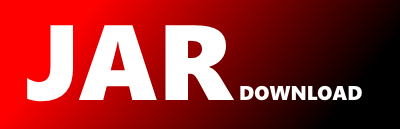
com.pulumi.digitalocean.kotlin.outputs.AppSpec.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-digitalocean-kotlin Show documentation
Show all versions of pulumi-digitalocean-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.digitalocean.kotlin.outputs
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property alerts Describes an alert policy for the app.
* @property databases
* @property domainNames Describes a domain where the application will be made available.
* @property domains
* @property egresses Specification for app egress configurations.
* @property envs Describes an app-wide environment variable made available to all components.
* @property features A list of the features applied to the app. The default buildpack can be overridden here. List of available buildpacks can be found using the [doctl CLI](https://docs.digitalocean.com/reference/doctl/reference/apps/list-buildpacks/)
* @property functions
* @property ingress Specification for component routing, rewrites, and redirects.
* @property jobs
* @property name The name of the app. Must be unique across all apps in the same account.
* @property region The slug for the DigitalOcean data center region hosting the app.
* @property services
* @property staticSites
* @property workers
*/
public data class AppSpec(
public val alerts: List? = null,
public val databases: List? = null,
public val domainNames: List? = null,
@Deprecated(
message = """
This attribute has been replaced by `domain` which supports additional functionality.
""",
)
public val domains: List? = null,
public val egresses: List? = null,
public val envs: List? = null,
public val features: List? = null,
public val functions: List? = null,
public val ingress: AppSpecIngress? = null,
public val jobs: List? = null,
public val name: String,
public val region: String? = null,
public val services: List? = null,
public val staticSites: List? = null,
public val workers: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.digitalocean.outputs.AppSpec): AppSpec = AppSpec(
alerts = javaType.alerts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecAlert.Companion.toKotlin(args0)
})
}),
databases = javaType.databases().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecDatabase.Companion.toKotlin(args0)
})
}),
domainNames = javaType.domainNames().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecDomainName.Companion.toKotlin(args0)
})
}),
domains = javaType.domains().map({ args0 -> args0 }),
egresses = javaType.egresses().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecEgress.Companion.toKotlin(args0)
})
}),
envs = javaType.envs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecEnv.Companion.toKotlin(args0)
})
}),
features = javaType.features().map({ args0 -> args0 }),
functions = javaType.functions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecFunction.Companion.toKotlin(args0)
})
}),
ingress = javaType.ingress().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecIngress.Companion.toKotlin(args0)
})
}).orElse(null),
jobs = javaType.jobs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecJob.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
region = javaType.region().map({ args0 -> args0 }).orElse(null),
services = javaType.services().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecService.Companion.toKotlin(args0)
})
}),
staticSites = javaType.staticSites().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecStaticSite.Companion.toKotlin(args0)
})
}),
workers = javaType.workers().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.AppSpecWorker.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy