*/
public val ipRange: Output
get() = javaResource.ipRange().applyValue({ args0 -> args0 })
/**
* A name for the VPC. Must be unique and contain alphanumeric characters, dashes, and periods only.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The DigitalOcean region slug for the VPC's location.
*/
public val region: Output
get() = javaResource.region().applyValue({ args0 -> args0 })
/**
* The uniform resource name (URN) for the VPC.
*/
public val vpcUrn: Output
get() = javaResource.vpcUrn().applyValue({ args0 -> args0 })
}
public object VpcMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.digitalocean.Vpc::class == javaResource::class
override fun map(javaResource: Resource): Vpc = Vpc(javaResource as com.pulumi.digitalocean.Vpc)
}
/**
* @see [Vpc].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Vpc].
*/
public suspend fun vpc(name: String, block: suspend VpcResourceBuilder.() -> Unit): Vpc {
val builder = VpcResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Vpc].
* @param name The _unique_ name of the resulting resource.
*/
public fun vpc(name: String): Vpc {
val builder = VpcResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy