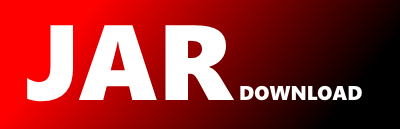
com.pulumi.digitalocean.kotlin.inputs.AppSpecDomainNameArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-digitalocean-kotlin Show documentation
Show all versions of pulumi-digitalocean-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.digitalocean.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.digitalocean.inputs.AppSpecDomainNameArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property name The hostname for the domain.
* @property type The domain type, which can be one of the following:
* - `DEFAULT`: The default .ondigitalocean.app domain assigned to this app.
* - `PRIMARY`: The primary domain for this app that is displayed as the default in the control panel, used in bindable environment variables, and any other places that reference an app's live URL. Only one domain may be set as primary.
* - `ALIAS`: A non-primary domain.
* @property wildcard A boolean indicating whether the domain includes all sub-domains, in addition to the given domain.
* @property zone If the domain uses DigitalOcean DNS and you would like App Platform to automatically manage it for you, set this to the name of the domain on your account.
*/
public data class AppSpecDomainNameArgs(
public val name: Output,
public val type: Output? = null,
public val wildcard: Output? = null,
public val zone: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.digitalocean.inputs.AppSpecDomainNameArgs =
com.pulumi.digitalocean.inputs.AppSpecDomainNameArgs.builder()
.name(name.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 }))
.wildcard(wildcard?.applyValue({ args0 -> args0 }))
.zone(zone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AppSpecDomainNameArgs].
*/
@PulumiTagMarker
public class AppSpecDomainNameArgsBuilder internal constructor() {
private var name: Output? = null
private var type: Output? = null
private var wildcard: Output? = null
private var zone: Output? = null
/**
* @param value The hostname for the domain.
*/
@JvmName("feccxyplxgwaranj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The domain type, which can be one of the following:
* - `DEFAULT`: The default .ondigitalocean.app domain assigned to this app.
* - `PRIMARY`: The primary domain for this app that is displayed as the default in the control panel, used in bindable environment variables, and any other places that reference an app's live URL. Only one domain may be set as primary.
* - `ALIAS`: A non-primary domain.
*/
@JvmName("qcdpbawfrjiaxxux")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value A boolean indicating whether the domain includes all sub-domains, in addition to the given domain.
*/
@JvmName("emtuldqycthjakmn")
public suspend fun wildcard(`value`: Output) {
this.wildcard = value
}
/**
* @param value If the domain uses DigitalOcean DNS and you would like App Platform to automatically manage it for you, set this to the name of the domain on your account.
*/
@JvmName("wvcfonsrdtmritiv")
public suspend fun zone(`value`: Output) {
this.zone = value
}
/**
* @param value The hostname for the domain.
*/
@JvmName("sdgtadsbcwgnwxvg")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The domain type, which can be one of the following:
* - `DEFAULT`: The default .ondigitalocean.app domain assigned to this app.
* - `PRIMARY`: The primary domain for this app that is displayed as the default in the control panel, used in bindable environment variables, and any other places that reference an app's live URL. Only one domain may be set as primary.
* - `ALIAS`: A non-primary domain.
*/
@JvmName("chrdkwelasjmxgad")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value A boolean indicating whether the domain includes all sub-domains, in addition to the given domain.
*/
@JvmName("jksheoiljoiyjotq")
public suspend fun wildcard(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wildcard = mapped
}
/**
* @param value If the domain uses DigitalOcean DNS and you would like App Platform to automatically manage it for you, set this to the name of the domain on your account.
*/
@JvmName("kcywbhrcxacgilot")
public suspend fun zone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zone = mapped
}
internal fun build(): AppSpecDomainNameArgs = AppSpecDomainNameArgs(
name = name ?: throw PulumiNullFieldException("name"),
type = type,
wildcard = wildcard,
zone = zone,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy