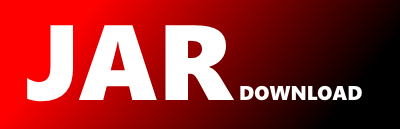
com.pulumi.digitalocean.kotlin.outputs.GetDropletsDroplet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-digitalocean-kotlin Show documentation
Show all versions of pulumi-digitalocean-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.digitalocean.kotlin.outputs
import kotlin.Boolean
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property backups Whether backups are enabled.
* @property createdAt the creation date for the Droplet
* @property disk The size of the Droplet's disk in GB.
* @property id The ID of the Droplet.
* @property image The Droplet image ID or slug.
* @property ipv4Address The Droplet's public IPv4 address
* @property ipv4AddressPrivate The Droplet's private IPv4 address
* @property ipv6 Whether IPv6 is enabled.
* @property ipv6Address The Droplet's public IPv6 address
* @property ipv6AddressPrivate The Droplet's private IPv6 address
* @property locked Whether the Droplet is locked.
* @property memory The amount of the Droplet's memory in MB.
* @property monitoring Whether monitoring agent is installed.
* @property name name of the Droplet
* @property priceHourly Droplet hourly price.
* @property priceMonthly Droplet monthly price.
* @property privateNetworking Whether private networks are enabled.
* @property region The region the Droplet is running in.
* @property size The unique slug that identifies the type of Droplet.
* @property status The status of the Droplet.
* @property tags A list of the tags associated to the Droplet.
* @property urn The uniform resource name of the Droplet
* @property vcpus The number of the Droplet's virtual CPUs.
* @property volumeIds List of the IDs of each volumes attached to the Droplet.
* @property vpcUuid The ID of the VPC where the Droplet is located.
*/
public data class GetDropletsDroplet(
public val backups: Boolean,
public val createdAt: String,
public val disk: Int,
public val id: Int,
public val image: String,
public val ipv4Address: String,
public val ipv4AddressPrivate: String,
public val ipv6: Boolean,
public val ipv6Address: String,
public val ipv6AddressPrivate: String,
public val locked: Boolean,
public val memory: Int,
public val monitoring: Boolean,
public val name: String,
public val priceHourly: Double,
public val priceMonthly: Double,
public val privateNetworking: Boolean,
public val region: String,
public val size: String,
public val status: String,
public val tags: List,
public val urn: String,
public val vcpus: Int,
public val volumeIds: List,
public val vpcUuid: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.digitalocean.outputs.GetDropletsDroplet): GetDropletsDroplet = GetDropletsDroplet(
backups = javaType.backups(),
createdAt = javaType.createdAt(),
disk = javaType.disk(),
id = javaType.id(),
image = javaType.image(),
ipv4Address = javaType.ipv4Address(),
ipv4AddressPrivate = javaType.ipv4AddressPrivate(),
ipv6 = javaType.ipv6(),
ipv6Address = javaType.ipv6Address(),
ipv6AddressPrivate = javaType.ipv6AddressPrivate(),
locked = javaType.locked(),
memory = javaType.memory(),
monitoring = javaType.monitoring(),
name = javaType.name(),
priceHourly = javaType.priceHourly(),
priceMonthly = javaType.priceMonthly(),
privateNetworking = javaType.privateNetworking(),
region = javaType.region(),
size = javaType.size(),
status = javaType.status(),
tags = javaType.tags().map({ args0 -> args0 }),
urn = javaType.urn(),
vcpus = javaType.vcpus(),
volumeIds = javaType.volumeIds().map({ args0 -> args0 }),
vpcUuid = javaType.vpcUuid(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy