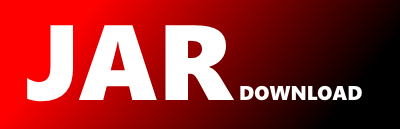
com.pulumi.gcp.activedirectory.kotlin.DomainTrustArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.activedirectory.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.activedirectory.DomainTrustArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Adds a trust between Active Directory domains
* To get more information about DomainTrust, see:
* * [API documentation](https://cloud.google.com/managed-microsoft-ad/reference/rest/v1/projects.locations.global.domains/attachTrust)
* * How-to Guides
* * [Active Directory Trust](https://cloud.google.com/managed-microsoft-ad/docs/create-one-way-trust)
* ## Example Usage
* ### Active Directory Domain Trust Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const ad_domain_trust = new gcp.activedirectory.DomainTrust("ad-domain-trust", {
* domain: "test-managed-ad.com",
* targetDomainName: "example-gcp.com",
* targetDnsIpAddresses: ["10.1.0.100"],
* trustDirection: "OUTBOUND",
* trustType: "FOREST",
* trustHandshakeSecret: "Testing1!",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* ad_domain_trust = gcp.activedirectory.DomainTrust("ad-domain-trust",
* domain="test-managed-ad.com",
* target_domain_name="example-gcp.com",
* target_dns_ip_addresses=["10.1.0.100"],
* trust_direction="OUTBOUND",
* trust_type="FOREST",
* trust_handshake_secret="Testing1!")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var ad_domain_trust = new Gcp.ActiveDirectory.DomainTrust("ad-domain-trust", new()
* {
* Domain = "test-managed-ad.com",
* TargetDomainName = "example-gcp.com",
* TargetDnsIpAddresses = new[]
* {
* "10.1.0.100",
* },
* TrustDirection = "OUTBOUND",
* TrustType = "FOREST",
* TrustHandshakeSecret = "Testing1!",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/activedirectory"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := activedirectory.NewDomainTrust(ctx, "ad-domain-trust", &activedirectory.DomainTrustArgs{
* Domain: pulumi.String("test-managed-ad.com"),
* TargetDomainName: pulumi.String("example-gcp.com"),
* TargetDnsIpAddresses: pulumi.StringArray{
* pulumi.String("10.1.0.100"),
* },
* TrustDirection: pulumi.String("OUTBOUND"),
* TrustType: pulumi.String("FOREST"),
* TrustHandshakeSecret: pulumi.String("Testing1!"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.activedirectory.DomainTrust;
* import com.pulumi.gcp.activedirectory.DomainTrustArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var ad_domain_trust = new DomainTrust("ad-domain-trust", DomainTrustArgs.builder()
* .domain("test-managed-ad.com")
* .targetDomainName("example-gcp.com")
* .targetDnsIpAddresses("10.1.0.100")
* .trustDirection("OUTBOUND")
* .trustType("FOREST")
* .trustHandshakeSecret("Testing1!")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* ad-domain-trust:
* type: gcp:activedirectory:DomainTrust
* properties:
* domain: test-managed-ad.com
* targetDomainName: example-gcp.com
* targetDnsIpAddresses:
* - 10.1.0.100
* trustDirection: OUTBOUND
* trustType: FOREST
* trustHandshakeSecret: Testing1!
* ```
*
* ## Import
* DomainTrust can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/global/domains/{{domain}}/{{target_domain_name}}`
* * `{{project}}/{{domain}}/{{target_domain_name}}`
* * `{{domain}}/{{target_domain_name}}`
* When using the `pulumi import` command, DomainTrust can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:activedirectory/domainTrust:DomainTrust default projects/{{project}}/locations/global/domains/{{domain}}/{{target_domain_name}}
* ```
* ```sh
* $ pulumi import gcp:activedirectory/domainTrust:DomainTrust default {{project}}/{{domain}}/{{target_domain_name}}
* ```
* ```sh
* $ pulumi import gcp:activedirectory/domainTrust:DomainTrust default {{domain}}/{{target_domain_name}}
* ```
* @property domain The fully qualified domain name. e.g. mydomain.myorganization.com, with the restrictions,
* https://cloud.google.com/managed-microsoft-ad/reference/rest/v1/projects.locations.global.domains.
* - - -
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property selectiveAuthentication Whether the trusted side has forest/domain wide access or selective access to an approved set of resources.
* @property targetDnsIpAddresses The target DNS server IP addresses which can resolve the remote domain involved in the trust.
* @property targetDomainName The fully qualified target domain name which will be in trust with the current domain.
* @property trustDirection The trust direction, which decides if the current domain is trusted, trusting, or both.
* Possible values are: `INBOUND`, `OUTBOUND`, `BIDIRECTIONAL`.
* @property trustHandshakeSecret The trust secret used for the handshake with the target domain. This will not be stored.
* **Note**: This property is sensitive and will not be displayed in the plan.
* @property trustType The type of trust represented by the trust resource.
* Possible values are: `FOREST`, `EXTERNAL`.
*/
public data class DomainTrustArgs(
public val domain: Output? = null,
public val project: Output? = null,
public val selectiveAuthentication: Output? = null,
public val targetDnsIpAddresses: Output>? = null,
public val targetDomainName: Output? = null,
public val trustDirection: Output? = null,
public val trustHandshakeSecret: Output? = null,
public val trustType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.activedirectory.DomainTrustArgs =
com.pulumi.gcp.activedirectory.DomainTrustArgs.builder()
.domain(domain?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.selectiveAuthentication(selectiveAuthentication?.applyValue({ args0 -> args0 }))
.targetDnsIpAddresses(targetDnsIpAddresses?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.targetDomainName(targetDomainName?.applyValue({ args0 -> args0 }))
.trustDirection(trustDirection?.applyValue({ args0 -> args0 }))
.trustHandshakeSecret(trustHandshakeSecret?.applyValue({ args0 -> args0 }))
.trustType(trustType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DomainTrustArgs].
*/
@PulumiTagMarker
public class DomainTrustArgsBuilder internal constructor() {
private var domain: Output? = null
private var project: Output? = null
private var selectiveAuthentication: Output? = null
private var targetDnsIpAddresses: Output>? = null
private var targetDomainName: Output? = null
private var trustDirection: Output? = null
private var trustHandshakeSecret: Output? = null
private var trustType: Output? = null
/**
* @param value The fully qualified domain name. e.g. mydomain.myorganization.com, with the restrictions,
* https://cloud.google.com/managed-microsoft-ad/reference/rest/v1/projects.locations.global.domains.
* - - -
*/
@JvmName("onggytlaaxavahuo")
public suspend fun domain(`value`: Output) {
this.domain = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("hyggodnrmntpwmic")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Whether the trusted side has forest/domain wide access or selective access to an approved set of resources.
*/
@JvmName("qpvpwjicgikrbfmt")
public suspend fun selectiveAuthentication(`value`: Output) {
this.selectiveAuthentication = value
}
/**
* @param value The target DNS server IP addresses which can resolve the remote domain involved in the trust.
*/
@JvmName("irdglqthqtlnfxts")
public suspend fun targetDnsIpAddresses(`value`: Output>) {
this.targetDnsIpAddresses = value
}
@JvmName("cdboymkvdlthktft")
public suspend fun targetDnsIpAddresses(vararg values: Output) {
this.targetDnsIpAddresses = Output.all(values.asList())
}
/**
* @param values The target DNS server IP addresses which can resolve the remote domain involved in the trust.
*/
@JvmName("mcttbttukouveljf")
public suspend fun targetDnsIpAddresses(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy