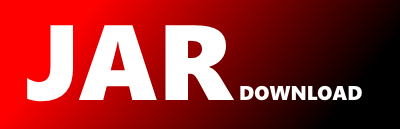
com.pulumi.digitalocean.kotlin.outputs.GetLoadBalancerResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-digitalocean-kotlin Show documentation
Show all versions of pulumi-digitalocean-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.digitalocean.kotlin.outputs
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getLoadBalancer.
* @property algorithm
* @property disableLetsEncryptDnsRecords
* @property domains
* @property dropletIds
* @property dropletTag
* @property enableBackendKeepalive
* @property enableProxyProtocol
* @property firewalls
* @property forwardingRules
* @property glbSettings
* @property healthchecks
* @property httpIdleTimeoutSeconds
* @property id
* @property ip
* @property loadBalancerUrn
* @property name
* @property network
* @property projectId
* @property redirectHttpToHttps
* @property region
* @property size
* @property sizeUnit
* @property status
* @property stickySessions
* @property targetLoadBalancerIds
* @property type
* @property vpcUuid
*/
public data class GetLoadBalancerResult(
@Deprecated(
message = """
This field has been deprecated. You can no longer specify an algorithm for load balancers.
""",
)
public val algorithm: String,
public val disableLetsEncryptDnsRecords: Boolean,
public val domains: List,
public val dropletIds: List,
public val dropletTag: String,
public val enableBackendKeepalive: Boolean,
public val enableProxyProtocol: Boolean,
public val firewalls: List,
public val forwardingRules: List,
public val glbSettings: List,
public val healthchecks: List,
public val httpIdleTimeoutSeconds: Int,
public val id: String? = null,
public val ip: String,
public val loadBalancerUrn: String,
public val name: String? = null,
public val network: String,
public val projectId: String,
public val redirectHttpToHttps: Boolean,
public val region: String,
public val size: String,
public val sizeUnit: Int,
public val status: String,
public val stickySessions: List,
public val targetLoadBalancerIds: List,
public val type: String,
public val vpcUuid: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.digitalocean.outputs.GetLoadBalancerResult): GetLoadBalancerResult = GetLoadBalancerResult(
algorithm = javaType.algorithm(),
disableLetsEncryptDnsRecords = javaType.disableLetsEncryptDnsRecords(),
domains = javaType.domains().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.GetLoadBalancerDomain.Companion.toKotlin(args0)
})
}),
dropletIds = javaType.dropletIds().map({ args0 -> args0 }),
dropletTag = javaType.dropletTag(),
enableBackendKeepalive = javaType.enableBackendKeepalive(),
enableProxyProtocol = javaType.enableProxyProtocol(),
firewalls = javaType.firewalls().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.GetLoadBalancerFirewall.Companion.toKotlin(args0)
})
}),
forwardingRules = javaType.forwardingRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.GetLoadBalancerForwardingRule.Companion.toKotlin(args0)
})
}),
glbSettings = javaType.glbSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.GetLoadBalancerGlbSetting.Companion.toKotlin(args0)
})
}),
healthchecks = javaType.healthchecks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.GetLoadBalancerHealthcheck.Companion.toKotlin(args0)
})
}),
httpIdleTimeoutSeconds = javaType.httpIdleTimeoutSeconds(),
id = javaType.id().map({ args0 -> args0 }).orElse(null),
ip = javaType.ip(),
loadBalancerUrn = javaType.loadBalancerUrn(),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
network = javaType.network(),
projectId = javaType.projectId(),
redirectHttpToHttps = javaType.redirectHttpToHttps(),
region = javaType.region(),
size = javaType.size(),
sizeUnit = javaType.sizeUnit(),
status = javaType.status(),
stickySessions = javaType.stickySessions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.digitalocean.kotlin.outputs.GetLoadBalancerStickySession.Companion.toKotlin(args0)
})
}),
targetLoadBalancerIds = javaType.targetLoadBalancerIds().map({ args0 -> args0 }),
type = javaType.type(),
vpcUuid = javaType.vpcUuid(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy