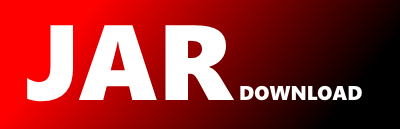
com.pulumi.gcp.accesscontextmanager.kotlin.inputs.AccessLevelsAccessLevelArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.accesscontextmanager.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.accesscontextmanager.inputs.AccessLevelsAccessLevelArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property basic A set of predefined conditions for the access level and a combining function.
* Structure is documented below.
* @property custom Custom access level conditions are set using the Cloud Common Expression Language to represent the necessary conditions for the level to apply to a request.
* See CEL spec at: https://github.com/google/cel-spec.
* Structure is documented below.
* @property description Description of the AccessLevel and its use. Does not affect behavior.
* @property name Resource name for the Access Level. The short_name component must begin
* with a letter and only include alphanumeric and '_'.
* Format: accessPolicies/{policy_id}/accessLevels/{short_name}
* @property title Human readable title. Must be unique within the Policy.
*/
public data class AccessLevelsAccessLevelArgs(
public val basic: Output? = null,
public val custom: Output? = null,
public val description: Output? = null,
public val name: Output,
public val title: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.accesscontextmanager.inputs.AccessLevelsAccessLevelArgs =
com.pulumi.gcp.accesscontextmanager.inputs.AccessLevelsAccessLevelArgs.builder()
.basic(basic?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.custom(custom?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.title(title.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AccessLevelsAccessLevelArgs].
*/
@PulumiTagMarker
public class AccessLevelsAccessLevelArgsBuilder internal constructor() {
private var basic: Output? = null
private var custom: Output? = null
private var description: Output? = null
private var name: Output? = null
private var title: Output? = null
/**
* @param value A set of predefined conditions for the access level and a combining function.
* Structure is documented below.
*/
@JvmName("glqdgbxabuwdrcqs")
public suspend fun basic(`value`: Output) {
this.basic = value
}
/**
* @param value Custom access level conditions are set using the Cloud Common Expression Language to represent the necessary conditions for the level to apply to a request.
* See CEL spec at: https://github.com/google/cel-spec.
* Structure is documented below.
*/
@JvmName("qgrqqytqgfnlkbjr")
public suspend fun custom(`value`: Output) {
this.custom = value
}
/**
* @param value Description of the AccessLevel and its use. Does not affect behavior.
*/
@JvmName("lwnrryilnowpkmvh")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Resource name for the Access Level. The short_name component must begin
* with a letter and only include alphanumeric and '_'.
* Format: accessPolicies/{policy_id}/accessLevels/{short_name}
*/
@JvmName("sfyjpyrkiufucmfx")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Human readable title. Must be unique within the Policy.
*/
@JvmName("ddrmpfjsdsiawttt")
public suspend fun title(`value`: Output) {
this.title = value
}
/**
* @param value A set of predefined conditions for the access level and a combining function.
* Structure is documented below.
*/
@JvmName("rfpdvpkjwcwkejuv")
public suspend fun basic(`value`: AccessLevelsAccessLevelBasicArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.basic = mapped
}
/**
* @param argument A set of predefined conditions for the access level and a combining function.
* Structure is documented below.
*/
@JvmName("fwusjhtbxamxgetf")
public suspend fun basic(argument: suspend AccessLevelsAccessLevelBasicArgsBuilder.() -> Unit) {
val toBeMapped = AccessLevelsAccessLevelBasicArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.basic = mapped
}
/**
* @param value Custom access level conditions are set using the Cloud Common Expression Language to represent the necessary conditions for the level to apply to a request.
* See CEL spec at: https://github.com/google/cel-spec.
* Structure is documented below.
*/
@JvmName("hvctgyjwgwtlirde")
public suspend fun custom(`value`: AccessLevelsAccessLevelCustomArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.custom = mapped
}
/**
* @param argument Custom access level conditions are set using the Cloud Common Expression Language to represent the necessary conditions for the level to apply to a request.
* See CEL spec at: https://github.com/google/cel-spec.
* Structure is documented below.
*/
@JvmName("ajcnbiovoendutpj")
public suspend fun custom(argument: suspend AccessLevelsAccessLevelCustomArgsBuilder.() -> Unit) {
val toBeMapped = AccessLevelsAccessLevelCustomArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.custom = mapped
}
/**
* @param value Description of the AccessLevel and its use. Does not affect behavior.
*/
@JvmName("hgjlrxueahkfmuwt")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Resource name for the Access Level. The short_name component must begin
* with a letter and only include alphanumeric and '_'.
* Format: accessPolicies/{policy_id}/accessLevels/{short_name}
*/
@JvmName("tpplwjaxddmhjobl")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Human readable title. Must be unique within the Policy.
*/
@JvmName("dahsavkrotxhtclt")
public suspend fun title(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.title = mapped
}
internal fun build(): AccessLevelsAccessLevelArgs = AccessLevelsAccessLevelArgs(
basic = basic,
custom = custom,
description = description,
name = name ?: throw PulumiNullFieldException("name"),
title = title ?: throw PulumiNullFieldException("title"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy