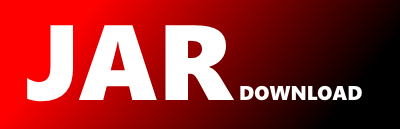
com.pulumi.gcp.accesscontextmanager.kotlin.inputs.ServicePerimetersServicePerimeterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.accesscontextmanager.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.accesscontextmanager.inputs.ServicePerimetersServicePerimeterArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property createTime (Output)
* Time the AccessPolicy was created in UTC.
* @property description Description of the ServicePerimeter and its use. Does not affect
* behavior.
* @property name Resource name for the ServicePerimeter. The short_name component must
* begin with a letter and only include alphanumeric and '_'.
* Format: accessPolicies/{policy_id}/servicePerimeters/{short_name}
* @property perimeterType Specifies the type of the Perimeter. There are two types: regular and
* bridge. Regular Service Perimeter contains resources, access levels,
* and restricted services. Every resource can be in at most
* ONE regular Service Perimeter.
* In addition to being in a regular service perimeter, a resource can also
* be in zero or more perimeter bridges. A perimeter bridge only contains
* resources. Cross project operations are permitted if all effected
* resources share some perimeter (whether bridge or regular). Perimeter
* Bridge does not contain access levels or services: those are governed
* entirely by the regular perimeter that resource is in.
* Perimeter Bridges are typically useful when building more complex
* topologies with many independent perimeters that need to share some data
* with a common perimeter, but should not be able to share data among
* themselves.
* Default value is `PERIMETER_TYPE_REGULAR`.
* Possible values are: `PERIMETER_TYPE_REGULAR`, `PERIMETER_TYPE_BRIDGE`.
* @property spec Proposed (or dry run) ServicePerimeter configuration.
* This configuration allows to specify and test ServicePerimeter configuration
* without enforcing actual access restrictions. Only allowed to be set when
* the `useExplicitDryRunSpec` flag is set.
* Structure is documented below.
* @property status ServicePerimeter configuration. Specifies sets of resources,
* restricted services and access levels that determine
* perimeter content and boundaries.
* Structure is documented below.
* @property title Human readable title. Must be unique within the Policy.
* @property updateTime (Output)
* Time the AccessPolicy was updated in UTC.
* @property useExplicitDryRunSpec Use explicit dry run spec flag. Ordinarily, a dry-run spec implicitly exists
* for all Service Perimeters, and that spec is identical to the status for those
* Service Perimeters. When this flag is set, it inhibits the generation of the
* implicit spec, thereby allowing the user to explicitly provide a
* configuration ("spec") to use in a dry-run version of the Service Perimeter.
* This allows the user to test changes to the enforced config ("status") without
* actually enforcing them. This testing is done through analyzing the differences
* between currently enforced and suggested restrictions. useExplicitDryRunSpec must
* bet set to True if any of the fields in the spec are set to non-default values.
*/
public data class ServicePerimetersServicePerimeterArgs(
public val createTime: Output? = null,
public val description: Output? = null,
public val name: Output,
public val perimeterType: Output? = null,
public val spec: Output? = null,
public val status: Output? = null,
public val title: Output,
public val updateTime: Output? = null,
public val useExplicitDryRunSpec: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.accesscontextmanager.inputs.ServicePerimetersServicePerimeterArgs =
com.pulumi.gcp.accesscontextmanager.inputs.ServicePerimetersServicePerimeterArgs.builder()
.createTime(createTime?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.perimeterType(perimeterType?.applyValue({ args0 -> args0 }))
.spec(spec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.status(status?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.title(title.applyValue({ args0 -> args0 }))
.updateTime(updateTime?.applyValue({ args0 -> args0 }))
.useExplicitDryRunSpec(useExplicitDryRunSpec?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServicePerimetersServicePerimeterArgs].
*/
@PulumiTagMarker
public class ServicePerimetersServicePerimeterArgsBuilder internal constructor() {
private var createTime: Output? = null
private var description: Output? = null
private var name: Output? = null
private var perimeterType: Output? = null
private var spec: Output? = null
private var status: Output? = null
private var title: Output? = null
private var updateTime: Output? = null
private var useExplicitDryRunSpec: Output? = null
/**
* @param value (Output)
* Time the AccessPolicy was created in UTC.
*/
@JvmName("amwukshofmylpktl")
public suspend fun createTime(`value`: Output) {
this.createTime = value
}
/**
* @param value Description of the ServicePerimeter and its use. Does not affect
* behavior.
*/
@JvmName("voeixpwvxcxogpil")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Resource name for the ServicePerimeter. The short_name component must
* begin with a letter and only include alphanumeric and '_'.
* Format: accessPolicies/{policy_id}/servicePerimeters/{short_name}
*/
@JvmName("smgorpjrhdgbsxsd")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Specifies the type of the Perimeter. There are two types: regular and
* bridge. Regular Service Perimeter contains resources, access levels,
* and restricted services. Every resource can be in at most
* ONE regular Service Perimeter.
* In addition to being in a regular service perimeter, a resource can also
* be in zero or more perimeter bridges. A perimeter bridge only contains
* resources. Cross project operations are permitted if all effected
* resources share some perimeter (whether bridge or regular). Perimeter
* Bridge does not contain access levels or services: those are governed
* entirely by the regular perimeter that resource is in.
* Perimeter Bridges are typically useful when building more complex
* topologies with many independent perimeters that need to share some data
* with a common perimeter, but should not be able to share data among
* themselves.
* Default value is `PERIMETER_TYPE_REGULAR`.
* Possible values are: `PERIMETER_TYPE_REGULAR`, `PERIMETER_TYPE_BRIDGE`.
*/
@JvmName("ajswnegelbawsjty")
public suspend fun perimeterType(`value`: Output) {
this.perimeterType = value
}
/**
* @param value Proposed (or dry run) ServicePerimeter configuration.
* This configuration allows to specify and test ServicePerimeter configuration
* without enforcing actual access restrictions. Only allowed to be set when
* the `useExplicitDryRunSpec` flag is set.
* Structure is documented below.
*/
@JvmName("spuwhlpxdftuhbeo")
public suspend fun spec(`value`: Output) {
this.spec = value
}
/**
* @param value ServicePerimeter configuration. Specifies sets of resources,
* restricted services and access levels that determine
* perimeter content and boundaries.
* Structure is documented below.
*/
@JvmName("vyfrycggqekxlbwb")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value Human readable title. Must be unique within the Policy.
*/
@JvmName("fjcmtigeqkgemxgr")
public suspend fun title(`value`: Output) {
this.title = value
}
/**
* @param value (Output)
* Time the AccessPolicy was updated in UTC.
*/
@JvmName("iyndtikxsludushx")
public suspend fun updateTime(`value`: Output) {
this.updateTime = value
}
/**
* @param value Use explicit dry run spec flag. Ordinarily, a dry-run spec implicitly exists
* for all Service Perimeters, and that spec is identical to the status for those
* Service Perimeters. When this flag is set, it inhibits the generation of the
* implicit spec, thereby allowing the user to explicitly provide a
* configuration ("spec") to use in a dry-run version of the Service Perimeter.
* This allows the user to test changes to the enforced config ("status") without
* actually enforcing them. This testing is done through analyzing the differences
* between currently enforced and suggested restrictions. useExplicitDryRunSpec must
* bet set to True if any of the fields in the spec are set to non-default values.
*/
@JvmName("qodacggnrdanbkkk")
public suspend fun useExplicitDryRunSpec(`value`: Output) {
this.useExplicitDryRunSpec = value
}
/**
* @param value (Output)
* Time the AccessPolicy was created in UTC.
*/
@JvmName("hcufudaonqdumagt")
public suspend fun createTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.createTime = mapped
}
/**
* @param value Description of the ServicePerimeter and its use. Does not affect
* behavior.
*/
@JvmName("tgshsbshugkftrle")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Resource name for the ServicePerimeter. The short_name component must
* begin with a letter and only include alphanumeric and '_'.
* Format: accessPolicies/{policy_id}/servicePerimeters/{short_name}
*/
@JvmName("lqpwunxrmkcqihvg")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Specifies the type of the Perimeter. There are two types: regular and
* bridge. Regular Service Perimeter contains resources, access levels,
* and restricted services. Every resource can be in at most
* ONE regular Service Perimeter.
* In addition to being in a regular service perimeter, a resource can also
* be in zero or more perimeter bridges. A perimeter bridge only contains
* resources. Cross project operations are permitted if all effected
* resources share some perimeter (whether bridge or regular). Perimeter
* Bridge does not contain access levels or services: those are governed
* entirely by the regular perimeter that resource is in.
* Perimeter Bridges are typically useful when building more complex
* topologies with many independent perimeters that need to share some data
* with a common perimeter, but should not be able to share data among
* themselves.
* Default value is `PERIMETER_TYPE_REGULAR`.
* Possible values are: `PERIMETER_TYPE_REGULAR`, `PERIMETER_TYPE_BRIDGE`.
*/
@JvmName("tietmuviqgwyxdkl")
public suspend fun perimeterType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.perimeterType = mapped
}
/**
* @param value Proposed (or dry run) ServicePerimeter configuration.
* This configuration allows to specify and test ServicePerimeter configuration
* without enforcing actual access restrictions. Only allowed to be set when
* the `useExplicitDryRunSpec` flag is set.
* Structure is documented below.
*/
@JvmName("oexgubbhgpyqorvg")
public suspend fun spec(`value`: ServicePerimetersServicePerimeterSpecArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spec = mapped
}
/**
* @param argument Proposed (or dry run) ServicePerimeter configuration.
* This configuration allows to specify and test ServicePerimeter configuration
* without enforcing actual access restrictions. Only allowed to be set when
* the `useExplicitDryRunSpec` flag is set.
* Structure is documented below.
*/
@JvmName("pajrygxnjmrojnau")
public suspend fun spec(argument: suspend ServicePerimetersServicePerimeterSpecArgsBuilder.() -> Unit) {
val toBeMapped = ServicePerimetersServicePerimeterSpecArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.spec = mapped
}
/**
* @param value ServicePerimeter configuration. Specifies sets of resources,
* restricted services and access levels that determine
* perimeter content and boundaries.
* Structure is documented below.
*/
@JvmName("odahsqgwubhnjmnm")
public suspend fun status(`value`: ServicePerimetersServicePerimeterStatusArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param argument ServicePerimeter configuration. Specifies sets of resources,
* restricted services and access levels that determine
* perimeter content and boundaries.
* Structure is documented below.
*/
@JvmName("cuhhjwppdheksfoo")
public suspend fun status(argument: suspend ServicePerimetersServicePerimeterStatusArgsBuilder.() -> Unit) {
val toBeMapped = ServicePerimetersServicePerimeterStatusArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.status = mapped
}
/**
* @param value Human readable title. Must be unique within the Policy.
*/
@JvmName("otdlfboqfcwgcvyk")
public suspend fun title(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.title = mapped
}
/**
* @param value (Output)
* Time the AccessPolicy was updated in UTC.
*/
@JvmName("xbdnkhhvcnhixoip")
public suspend fun updateTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.updateTime = mapped
}
/**
* @param value Use explicit dry run spec flag. Ordinarily, a dry-run spec implicitly exists
* for all Service Perimeters, and that spec is identical to the status for those
* Service Perimeters. When this flag is set, it inhibits the generation of the
* implicit spec, thereby allowing the user to explicitly provide a
* configuration ("spec") to use in a dry-run version of the Service Perimeter.
* This allows the user to test changes to the enforced config ("status") without
* actually enforcing them. This testing is done through analyzing the differences
* between currently enforced and suggested restrictions. useExplicitDryRunSpec must
* bet set to True if any of the fields in the spec are set to non-default values.
*/
@JvmName("lbqkviwfcxjlrwyc")
public suspend fun useExplicitDryRunSpec(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useExplicitDryRunSpec = mapped
}
internal fun build(): ServicePerimetersServicePerimeterArgs =
ServicePerimetersServicePerimeterArgs(
createTime = createTime,
description = description,
name = name ?: throw PulumiNullFieldException("name"),
perimeterType = perimeterType,
spec = spec,
status = status,
title = title ?: throw PulumiNullFieldException("title"),
updateTime = updateTime,
useExplicitDryRunSpec = useExplicitDryRunSpec,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy